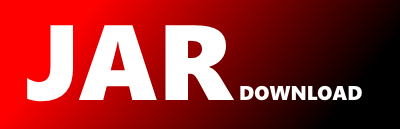
de.vandermeer.asciitable.v2.render.V2_AsciiTableRenderer Maven / Gradle / Ivy
/* Copyright 2014 Sven van der Meer
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.vandermeer.asciitable.v2.render;
import java.util.LinkedList;
import java.util.List;
import org.apache.commons.lang3.ArrayUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.text.StrBuilder;
import de.vandermeer.asciitable.v2.RenderedTable;
import de.vandermeer.asciitable.v2.V2_AsciiTable;
import de.vandermeer.asciitable.v2.row.ContentRow;
import de.vandermeer.asciitable.v2.row.RuleRow;
import de.vandermeer.asciitable.v2.row.RuleRowType;
import de.vandermeer.asciitable.v2.row.V2_Row;
import de.vandermeer.asciitable.v2.themes.ThemeValidator;
import de.vandermeer.asciitable.v2.themes.V2_E_TableThemes;
import de.vandermeer.asciitable.v2.themes.V2_RowTheme;
import de.vandermeer.asciitable.v2.themes.V2_TableTheme;
/**
* Renders a table.
*
* Using the renderer is very simple. First, create and fill a table:
* {@code
V2_AsciiTable at = new V2_AsciiTable();
at.addRule();
at.addRow("1-1", "1-2", "1-3");
at.addRule();
at.addRow("2-1", "2-2", "2-3");
at.addRule();
* }
*
* Then create a renderer and set the table width,
* set the theme to be used (if other than the default theme {@link V2_E_TableThemes#PLAIN_7BIT}) and
* set the padding character to be used (if other than the default padding character ' '):
* {@code
V2_AsciiTableRenderer rend = new V2_AsciiTableRenderer();
rend.setTheme(V2_E_TableThemes.UTF_LIGHT.get());
rend.setWidth(new V2_WidthAbsoluteEven().setWidth(25));
rend.setPaddingChar('_');
* }
*
* Finally render the created table, resulting in a rendered table ready to be printed or written to a file:
* {@code
RenderedTable rt = rend.render(at);
System.out.println(rt);
* }
*
* The example above will result in this table printed to standard out:
*
┌───────┬───────┬───────┐
│_1-1___│_1-2___│_1-3___│
├───────┼───────┼───────┤
│_2-1___│_2-2___│_2-3___│
└───────┴───────┴───────┘
*
*
*
* @author Sven van der Meer <[email protected]>
* @version v1.0.0 build 160319 (19-Mar-16) for Java 1.7
* @since v0.0.5
*/
public class V2_AsciiTableRenderer implements V2_TableRenderer {
/** Character used for padding in table columns. */
char paddingChar;
/** The theme for the table. */
V2_TableTheme theme;
/** Width of the table. */
V2_Width width;
/** List of rows processed and ready to b rendered. */
List rows;
/**
* Returns a new table row renderer.
* Default values are:
*
* - Padding character: blank (' ')
* - Theme: plain 7 bit ASCII theme
*
*/
public V2_AsciiTableRenderer(){
this.paddingChar = ' ';
this.theme = V2_E_TableThemes.PLAIN_7BIT.get();
this.width = null;
this.rows = new LinkedList<>();
}
@Override
public V2_AsciiTableRenderer setWidth(V2_Width width){
if(width!=null){
this.width = width;
}
return this;
}
@Override
public RenderedTable render(V2_AsciiTable table){
this.rows.clear();
//nothing to do
if(table==null || table.getColumnCount()==0){
throw new IllegalArgumentException("wrong table argument: table is null or has no columns");
}
//no width set for table, nothing we can do
if(this.width==null){
throw new IllegalArgumentException("wrong table width argument: no width set");
}
int[] cols = this.width.getColumnWidths(table);
//got width, now prepare all table information
//now create a list of processed table rows
for(V2_Row row : table.getTable()){
ProcessedRow pr = new ProcessedRow(row, cols, table.getColumnCount());
if(row instanceof ContentRow){
ContentRow crow = (ContentRow)row;
String[][] procColumns = RenderUtilities.createContentArray(crow.getColumns(), cols, crow.getPadding());
pr.setProcessedColumns(procColumns);
pr.setBorderTypes(RenderUtilities.getBorderTypes_ContentRow(procColumns[0], crow, table.getColumnCount()));
}
this.rows.add(pr);
}
//now adjust borders for top rule
BorderType[] array = RenderUtilities.getBorderTypes_TopRule((this.rows.size()>1)?this.rows.get(1):null, this.rows.get(0).getOriginalRow(), table.getColumnCount());
this.rows.get(0).setBorderTypes(array);
//adjust border for bottom rule
array = RenderUtilities.getBorderTypes_BottomRule((this.rows.size()>1)?this.rows.get(this.rows.size()-2):null, this.rows.get(this.rows.size()-1).getOriginalRow(), table.getColumnCount());
this.rows.get(this.rows.size()-1).setBorderTypes(array);
//and now adjust borders for all mid rules
if(this.rows.size()>2){
for(int r=1; r ret = new LinkedList();
for(int i=0; i0){
width = width - padding*2;
}
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy