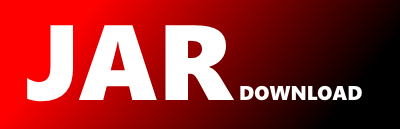
de.vandermeer.asciitable.v1.V1_AsciiTable Maven / Gradle / Ivy
/* Copyright 2014 Sven van der Meer
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.vandermeer.asciitable.v1;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.ArrayUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.text.StrBuilder;
import org.apache.commons.lang3.text.WordUtils;
import de.vandermeer.asciitable.commons.ArrayTransformations;
import de.vandermeer.asciitable.commons.ObjectToStringStyle;
/**
* Original ASCII table with flexible column number, column width, wrapping, spanning and themes.
*
* @author Sven van der Meer <[email protected]>
* @version v1.0.0 build 160319 (19-Mar-16) for Java 1.7
* @since v0.0.1
*/
public final class V1_AsciiTable {
/** Padding character, default is blank (␣). */
char padChar = ' ';
/** Rendering theme, default is plain 7 bit */
V1_StandardTableThemes theme = V1_StandardTableThemes.PLAIN_7BIT;
/** Column array. Entry 0 holds the width of the table, each following entry i the width of column i. */
private Integer[] columns;
/** The actual table. Each row is identified by an integer, count starts at 1. Columns are then linked lists of strings */
protected Map table;
/**
* Returns a new instance of an ASCII Table.
* The available width will be evenly distributed over the number of columns. If space is left, the columns
* starting from 1 upwards will receive an extra character until the left space is consumed.
* The minimum width of a column is 3. This means that width must be at least (columns * 3 + columns + 1).
* @param columns number of columns for the table
* @param width maximum width of the table
* @return new instance or null in case the required width was insufficient for the number of columns
*/
public static V1_AsciiTable newTable(Integer columns, Integer width){
V1_AsciiTable ret = new V1_AsciiTable();
if(ret._init(columns, width)==false){
return null;
}
return ret;
}
/**
* Returns a new instance of an ASCII Table.
* The actual width of the table is the sum of the width of all columns plus the characters needed to separate columns.
* The latter is calculated as (number of columns + 1). Each entry in the column array will be processed and <null> will
* be returned if the entry is <null> or if the requested width for a column is less than 3.
* @param columns array with information about the width of each column (entries of array) and number of columns (size of array)
* @return new instance or null if no columns given or a column was null or a column width was set to smaller than 3
*/
public static V1_AsciiTable newTable(Integer[] columns){
V1_AsciiTable ret = new V1_AsciiTable();
if(ret._init(columns)==false){
return null;
}
return ret;
}
/**
* Instantiates a table.
* Use {@link #newTable(Integer[])} or {@link #newTable(Integer, Integer)} to obtain an instance of the ASCII table.
*/
protected V1_AsciiTable(){
this.table=new HashMap(10);
// this.rowFormat=new HashMap(10);
}
/**
* Sets the padding character, default is blank.
* @param padChar new padding character
* @return self for chaining
*/
public V1_AsciiTable setPaddingCharacter(char padChar){
this.padChar = padChar;
return this;
}
/**
* Sets the rendering theme, default is plain 7 bit.
* @param theme new theme
* @return self for chaining
*/
public V1_AsciiTable setTheme(V1_StandardTableThemes theme){
if(theme!=null){
this.theme = theme;
}
return this;
}
/**
* Adds a new row to the table.
* For each column of the set column size an object must be provided. This object can be <null>.
* It is also allowed that the object's toString() method returns null. The table row will later be build using this information
* in the following way:
*
* - if the object for a column is null (or it's toString() method returns null), the column is assumed to be part of a column span.
* This span is calculated from the first column set to null up to the next column that is set to either empty ("") or some content
* (non-empty string). Column spans at the end of a row result in an empty row spanning all requested columns.
* For example, for a 3-column row with equal column-width of 4 characters (plus vertical separators)
*
* - (null, null, "text") will result in a row {@code "|␣␣␣␣␣text␣␣␣␣␣|"}
* - (null, "text", null) will result in a row {@code "|␣␣text␣␣␣|␣␣␣␣|"}
* - ("text", null, null) will result in a row {@code "|text|␣␣␣␣␣␣␣␣␣|"}
* - (null, null, null) will result in a row {@code "|␣␣␣␣␣␣␣␣␣␣␣␣␣␣|"}
*
* - if the object's toString() method results in an empty string (""), the column will be padded with the padding character
* - in any other cases the result of the object's toString() method is used as text for the column
*
* @param columns text for the columns
* @return -1 if table has no columns, 0 if given columns are 0 or if number of given columns is not the number of the set columns, index of the added row in all other cases
*/
public int addRow(Object ...columns){
if(this.columns==null){
return -1;
}
if(columns==null || columns.length!=this.getColumnCount()){
return 0;
}
String[][] ar = new String[this.getColumnCount()][];
for(int i=0; i tab = this.renderTable(this.padChar, this.theme.getTheme());
for(StrBuilder b : tab){
ret.appendln(b);
}
return ret.toString();
}
/**
* Renders a middle rule, that is a row separating two content rows.
* @param top content row above the middle rule
* @param bot content row below the middle rule
* @param theme row theme
* @return line for the middle rule
*/
protected final StrBuilder renderMiddleRule(String[][] top, String[][] bot, char[] theme){
StrBuilder ret = new StrBuilder(100);
ret.append(theme[V1_TableTheme.VERTICAL_AND_RIGHT]);
String[] topSt = top[top.length-1];
String[] botSt = bot[0];
for(int i=0; i renderTable(char padChar, char[] theme){
List ret = new ArrayList();
if(this.table.size()>0){
ret.add(this.renderTopRule(this.table.get(1), theme));
if(this.table.size()>1){ //if more than one row do all but the last row
for(int i=1; i
// * l = align left (default)
// * r = align right
// * c = centre
// * null = use the default or ignore if column is set to span other columns
// *
// * The argument {@code characters} must have the same length as columns set.
// * @param row index for the row
// * @param characters array of formatting instructions
// * @return index of row with format, -1 if no columns set or row index does not exist, 0 if problem with format characters (no formats given, wrong count)
// */
// public int setRowFormats(int row, Character ...characters){
// if(this.columns==null){
// return -1;
// }
// if(!this.table.containsKey(row)){
// return -1;
// }
// if(characters==null||characters.length!=this.getColumnCount()){
// return 0;
// }
//
// this.rowFormat.put(row, characters);
// return row;
// }
/**
* Returns a string with debug information.
* @return string with debug information about the table
*/
public String toString(){
ToStringBuilder ret = new ToStringBuilder(this, ObjectToStringStyle.getStyle())
.append("columns ", this.columns, false)
.append("columns ", this.columns)
.append("------------------------------------")
.append("table ", this.table, false)
;
if(this.table!=null && this.table.size()>0){
for(Integer i : this.table.keySet()){
ret.append(String.format(" row(%3d)", i), this.table.get(i));
}
}
ret.append("------------------------------------");
return ret.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy