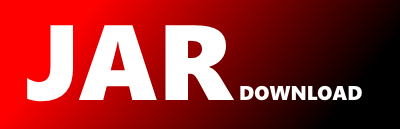
dev.ai4j.openai4j.chat.Function Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openai4j Show documentation
Show all versions of openai4j Show documentation
Java Client for OpenAI (ChatGPT)
package dev.ai4j.openai4j.chat;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.PropertyNamingStrategies;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonNaming;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import java.util.Objects;
@JsonDeserialize(builder = Function.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonNaming(PropertyNamingStrategies.SnakeCaseStrategy.class)
public class Function {
@JsonProperty
private final String name;
@JsonProperty
private final String description;
@JsonProperty
private final Boolean strict;
@JsonProperty
private final JsonObjectSchema parameters;
private Function(Builder builder) {
this.name = builder.name;
this.description = builder.description;
this.strict = builder.strict;
this.parameters = builder.parameters;
}
public String name() {
return name;
}
public String description() {
return description;
}
public Boolean strict() {
return strict;
}
public JsonObjectSchema parameters() {
return parameters;
}
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof Function
&& equalTo((Function) another);
}
private boolean equalTo(Function another) {
return Objects.equals(name, another.name)
&& Objects.equals(description, another.description)
&& Objects.equals(strict, another.strict)
&& Objects.equals(parameters, another.parameters);
}
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + Objects.hashCode(strict);
h += (h << 5) + Objects.hashCode(parameters);
return h;
}
@Override
public String toString() {
return "Function{"
+ "name=" + name
+ ", description=" + description
+ ", strict=" + strict
+ ", parameters=" + parameters
+ "}";
}
public static Builder builder() {
return new Builder();
}
@JsonPOJOBuilder(withPrefix = "")
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonNaming(PropertyNamingStrategies.SnakeCaseStrategy.class)
public static final class Builder {
private String name;
private String description;
private Boolean strict;
private JsonObjectSchema parameters = JsonObjectSchema.builder().build();
private Builder() {
}
public Builder name(String name) {
this.name = name;
return this;
}
public Builder description(String description) {
this.description = description;
return this;
}
public Builder strict(Boolean strict) {
this.strict = strict;
return this;
}
public Builder parameters(JsonObjectSchema parameters) {
this.parameters = parameters;
return this;
}
public Function build() {
return new Function(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy