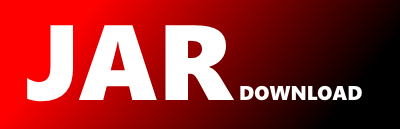
dev.cel.common.ast.AutoValue_CelExpr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
package dev.cel.common.ast;
@SuppressWarnings("unchecked")
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_CelExpr extends CelExpr {
private final long id;
private final CelExpr.ExprKind exprKind;
private AutoValue_CelExpr(
long id,
CelExpr.ExprKind exprKind) {
this.id = id;
this.exprKind = exprKind;
}
@Override
public long id() {
return id;
}
@Override
public CelExpr.ExprKind exprKind() {
return exprKind;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CelExpr) {
CelExpr that = (CelExpr) o;
return this.id == that.id()
&& this.exprKind.equals(that.exprKind());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (int) ((id >>> 32) ^ id);
h$ *= 1000003;
h$ ^= exprKind.hashCode();
return h$;
}
@Override
public CelExpr.Builder toBuilder() {
return new AutoValue_CelExpr.Builder(this);
}
static final class Builder extends CelExpr.Builder {
private long id;
private CelExpr.ExprKind exprKind;
private byte set$0;
Builder() {
}
Builder(CelExpr source) {
this.id = source.id();
this.exprKind = source.exprKind();
set$0 = (byte) 1;
}
@Override
public CelExpr.Builder setId(long id) {
this.id = id;
set$0 |= (byte) 1;
return this;
}
@Override
public long id() {
if ((set$0 & 1) == 0) {
throw new IllegalStateException("Property \"id\" has not been set");
}
return id;
}
@Override
public CelExpr.Builder setExprKind(CelExpr.ExprKind exprKind) {
if (exprKind == null) {
throw new NullPointerException("Null exprKind");
}
this.exprKind = exprKind;
return this;
}
@Override
public CelExpr.ExprKind exprKind() {
if (this.exprKind == null) {
throw new IllegalStateException("Property \"exprKind\" has not been set");
}
return exprKind;
}
@Override
public CelExpr build() {
if (set$0 != 1
|| this.exprKind == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" id");
}
if (this.exprKind == null) {
missing.append(" exprKind");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CelExpr(
this.id,
this.exprKind);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy