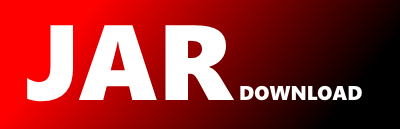
de.viadee.bpm.vPAV.OuterProcessVariablesScanner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of viadeeProcessApplicationValidator Show documentation
Show all versions of viadeeProcessApplicationValidator Show documentation
The tool checks Camunda projects for consistency and discovers errors in process-driven applications.
Called as a Maven plugin or JUnit test, it discovers esp. inconsistencies of a given BPMN model in the classpath and the
sourcecode of an underlying java project, such as a delegate reference to a non-existing java class or a non-existing Spring bean.
/**
* Copyright © 2017, viadee Unternehmensberatung GmbH
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. All advertising materials mentioning features or use of this software
* must display the following acknowledgement:
* This product includes software developed by the viadee Unternehmensberatung GmbH.
* 4. Neither the name of the viadee Unternehmensberatung GmbH nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY ''AS IS'' AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package de.viadee.bpm.vPAV;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.collections4.ListUtils;
import org.apache.commons.io.IOUtils;
import org.apache.tools.ant.DirectoryScanner;
import org.apache.tools.ant.types.Resource;
import groovyjarjarasm.asm.ClassReader;
import groovyjarjarasm.asm.ClassVisitor;
import groovyjarjarasm.asm.FieldVisitor;
import groovyjarjarasm.asm.Opcodes;
/**
* scan process variables, which are set in outer java classes
*
* important TODO: variables to bpmn element (message correlation)
*/
public class OuterProcessVariablesScanner {
private Set javaResources;
private Map> messageIdToVariableMap = new HashMap>();
private Map> processIdToVariableMap = new HashMap>();
public OuterProcessVariablesScanner(final Set javaResources) {
this.javaResources = javaResources;
}
/**
* scan variables
*
* @throws IOException
* possible exception if filepath can not be resolved
*/
public void scanProcessVariables() throws IOException {
for (final String filePath : javaResources) {
if (!filePath.startsWith("javax")) {
final String content = readResourceFile(filePath);
if (content != null) {
final Collection initialProcessVariablesInFilePath = readVariablesOfInnerClassInitialProcessVariables(
filePath);
if (!initialProcessVariablesInFilePath.isEmpty()) {
// if correlateMessage and startProcessInstanceByMessage called
// together in one class take the intersection to avoid duplicates
final Set messageIds = new HashSet();
messageIds.addAll(checkStartProcessByMessageIdPattern(content));
messageIds.addAll(checkCorrelateMessagePattern(content));
for (final String messageId : messageIds) {
if (messageIdToVariableMap.containsKey(messageId)) {
// if messageId is already set, create intersection of variables and overwrite map
// item
final Collection existingProcessVariables = messageIdToVariableMap
.get(messageId);
final List intersectionProcessVariables = ListUtils.intersection(
(List) existingProcessVariables,
(List) initialProcessVariablesInFilePath);
messageIdToVariableMap.put(messageId, intersectionProcessVariables);
} else {
messageIdToVariableMap.put(messageId, initialProcessVariablesInFilePath);
}
}
final Collection processIds = checkStartProcessByKeyPattern(content);
for (final String processId : processIds) {
processIdToVariableMap.put(processId, initialProcessVariablesInFilePath);
}
}
}
}
}
}
/**
* get mapping for message id
*
* @return messageIdToVariableMap returns messageIdToVariableMap
*/
public Map> getMessageIdToVariableMap() {
return messageIdToVariableMap;
}
/**
* get mapping for process id
*
* @return processIdToVariableMap returns processIdToVariableMap
*/
public Map> getProcessIdToVariableMap() {
return processIdToVariableMap;
}
/**
* read resource file
*
* @param fileName
* Name of file
* @return methodBody returns methodBody
*/
private String readResourceFile(final String filePath) {
String methodBody = "";
if (filePath != null && filePath.trim().length() > 0) {
try {
final DirectoryScanner scanner = new DirectoryScanner();
if (RuntimeConfig.getInstance().isTest()) {
scanner.setBasedir(ConstantsConfig.TEST_JAVAPATH);
} else {
scanner.setBasedir(ConstantsConfig.JAVAPATH);
}
Resource s = scanner.getResource(filePath);
if (s.isExists()) {
InputStreamReader resource = new InputStreamReader(new FileInputStream(s.toString()));
methodBody = IOUtils.toString(resource);
} else {
}
} catch (final IOException ex) {
throw new RuntimeException(
"resource '" + filePath + "' could not be read: " + ex.getMessage());
}
}
return methodBody;
}
/**
* check pattern for startProcessInstanceByMessage
*
* @param code
* @return message ids
*/
private Collection checkStartProcessByMessageIdPattern(final String code) {
// remove special characters from code
final String FILTER_PATTERN = "'|\"| ";
final String cleanedCode = code.replaceAll(FILTER_PATTERN, "");
// search locations where variables are read
final Pattern pattern = Pattern.compile("\\.startProcessInstanceByMessage\\((\\w+),(.*)");
final Matcher matcher = pattern.matcher(cleanedCode);
final Collection messageIds = new ArrayList();
while (matcher.find()) {
final String match = matcher.group(1);
messageIds.add(match);
}
return messageIds;
}
/**
* check pattern for startProcessInstanceByKey
*
* @param code
* @return process keys
*/
private Collection checkStartProcessByKeyPattern(final String code) {
// remove special characters from code
final String FILTER_PATTERN = "'|\"| ";
final String cleanedCode = code.replaceAll(FILTER_PATTERN, "");
// search locations where variables are read
final Pattern pattern = Pattern.compile("\\.startProcessInstanceByKey\\((\\w+),(.*)");
final Matcher matcher = pattern.matcher(cleanedCode);
final Collection processIds = new ArrayList();
while (matcher.find()) {
final String match = matcher.group(1);
processIds.add(match);
}
return processIds;
}
/**
* check pattern for correlateMessage
*
* @param code
* @return message ids
*/
private Collection checkCorrelateMessagePattern(final String code) {
// remove special characters from code
final String FILTER_PATTERN = "'|\"| ";
final String cleanedCode = code.replaceAll(FILTER_PATTERN, "");
// search locations where variables are read
final Pattern pattern = Pattern.compile("\\.correlateMessage\\((\\w+),(.*)");
final Matcher matcher = pattern.matcher(cleanedCode);
final Collection messageIds = new ArrayList();
while (matcher.find()) {
final String match = matcher.group(1);
messageIds.add(match);
}
return messageIds;
}
/**
* For given filePath returns fields of inner class InitialProcessVariables. This class is used to initialize the
* process
*
* @param filePath
* @return
* @throws IOException
*/
private Collection readVariablesOfInnerClassInitialProcessVariables(final String filePath)
throws IOException {
final Collection processVariables = new ArrayList();
if (filePath != null) {
final String[] splittedFilePath = filePath.split("\\.");
if (splittedFilePath.length > 0) {
ClassVisitor cl = new ClassVisitor(Opcodes.ASM4) {
@Override
public FieldVisitor visitField(int access, String name, String desc, String signature,
Object value) {
if (!name.startsWith("this")) {
processVariables.add(name);
}
super.visitField(access, name, desc, signature, value);
return null;
}
};
InputStream in = RuntimeConfig.getInstance().getClassLoader()
.getResourceAsStream(splittedFilePath[0] + "$InitialProcessVariables.class");
if (in != null) {
ClassReader classReader = new ClassReader(in);
classReader.accept(cl, 0);
}
}
}
return processVariables;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy