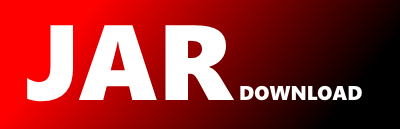
dev.cel.common.ast.AutoOneOf_CelExpr_ExprKind Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
package dev.cel.common.ast;
// Generated by com.google.auto.value.processor.AutoOneOfProcessor
final class AutoOneOf_CelExpr_ExprKind {
private AutoOneOf_CelExpr_ExprKind() {} // There are no instances of this type.
static CelExpr.ExprKind notSet(CelExpr.CelNotSet notSet) {
if (notSet == null) {
throw new NullPointerException();
}
return new Impl_notSet(notSet);
}
static CelExpr.ExprKind constant(CelConstant constant) {
if (constant == null) {
throw new NullPointerException();
}
return new Impl_constant(constant);
}
static CelExpr.ExprKind ident(CelExpr.CelIdent ident) {
if (ident == null) {
throw new NullPointerException();
}
return new Impl_ident(ident);
}
static CelExpr.ExprKind select(CelExpr.CelSelect select) {
if (select == null) {
throw new NullPointerException();
}
return new Impl_select(select);
}
static CelExpr.ExprKind call(CelExpr.CelCall call) {
if (call == null) {
throw new NullPointerException();
}
return new Impl_call(call);
}
static CelExpr.ExprKind list(CelExpr.CelList list) {
if (list == null) {
throw new NullPointerException();
}
return new Impl_list(list);
}
static CelExpr.ExprKind struct(CelExpr.CelStruct struct) {
if (struct == null) {
throw new NullPointerException();
}
return new Impl_struct(struct);
}
static CelExpr.ExprKind map(CelExpr.CelMap map) {
if (map == null) {
throw new NullPointerException();
}
return new Impl_map(map);
}
static CelExpr.ExprKind comprehension(CelExpr.CelComprehension comprehension) {
if (comprehension == null) {
throw new NullPointerException();
}
return new Impl_comprehension(comprehension);
}
// Parent class that each implementation will inherit from.
private abstract static class Parent_ extends CelExpr.ExprKind {
@Override
public CelExpr.CelNotSet notSet() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelConstant constant() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelIdent ident() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelSelect select() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelCall call() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelList list() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelStruct struct() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelMap map() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public CelExpr.CelComprehension comprehension() {
throw new UnsupportedOperationException(getKind().toString());
}
}
// Implementation when the contained property is "notSet".
private static final class Impl_notSet extends Parent_ {
private final CelExpr.CelNotSet notSet;
Impl_notSet(CelExpr.CelNotSet notSet) {
this.notSet = notSet;
}
@Override
public CelExpr.CelNotSet notSet() {
return notSet;
}
@Override
public String toString() {
return "ExprKind{notSet=" + this.notSet + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.notSet.equals(that.notSet());
} else {
return false;
}
}
@Override
public int hashCode() {
return notSet.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.NOT_SET;
}
}
// Implementation when the contained property is "constant".
private static final class Impl_constant extends Parent_ {
private final CelConstant constant;
Impl_constant(CelConstant constant) {
this.constant = constant;
}
@Override
public CelConstant constant() {
return constant;
}
@Override
public String toString() {
return "ExprKind{constant=" + this.constant + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.constant.equals(that.constant());
} else {
return false;
}
}
@Override
public int hashCode() {
return constant.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.CONSTANT;
}
}
// Implementation when the contained property is "ident".
private static final class Impl_ident extends Parent_ {
private final CelExpr.CelIdent ident;
Impl_ident(CelExpr.CelIdent ident) {
this.ident = ident;
}
@Override
public CelExpr.CelIdent ident() {
return ident;
}
@Override
public String toString() {
return "ExprKind{ident=" + this.ident + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.ident.equals(that.ident());
} else {
return false;
}
}
@Override
public int hashCode() {
return ident.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.IDENT;
}
}
// Implementation when the contained property is "select".
private static final class Impl_select extends Parent_ {
private final CelExpr.CelSelect select;
Impl_select(CelExpr.CelSelect select) {
this.select = select;
}
@Override
public CelExpr.CelSelect select() {
return select;
}
@Override
public String toString() {
return "ExprKind{select=" + this.select + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.select.equals(that.select());
} else {
return false;
}
}
@Override
public int hashCode() {
return select.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.SELECT;
}
}
// Implementation when the contained property is "call".
private static final class Impl_call extends Parent_ {
private final CelExpr.CelCall call;
Impl_call(CelExpr.CelCall call) {
this.call = call;
}
@Override
public CelExpr.CelCall call() {
return call;
}
@Override
public String toString() {
return "ExprKind{call=" + this.call + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.call.equals(that.call());
} else {
return false;
}
}
@Override
public int hashCode() {
return call.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.CALL;
}
}
// Implementation when the contained property is "list".
private static final class Impl_list extends Parent_ {
private final CelExpr.CelList list;
Impl_list(CelExpr.CelList list) {
this.list = list;
}
@Override
public CelExpr.CelList list() {
return list;
}
@Override
public String toString() {
return "ExprKind{list=" + this.list + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.list.equals(that.list());
} else {
return false;
}
}
@Override
public int hashCode() {
return list.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.LIST;
}
}
// Implementation when the contained property is "struct".
private static final class Impl_struct extends Parent_ {
private final CelExpr.CelStruct struct;
Impl_struct(CelExpr.CelStruct struct) {
this.struct = struct;
}
@Override
public CelExpr.CelStruct struct() {
return struct;
}
@Override
public String toString() {
return "ExprKind{struct=" + this.struct + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.struct.equals(that.struct());
} else {
return false;
}
}
@Override
public int hashCode() {
return struct.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.STRUCT;
}
}
// Implementation when the contained property is "map".
private static final class Impl_map extends Parent_ {
private final CelExpr.CelMap map;
Impl_map(CelExpr.CelMap map) {
this.map = map;
}
@Override
public CelExpr.CelMap map() {
return map;
}
@Override
public String toString() {
return "ExprKind{map=" + this.map + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.map.equals(that.map());
} else {
return false;
}
}
@Override
public int hashCode() {
return map.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.MAP;
}
}
// Implementation when the contained property is "comprehension".
private static final class Impl_comprehension extends Parent_ {
private final CelExpr.CelComprehension comprehension;
Impl_comprehension(CelExpr.CelComprehension comprehension) {
this.comprehension = comprehension;
}
@Override
public CelExpr.CelComprehension comprehension() {
return comprehension;
}
@Override
public String toString() {
return "ExprKind{comprehension=" + this.comprehension + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof CelExpr.ExprKind) {
CelExpr.ExprKind that = (CelExpr.ExprKind) x;
return this.getKind() == that.getKind()
&& this.comprehension.equals(that.comprehension());
} else {
return false;
}
}
@Override
public int hashCode() {
return comprehension.hashCode();
}
@Override
public CelExpr.ExprKind.Kind getKind() {
return CelExpr.ExprKind.Kind.COMPREHENSION;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy