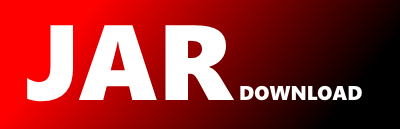
com.sedmelluq.discord.lavaplayer.player.AudioPlayerLifecycleManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lavaplayer Show documentation
Show all versions of lavaplayer Show documentation
A Lavaplayer fork maintained by Lavalink
The newest version!
package com.sedmelluq.discord.lavaplayer.player;
import com.sedmelluq.discord.lavaplayer.player.event.AudioEvent;
import com.sedmelluq.discord.lavaplayer.player.event.AudioEventListener;
import com.sedmelluq.discord.lavaplayer.player.event.TrackEndEvent;
import com.sedmelluq.discord.lavaplayer.player.event.TrackStartEvent;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicLong;
import java.util.concurrent.atomic.AtomicReference;
/**
* Triggers cleanup checks on all active audio players at a fixed interval.
*/
public class AudioPlayerLifecycleManager implements Runnable, AudioEventListener {
private static final long CHECK_INTERVAL = 10000;
private final ConcurrentMap activePlayers;
private final ScheduledExecutorService scheduler;
private final AtomicLong cleanupThreshold;
private final AtomicReference> scheduledTask;
/**
* @param scheduler Scheduler to use for the cleanup check task
* @param cleanupThreshold Threshold for player cleanup
*/
public AudioPlayerLifecycleManager(ScheduledExecutorService scheduler, AtomicLong cleanupThreshold) {
this.activePlayers = new ConcurrentHashMap<>();
this.scheduler = scheduler;
this.cleanupThreshold = cleanupThreshold;
this.scheduledTask = new AtomicReference<>();
}
/**
* Initialise the scheduled task.
*/
public void initialise() {
ScheduledFuture> task = scheduler.scheduleAtFixedRate(this, CHECK_INTERVAL, CHECK_INTERVAL, TimeUnit.MILLISECONDS);
if (!scheduledTask.compareAndSet(null, task)) {
task.cancel(false);
}
}
/**
* Stop the scheduled task.
*/
public void shutdown() {
ScheduledFuture> task = scheduledTask.getAndSet(null);
if (task != null) {
task.cancel(false);
}
}
@Override
public void onEvent(AudioEvent event) {
if (event instanceof TrackStartEvent) {
activePlayers.put(event.player, event.player);
} else if (event instanceof TrackEndEvent) {
activePlayers.remove(event.player);
}
}
@Override
public void run() {
for (AudioPlayer player : activePlayers.keySet()) {
player.checkCleanup(cleanupThreshold.get());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy