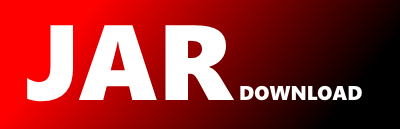
dev.cel.common.ast.AutoValue_CelExpr_CelList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
package dev.cel.common.ast;
import com.google.common.collect.ImmutableList;
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_CelExpr_CelList extends CelExpr.CelList {
private final ImmutableList elements;
private final ImmutableList optionalIndices;
private AutoValue_CelExpr_CelList(
ImmutableList elements,
ImmutableList optionalIndices) {
this.elements = elements;
this.optionalIndices = optionalIndices;
}
@Override
public ImmutableList elements() {
return elements;
}
@Override
public ImmutableList optionalIndices() {
return optionalIndices;
}
@Override
public String toString() {
return "CelList{"
+ "elements=" + elements + ", "
+ "optionalIndices=" + optionalIndices
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CelExpr.CelList) {
CelExpr.CelList that = (CelExpr.CelList) o;
return this.elements.equals(that.elements())
&& this.optionalIndices.equals(that.optionalIndices());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= elements.hashCode();
h$ *= 1000003;
h$ ^= optionalIndices.hashCode();
return h$;
}
@Override
CelExpr.CelList.Builder autoToBuilder() {
return new AutoValue_CelExpr_CelList.Builder(this);
}
static final class Builder extends CelExpr.CelList.Builder {
private ImmutableList elements;
private ImmutableList.Builder optionalIndicesBuilder$;
private ImmutableList optionalIndices;
Builder() {
}
Builder(CelExpr.CelList source) {
this.elements = source.elements();
this.optionalIndices = source.optionalIndices();
}
@Override
CelExpr.CelList.Builder setElements(ImmutableList elements) {
if (elements == null) {
throw new NullPointerException("Null elements");
}
this.elements = elements;
return this;
}
@Override
ImmutableList elements() {
if (this.elements == null) {
throw new IllegalStateException("Property \"elements\" has not been set");
}
return elements;
}
@Override
ImmutableList.Builder optionalIndicesBuilder() {
if (optionalIndicesBuilder$ == null) {
if (optionalIndices == null) {
optionalIndicesBuilder$ = ImmutableList.builder();
} else {
optionalIndicesBuilder$ = ImmutableList.builder();
optionalIndicesBuilder$.addAll(optionalIndices);
optionalIndices = null;
}
}
return optionalIndicesBuilder$;
}
@Override
CelExpr.CelList autoBuild() {
if (optionalIndicesBuilder$ != null) {
this.optionalIndices = optionalIndicesBuilder$.build();
} else if (this.optionalIndices == null) {
this.optionalIndices = ImmutableList.of();
}
if (this.elements == null) {
String missing = " elements";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CelExpr_CelList(
this.elements,
this.optionalIndices);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy