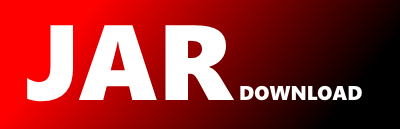
de.viadee.bpm.vPAV.processing.model.data.BpmnElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of viadeeProcessApplicationValidator Show documentation
Show all versions of viadeeProcessApplicationValidator Show documentation
The tool checks Camunda projects for consistency and discovers errors in process-driven applications.
Called as a Maven plugin or JUnit test, it discovers esp. inconsistencies of a given BPMN model in the classpath and the
sourcecode of an underlying java project, such as a delegate reference to a non-existing java class or a non-existing Spring bean.
/**
* Copyright © 2017, viadee Unternehmensberatung GmbH
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. All advertising materials mentioning features or use of this software
* must display the following acknowledgement:
* This product includes software developed by the viadee Unternehmensberatung GmbH.
* 4. Neither the name of the viadee Unternehmensberatung GmbH nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY ''AS IS'' AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package de.viadee.bpm.vPAV.processing.model.data;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.camunda.bpm.model.bpmn.instance.BaseElement;
/**
* Represents an BPMN element
*
*/
public class BpmnElement {
private String processdefinition;
private BaseElement baseElement;
private Map used = new HashMap();
private Map defined = new HashMap();
private Map in = new HashMap();
private Map out = new HashMap();
/* in interface for call activity */
private Collection inCa;
/* out interface for call activity */
private Collection outCa;
private Map processVariables;
public BpmnElement(final String processdefinition, final BaseElement element) {
this.processdefinition = processdefinition;
this.baseElement = element;
this.processVariables = new HashMap();
}
public String getProcessdefinition() {
return processdefinition;
}
public BaseElement getBaseElement() {
return baseElement;
}
public Map getProcessVariables() {
return processVariables;
}
public void setProcessVariables(final Map variables) {
this.processVariables = variables;
}
public void setProcessVariable(final String variableName, final ProcessVariable variableObject) {
processVariables.put(variableName, variableObject);
}
@Override
public int hashCode() {
return baseElement.getId().hashCode();
}
@Override
public boolean equals(Object o) {
if (o instanceof BpmnElement && this.hashCode() == o.hashCode()) {
return true;
}
return false;
}
@Override
public String toString() {
return baseElement.getId();
}
public Map getIn() {
return in;
}
public Map getOut() {
return out;
}
public void setIn(final Map outPredecessor) {
this.in = outPredecessor;
// TODO: call activity (create own method)
if (inCa != null) {
final Collection removeCandidates = new ArrayList();
for (final String variable : in.keySet()) {
if (!inCa.contains(variable)) {
removeCandidates.add(variable);
}
}
for (final String var : removeCandidates) {
in.remove(var);
}
}
}
public void setOut() {
out.putAll(defined());
changeStatusToRead(in);
out.putAll(killed());
// TODO: call activity (create own method)
if (outCa != null) {
final Collection removeCandidates = new ArrayList();
for (final String variable : out.keySet()) {
if (!outCa.contains(variable)) {
removeCandidates.add(variable);
} else {
final InOutState state = out.get(variable);
if (state == InOutState.DELETED) {
removeCandidates.add(variable);
}
}
}
for (final String var : removeCandidates) {
out.remove(var);
}
}
}
private Map used() {
if (this.used.isEmpty()) {
for (final ProcessVariable var : processVariables.values()) {
if (var.getOperation() == VariableOperation.READ) {
used.put(var.getName(), InOutState.READ);
}
}
}
return used;
}
public Map defined() {
if (this.defined.isEmpty()) {
for (final ProcessVariable var : processVariables.values()) {
if (var.getOperation() == VariableOperation.WRITE) {
defined.put(var.getName(), InOutState.DEFINED);
}
}
}
return defined;
}
private Map killed() {
final Map killedVariables = new HashMap();
for (final ProcessVariable var : processVariables.values()) {
if (var.getOperation() == VariableOperation.DELETE) {
killedVariables.put(var.getName(), InOutState.DELETED);
}
}
return killedVariables;
}
public void setInCa(final Collection in) {
this.inCa = in;
}
public void setOutCa(final Collection out) {
this.outCa = out;
}
public boolean ur(final String varName) {
if ((in.containsKey(varName) == false
|| (in.containsKey(varName) == true && in.get(varName) == InOutState.DELETED))
&& used().containsKey(varName)) {
return true;
}
return false;
}
public boolean du(final String varName) {
if (in.containsKey(varName) && in.get(varName) == InOutState.DEFINED && out.containsKey(varName)
&& out.get(varName) == InOutState.DELETED) {
return true;
}
return false;
}
public boolean dd(final String varName) {
if (in.containsKey(varName) && in.get(varName) == InOutState.DEFINED
&& defined().containsKey(varName)) {
return true;
}
return false;
}
public Map> getAnomalies() {
final Map> anomalyMap = new HashMap>();
final Set variableNames = new HashSet();
variableNames.addAll(used().keySet());
for (final String variableName : in.keySet()) {
if (in.get(variableName) == InOutState.DEFINED) {
variableNames.add(variableName);
}
}
final List anomalies = new ArrayList();
for (final String variableName : variableNames) {
if (ur(variableName)) {
anomalies.add(new AnomalyContainer(variableName, Anomaly.UR, baseElement.getId(),
processVariables.get(variableName)));
}
if (du(variableName)) {
anomalies.add(new AnomalyContainer(variableName, Anomaly.DU, baseElement.getId(),
processVariables.get(variableName)));
}
if (dd(variableName)) {
anomalies.add(new AnomalyContainer(variableName, Anomaly.DD, baseElement.getId(),
processVariables.get(variableName)));
}
}
anomalyMap.put(this, anomalies);
return anomalyMap;
}
private void changeStatusToRead(final Map inVariables) {
for (final String varName : inVariables.keySet()) {
if (used().containsKey(varName)) {
out.put(varName, InOutState.READ);
} else {
out.put(varName, inVariables.get(varName));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy