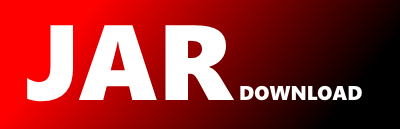
dev.ai4j.openai4j.chat.Delta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openai4j Show documentation
Show all versions of openai4j Show documentation
Java Client for OpenAI (ChatGPT)
package dev.ai4j.openai4j.chat;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.PropertyNamingStrategies;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonNaming;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import java.util.List;
import java.util.Objects;
import static java.util.Collections.unmodifiableList;
@JsonDeserialize(builder = Delta.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonNaming(PropertyNamingStrategies.SnakeCaseStrategy.class)
public final class Delta {
@JsonProperty
private final Role role;
@JsonProperty
private final String content;
@JsonProperty
private final List toolCalls;
@JsonProperty
@Deprecated
private final FunctionCall functionCall;
private Delta(Builder builder) {
this.role = builder.role;
this.content = builder.content;
this.toolCalls = builder.toolCalls;
this.functionCall = builder.functionCall;
}
public Role role() {
return role;
}
public String content() {
return content;
}
public List toolCalls() {
return toolCalls;
}
@Deprecated
public FunctionCall functionCall() {
return functionCall;
}
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof Delta
&& equalTo((Delta) another);
}
private boolean equalTo(Delta another) {
return Objects.equals(role, another.role)
&& Objects.equals(content, another.content)
&& Objects.equals(toolCalls, another.toolCalls)
&& Objects.equals(functionCall, another.functionCall);
}
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(role);
h += (h << 5) + Objects.hashCode(content);
h += (h << 5) + Objects.hashCode(toolCalls);
h += (h << 5) + Objects.hashCode(functionCall);
return h;
}
@Override
public String toString() {
return "Delta{"
+ "role=" + role
+ ", content=" + content
+ ", toolCalls=" + toolCalls
+ ", functionCall=" + functionCall
+ "}";
}
public static Builder builder() {
return new Builder();
}
@JsonPOJOBuilder(withPrefix = "")
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonNaming(PropertyNamingStrategies.SnakeCaseStrategy.class)
public static final class Builder {
private Role role;
private String content;
private List toolCalls;
@Deprecated
private FunctionCall functionCall;
private Builder() {
}
public Builder role(Role role) {
this.role = role;
return this;
}
public Builder content(String content) {
this.content = content;
return this;
}
public Builder toolCalls(List toolCalls) {
if (toolCalls != null) {
this.toolCalls = unmodifiableList(toolCalls);
}
return this;
}
@Deprecated
public Builder functionCall(FunctionCall functionCall) {
this.functionCall = functionCall;
return this;
}
public Delta build() {
return new Delta(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy