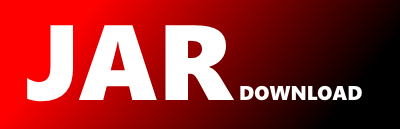
blended.updater.config.ConfigConverter.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blended.updater.config Show documentation
Show all versions of blended.updater.config Show documentation
Configurations for Updater and Launcher
package blended.updater.config
import java.io.File
import scala.collection.immutable._
import blended.launcher.config.LauncherConfig
import org.slf4j.LoggerFactory
/**
* Convert between [[LauncherConfig]] and [[ResolvedRuntimeConfig]].
*/
trait ConfigConverter {
import ConfigConverter._
private[this] val log = LoggerFactory.getLogger(classOf[ConfigConverter])
def runtimeConfigToLauncherConfig(resolvedRuntimeConfig: ResolvedRuntimeConfig, profileDir: String): LauncherConfig = {
import LauncherConfig._
val bundlePrefix = s"${profileDir}/bundles"
val runtimeConfig = resolvedRuntimeConfig.runtimeConfig
val allBundles = resolvedRuntimeConfig.allBundles.
filter(b => b.startLevel != Some(0)).
map { bc =>
BundleConfig(
location = s"${bundlePrefix}/${bc.jarName.getOrElse(runtimeConfig.resolveFileName(bc.url).get)}",
start = bc.start,
startLevel = bc.startLevel.getOrElse(runtimeConfig.defaultStartLevel))
}.
distinct
log.debug("Converted bundles: {}", allBundles)
LauncherConfig(
frameworkJar = s"${bundlePrefix}/${resolvedRuntimeConfig.framework.jarName.getOrElse(runtimeConfig.resolveFileName(resolvedRuntimeConfig.framework.url).get)}",
systemProperties = runtimeConfig.systemProperties,
frameworkProperties = runtimeConfig.frameworkProperties,
startLevel = runtimeConfig.startLevel,
defaultStartLevel = runtimeConfig.defaultStartLevel,
bundles = allBundles,
branding = runtimeConfig.properties ++ Map(
RuntimeConfig.Properties.PROFILE_NAME -> runtimeConfig.name,
RuntimeConfig.Properties.PROFILE_VERSION -> runtimeConfig.version
)
)
}
def launcherConfigToRuntimeConfig(launcherConfig: LauncherConfig, missingPlaceholder: String): RuntimeConfig = {
import RuntimeConfig._
RuntimeConfig(
name = launcherConfig.branding.getOrElse(RuntimeConfig.Properties.PROFILE_NAME, missingPlaceholder),
version = launcherConfig.branding.getOrElse(RuntimeConfig.Properties.PROFILE_VERSION, missingPlaceholder),
startLevel = launcherConfig.startLevel,
defaultStartLevel = launcherConfig.defaultStartLevel,
frameworkProperties = launcherConfig.frameworkProperties,
systemProperties = launcherConfig.systemProperties,
bundles = BundleConfig(
url = missingPlaceholder,
jarName = new File(launcherConfig.frameworkJar).getName(),
sha1Sum = digestFile(new File(launcherConfig.frameworkJar)).orNull,
start = true,
startLevel = 0
) +:
launcherConfig.bundles.toList.map { b =>
BundleConfig(
url = missingPlaceholder,
jarName = new File(b.location).getName(),
sha1Sum = digestFile(new File(b.location)).orNull,
start = b.start,
startLevel = b.startLevel
)
},
properties = launcherConfig.branding
)
}
}
object ConfigConverter extends ConfigConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy