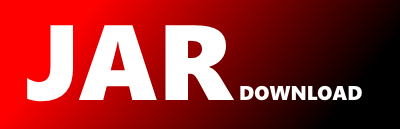
dev.cel.common.ast.AutoOneOf_CelConstant Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
package dev.cel.common.ast;
import com.google.common.primitives.UnsignedLong;
import com.google.protobuf.ByteString;
import com.google.protobuf.Duration;
import com.google.protobuf.NullValue;
import com.google.protobuf.Timestamp;
// Generated by com.google.auto.value.processor.AutoOneOfProcessor
final class AutoOneOf_CelConstant {
private AutoOneOf_CelConstant() {} // There are no instances of this type.
static CelConstant notSet(CelConstant.CelConstantNotSet notSet) {
if (notSet == null) {
throw new NullPointerException();
}
return new Impl_notSet(notSet);
}
static CelConstant nullValue(NullValue nullValue) {
if (nullValue == null) {
throw new NullPointerException();
}
return new Impl_nullValue(nullValue);
}
static CelConstant booleanValue(boolean booleanValue) {
return new Impl_booleanValue(booleanValue);
}
static CelConstant int64Value(long int64Value) {
return new Impl_int64Value(int64Value);
}
static CelConstant uint64Value(UnsignedLong uint64Value) {
if (uint64Value == null) {
throw new NullPointerException();
}
return new Impl_uint64Value(uint64Value);
}
static CelConstant doubleValue(double doubleValue) {
return new Impl_doubleValue(doubleValue);
}
static CelConstant stringValue(String stringValue) {
if (stringValue == null) {
throw new NullPointerException();
}
return new Impl_stringValue(stringValue);
}
static CelConstant bytesValue(ByteString bytesValue) {
if (bytesValue == null) {
throw new NullPointerException();
}
return new Impl_bytesValue(bytesValue);
}
static CelConstant timestampValue(Timestamp timestampValue) {
if (timestampValue == null) {
throw new NullPointerException();
}
return new Impl_timestampValue(timestampValue);
}
static CelConstant durationValue(Duration durationValue) {
if (durationValue == null) {
throw new NullPointerException();
}
return new Impl_durationValue(durationValue);
}
// Parent class that each implementation will inherit from.
private abstract static class Parent_ extends CelConstant {
@Override
public CelConstant.CelConstantNotSet notSet() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public NullValue nullValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public boolean booleanValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public long int64Value() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public UnsignedLong uint64Value() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public double doubleValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public String stringValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public ByteString bytesValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public Timestamp timestampValue() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public Duration durationValue() {
throw new UnsupportedOperationException(getKind().toString());
}
}
// Implementation when the contained property is "notSet".
private static final class Impl_notSet extends Parent_ {
private final CelConstant.CelConstantNotSet notSet;
Impl_notSet(CelConstant.CelConstantNotSet notSet) {
this.notSet = notSet;
}
@Override
public CelConstant.CelConstantNotSet notSet() {
return notSet;
}
@Override
public String toString() {
return "CelConstant{notSet=" + this.notSet + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.notSet.equals(that.notSet());
} else {
return false;
}
}
@Override
public int hashCode() {
return notSet.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.NOT_SET;
}
}
// Implementation when the contained property is "nullValue".
private static final class Impl_nullValue extends Parent_ {
private final NullValue nullValue;
Impl_nullValue(NullValue nullValue) {
this.nullValue = nullValue;
}
@Override
public NullValue nullValue() {
return nullValue;
}
@Override
public String toString() {
return "CelConstant{nullValue=" + this.nullValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.nullValue.equals(that.nullValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return nullValue.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.NULL_VALUE;
}
}
// Implementation when the contained property is "booleanValue".
private static final class Impl_booleanValue extends Parent_ {
private final boolean booleanValue;
Impl_booleanValue(boolean booleanValue) {
this.booleanValue = booleanValue;
}
@Override
public boolean booleanValue() {
return booleanValue;
}
@Override
public String toString() {
return "CelConstant{booleanValue=" + this.booleanValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.booleanValue == that.booleanValue();
} else {
return false;
}
}
@Override
public int hashCode() {
return booleanValue ? 1231 : 1237;
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.BOOLEAN_VALUE;
}
}
// Implementation when the contained property is "int64Value".
private static final class Impl_int64Value extends Parent_ {
private final long int64Value;
Impl_int64Value(long int64Value) {
this.int64Value = int64Value;
}
@Override
public long int64Value() {
return int64Value;
}
@Override
public String toString() {
return "CelConstant{int64Value=" + this.int64Value + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.int64Value == that.int64Value();
} else {
return false;
}
}
@Override
public int hashCode() {
return (int) ((int64Value >>> 32) ^ int64Value);
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.INT64_VALUE;
}
}
// Implementation when the contained property is "uint64Value".
private static final class Impl_uint64Value extends Parent_ {
private final UnsignedLong uint64Value;
Impl_uint64Value(UnsignedLong uint64Value) {
this.uint64Value = uint64Value;
}
@Override
public UnsignedLong uint64Value() {
return uint64Value;
}
@Override
public String toString() {
return "CelConstant{uint64Value=" + this.uint64Value + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.uint64Value.equals(that.uint64Value());
} else {
return false;
}
}
@Override
public int hashCode() {
return uint64Value.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.UINT64_VALUE;
}
}
// Implementation when the contained property is "doubleValue".
private static final class Impl_doubleValue extends Parent_ {
private final double doubleValue;
Impl_doubleValue(double doubleValue) {
this.doubleValue = doubleValue;
}
@Override
public double doubleValue() {
return doubleValue;
}
@Override
public String toString() {
return "CelConstant{doubleValue=" + this.doubleValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& Double.doubleToLongBits(this.doubleValue) == Double.doubleToLongBits(that.doubleValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return (int) ((Double.doubleToLongBits(doubleValue) >>> 32) ^ Double.doubleToLongBits(doubleValue));
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.DOUBLE_VALUE;
}
}
// Implementation when the contained property is "stringValue".
private static final class Impl_stringValue extends Parent_ {
private final String stringValue;
Impl_stringValue(String stringValue) {
this.stringValue = stringValue;
}
@Override
public String stringValue() {
return stringValue;
}
@Override
public String toString() {
return "CelConstant{stringValue=" + this.stringValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.stringValue.equals(that.stringValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return stringValue.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.STRING_VALUE;
}
}
// Implementation when the contained property is "bytesValue".
private static final class Impl_bytesValue extends Parent_ {
private final ByteString bytesValue;
Impl_bytesValue(ByteString bytesValue) {
this.bytesValue = bytesValue;
}
@Override
public ByteString bytesValue() {
return bytesValue;
}
@Override
public String toString() {
return "CelConstant{bytesValue=" + this.bytesValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.bytesValue.equals(that.bytesValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return bytesValue.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.BYTES_VALUE;
}
}
// Implementation when the contained property is "timestampValue".
private static final class Impl_timestampValue extends Parent_ {
private final Timestamp timestampValue;
Impl_timestampValue(Timestamp timestampValue) {
this.timestampValue = timestampValue;
}
@Override
public Timestamp timestampValue() {
return timestampValue;
}
@Override
public String toString() {
return "CelConstant{timestampValue=" + this.timestampValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.timestampValue.equals(that.timestampValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return timestampValue.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.TIMESTAMP_VALUE;
}
}
// Implementation when the contained property is "durationValue".
private static final class Impl_durationValue extends Parent_ {
private final Duration durationValue;
Impl_durationValue(Duration durationValue) {
this.durationValue = durationValue;
}
@Override
public Duration durationValue() {
return durationValue;
}
@Override
public String toString() {
return "CelConstant{durationValue=" + this.durationValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelConstant) {
CelConstant that = (CelConstant) x;
return this.getKind() == that.getKind()
&& this.durationValue.equals(that.durationValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return durationValue.hashCode();
}
@Override
public CelConstant.Kind getKind() {
return CelConstant.Kind.DURATION_VALUE;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy