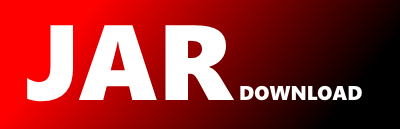
de.viadee.bpm.vPAV.config.reader.XmlConfigReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of viadeeProcessApplicationValidator Show documentation
Show all versions of viadeeProcessApplicationValidator Show documentation
The tool checks Camunda projects for consistency and discovers errors in process-driven applications.
Called as a Maven plugin or JUnit test, it discovers esp. inconsistencies of a given BPMN model in the classpath and the
sourcecode of an underlying java project, such as a delegate reference to a non-existing java class or a non-existing Spring bean.
/**
* BSD 3-Clause License
*
* Copyright © 2019, viadee Unternehmensberatung AG
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* * Neither the name of the copyright holder nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package de.viadee.bpm.vPAV.config.reader;
import de.viadee.bpm.vPAV.RuntimeConfig;
import de.viadee.bpm.vPAV.config.model.*;
import de.viadee.bpm.vPAV.constants.ConfigConstants;
import de.viadee.bpm.vPAV.processing.checker.DataFlowChecker;
import de.viadee.bpm.vPAV.processing.checker.ProcessVariablesModelChecker;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Logger;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
/**
* Used to read the config file (ruleSet.xml) and extract the configured rules Requirements:
* Existing ruleSet.xml in src/test/resources
*/
public final class XmlConfigReader implements ConfigReader {
private static final Logger LOGGER = Logger.getLogger(XmlConfigReader.class.getName());
/**
* @param file Location of file relative to project
* @throws ConfigReaderException If file can not be found in classpath
*/
@Override
public Map> read(final String file) throws ConfigReaderException {
try {
final JAXBContext jaxbContext = JAXBContext.newInstance(XmlRuleSet.class);
final Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
InputStream fRuleSet = RuntimeConfig.getInstance().getClassLoader().getResourceAsStream(file);
if (fRuleSet != null) {
final XmlRuleSet ruleSet = (XmlRuleSet) jaxbUnmarshaller.unmarshal(fRuleSet);
return transformFromXmlDatastructues(ruleSet);
} else {
throw new ConfigReaderException("ConfigFile could not be found");
}
} catch (JAXBException e) {
throw new ConfigReaderException(e);
}
}
/**
* Retrieves all rules, by default deactivated
*
* @return rules
*/
public Map> getDeactivatedRuleSet() {
final Map> rules = new HashMap<>();
Map newrule;
for (String name : RuntimeConfig.getInstance().getViadeeRules()) {
newrule = new HashMap<>();
if (name.equals("CreateOutputHTML")) {
newrule.put(
name,
new Rule(
name,
true,
null,
new HashMap(),
new ArrayList(),
new ArrayList()));
} else {
newrule.put(
name,
new Rule(
name,
false,
null,
new HashMap(),
new ArrayList(),
new ArrayList()));
}
rules.put(name, newrule);
}
return rules;
}
/**
* Transforms XmlRuleSet to rules
*
* @param ruleSet
* @return rules
* @throws ConfigReaderException If file could not be read properly
*/
private static Map> transformFromXmlDatastructues(
final XmlRuleSet ruleSet) throws ConfigReaderException {
final Map> rules = new HashMap<>();
final Collection xmlRules = ruleSet.getRules();
for (final XmlRule rule : xmlRules) {
final String id = (rule.getId() == null) ? rule.getName() : rule.getId();
final String name = rule.getName();
if (name == null) throw new ConfigReaderException("rule name is not set");
final boolean state = rule.isState();
final String ruleDescription = rule.getDescription();
final Collection xmlElementConventions = rule.getElementConventions();
final ArrayList elementConventions = new ArrayList();
if (xmlElementConventions != null) {
for (final XmlElementConvention xmlElementConvention : xmlElementConventions) {
final XmlElementFieldTypes xmlElementFieldTypes =
xmlElementConvention.getElementFieldTypes();
ElementFieldTypes elementFieldTypes = null;
if (xmlElementFieldTypes != null) {
elementFieldTypes =
new ElementFieldTypes(
xmlElementFieldTypes.getElementFieldTypes(), xmlElementFieldTypes.isExcluded());
}
if (!checkRegEx(xmlElementConvention.getPattern()))
throw new ConfigReaderException(
"RegEx ("
+ xmlElementConvention.getPattern()
+ ") of "
+ name
+ " ("
+ xmlElementConvention.getName()
+ ") is incorrect");
elementConventions.add(
new ElementConvention(
xmlElementConvention.getName(),
elementFieldTypes,
xmlElementConvention.getDescription(),
xmlElementConvention.getPattern()));
}
}
final Collection xmlModelConventions = rule.getModelConventions();
final ArrayList modelConventions = new ArrayList();
if (xmlModelConventions != null) {
for (final XmlModelConvention xmlModelConvention : xmlModelConventions) {
modelConventions.add(new ModelConvention(xmlModelConvention.getType()));
}
}
final Collection xmlSettings = rule.getSettings();
final Map settings = new HashMap();
if (xmlSettings != null) {
for (final XmlSetting xmlSetting : xmlSettings) {
if (!settings.containsKey(xmlSetting.getName())) {
settings.put(
xmlSetting.getName(),
new Setting(
xmlSetting.getName(),
xmlSetting.getScript(),
xmlSetting.getType(),
xmlSetting.getId(),
xmlSetting.getRequired(),
xmlSetting.getValue()));
} else {
settings.get(xmlSetting.getName()).addScriptPlace(xmlSetting.getScript());
}
}
}
if (!rules.containsKey(name)) {
rules.put(name, new HashMap());
}
rules.get(name).put(id, new Rule(id, name, state, ruleDescription, settings, elementConventions, modelConventions));
}
// TODO as soon as we finally move the properties to an external file, we don't need this checks anymore
// Some rules are only allowed once. Check this.
checkSingletonRule(rules, ConfigConstants.HASPARENTRULESET);
checkSingletonRule(rules, ConfigConstants.CREATE_OUTPUT_RULE);
checkSingletonRule(rules, "language");
if (ProcessVariablesModelChecker.isSingletonChecker()) {
checkSingletonRule(rules, ProcessVariablesModelChecker.class.getSimpleName());
}
if (DataFlowChecker.isSingletonChecker()) {
checkSingletonRule(rules, DataFlowChecker.class.getSimpleName());
}
return rules;
}
private static void checkSingletonRule(Map> rules, String rulename) {
Map rulesSubset = rules.get(rulename);
if (rulesSubset != null) {
if (rulesSubset.size() > 1 || rulesSubset.get(rulename) == null) {
LOGGER.severe("Rule '" + rulename + "' is only allowed once and without defining an ID.");
}
}
}
private static boolean checkRegEx(String regEx) {
boolean correct = false;
if (regEx.isEmpty()) return correct;
try {
Pattern.compile(regEx);
correct = true;
} catch (PatternSyntaxException e) {
}
return correct;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy