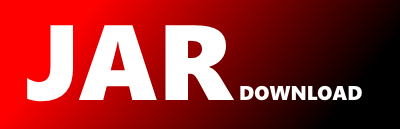
dev.cel.expr.Reference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: cel/expr/checked.proto
// Protobuf Java Version: 4.28.2
package dev.cel.expr;
/**
*
* Describes a resolved reference to a declaration.
*
*
* Protobuf type {@code cel.expr.Reference}
*/
public final class Reference extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:cel.expr.Reference)
ReferenceOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Reference.class.getName());
}
// Use Reference.newBuilder() to construct.
private Reference(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Reference() {
name_ = "";
overloadId_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dev.cel.expr.DeclProto.internal_static_cel_expr_Reference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return dev.cel.expr.DeclProto.internal_static_cel_expr_Reference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dev.cel.expr.Reference.class, dev.cel.expr.Reference.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OVERLOAD_ID_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList overloadId_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @return A list containing the overloadId.
*/
public com.google.protobuf.ProtocolStringList
getOverloadIdList() {
return overloadId_;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @return The count of overloadId.
*/
public int getOverloadIdCount() {
return overloadId_.size();
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param index The index of the element to return.
* @return The overloadId at the given index.
*/
public java.lang.String getOverloadId(int index) {
return overloadId_.get(index);
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param index The index of the value to return.
* @return The bytes of the overloadId at the given index.
*/
public com.google.protobuf.ByteString
getOverloadIdBytes(int index) {
return overloadId_.getByteString(index);
}
public static final int VALUE_FIELD_NUMBER = 4;
private dev.cel.expr.Constant value_;
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
* @return The value.
*/
@java.lang.Override
public dev.cel.expr.Constant getValue() {
return value_ == null ? dev.cel.expr.Constant.getDefaultInstance() : value_;
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
@java.lang.Override
public dev.cel.expr.ConstantOrBuilder getValueOrBuilder() {
return value_ == null ? dev.cel.expr.Constant.getDefaultInstance() : value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, name_);
}
for (int i = 0; i < overloadId_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, overloadId_.getRaw(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(4, getValue());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, name_);
}
{
int dataSize = 0;
for (int i = 0; i < overloadId_.size(); i++) {
dataSize += computeStringSizeNoTag(overloadId_.getRaw(i));
}
size += dataSize;
size += 1 * getOverloadIdList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dev.cel.expr.Reference)) {
return super.equals(obj);
}
dev.cel.expr.Reference other = (dev.cel.expr.Reference) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getOverloadIdList()
.equals(other.getOverloadIdList())) return false;
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (getOverloadIdCount() > 0) {
hash = (37 * hash) + OVERLOAD_ID_FIELD_NUMBER;
hash = (53 * hash) + getOverloadIdList().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static dev.cel.expr.Reference parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.Reference parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.Reference parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.Reference parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.Reference parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.Reference parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.Reference parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static dev.cel.expr.Reference parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dev.cel.expr.Reference parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static dev.cel.expr.Reference parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dev.cel.expr.Reference parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static dev.cel.expr.Reference parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dev.cel.expr.Reference prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a resolved reference to a declaration.
*
*
* Protobuf type {@code cel.expr.Reference}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:cel.expr.Reference)
dev.cel.expr.ReferenceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dev.cel.expr.DeclProto.internal_static_cel_expr_Reference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return dev.cel.expr.DeclProto.internal_static_cel_expr_Reference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dev.cel.expr.Reference.class, dev.cel.expr.Reference.Builder.class);
}
// Construct using dev.cel.expr.Reference.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
overloadId_ =
com.google.protobuf.LazyStringArrayList.emptyList();
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dev.cel.expr.DeclProto.internal_static_cel_expr_Reference_descriptor;
}
@java.lang.Override
public dev.cel.expr.Reference getDefaultInstanceForType() {
return dev.cel.expr.Reference.getDefaultInstance();
}
@java.lang.Override
public dev.cel.expr.Reference build() {
dev.cel.expr.Reference result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dev.cel.expr.Reference buildPartial() {
dev.cel.expr.Reference result = new dev.cel.expr.Reference(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(dev.cel.expr.Reference result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
overloadId_.makeImmutable();
result.overloadId_ = overloadId_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.value_ = valueBuilder_ == null
? value_
: valueBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dev.cel.expr.Reference) {
return mergeFrom((dev.cel.expr.Reference)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dev.cel.expr.Reference other) {
if (other == dev.cel.expr.Reference.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.overloadId_.isEmpty()) {
if (overloadId_.isEmpty()) {
overloadId_ = other.overloadId_;
bitField0_ |= 0x00000002;
} else {
ensureOverloadIdIsMutable();
overloadId_.addAll(other.overloadId_);
}
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 26: {
java.lang.String s = input.readStringRequireUtf8();
ensureOverloadIdIsMutable();
overloadId_.add(s);
break;
} // case 26
case 34: {
input.readMessage(
getValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The fully qualified name of the declaration.
*
*
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList overloadId_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureOverloadIdIsMutable() {
if (!overloadId_.isModifiable()) {
overloadId_ = new com.google.protobuf.LazyStringArrayList(overloadId_);
}
bitField0_ |= 0x00000002;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @return A list containing the overloadId.
*/
public com.google.protobuf.ProtocolStringList
getOverloadIdList() {
overloadId_.makeImmutable();
return overloadId_;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @return The count of overloadId.
*/
public int getOverloadIdCount() {
return overloadId_.size();
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param index The index of the element to return.
* @return The overloadId at the given index.
*/
public java.lang.String getOverloadId(int index) {
return overloadId_.get(index);
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param index The index of the value to return.
* @return The bytes of the overloadId at the given index.
*/
public com.google.protobuf.ByteString
getOverloadIdBytes(int index) {
return overloadId_.getByteString(index);
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param index The index to set the value at.
* @param value The overloadId to set.
* @return This builder for chaining.
*/
public Builder setOverloadId(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureOverloadIdIsMutable();
overloadId_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param value The overloadId to add.
* @return This builder for chaining.
*/
public Builder addOverloadId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureOverloadIdIsMutable();
overloadId_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param values The overloadId to add.
* @return This builder for chaining.
*/
public Builder addAllOverloadId(
java.lang.Iterable values) {
ensureOverloadIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, overloadId_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @return This builder for chaining.
*/
public Builder clearOverloadId() {
overloadId_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);;
onChanged();
return this;
}
/**
*
* For references to functions, this is a list of `Overload.overload_id`
* values which match according to typing rules.
*
* If the list has more than one element, overload resolution among the
* presented candidates must happen at runtime because of dynamic types. The
* type checker attempts to narrow down this list as much as possible.
*
* Empty if this is not a reference to a
* [Decl.FunctionDecl][cel.expr.Decl.FunctionDecl].
*
*
* repeated string overload_id = 3;
* @param value The bytes of the overloadId to add.
* @return This builder for chaining.
*/
public Builder addOverloadIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureOverloadIdIsMutable();
overloadId_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private dev.cel.expr.Constant value_;
private com.google.protobuf.SingleFieldBuilder<
dev.cel.expr.Constant, dev.cel.expr.Constant.Builder, dev.cel.expr.ConstantOrBuilder> valueBuilder_;
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
* @return The value.
*/
public dev.cel.expr.Constant getValue() {
if (valueBuilder_ == null) {
return value_ == null ? dev.cel.expr.Constant.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public Builder setValue(dev.cel.expr.Constant value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public Builder setValue(
dev.cel.expr.Constant.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public Builder mergeValue(dev.cel.expr.Constant value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
value_ != null &&
value_ != dev.cel.expr.Constant.getDefaultInstance()) {
getValueBuilder().mergeFrom(value);
} else {
value_ = value;
}
} else {
valueBuilder_.mergeFrom(value);
}
if (value_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000004);
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public dev.cel.expr.Constant.Builder getValueBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
public dev.cel.expr.ConstantOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
dev.cel.expr.Constant.getDefaultInstance() : value_;
}
}
/**
*
* For references to constants, this may contain the value of the
* constant if known at compile time.
*
*
* .cel.expr.Constant value = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
dev.cel.expr.Constant, dev.cel.expr.Constant.Builder, dev.cel.expr.ConstantOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
dev.cel.expr.Constant, dev.cel.expr.Constant.Builder, dev.cel.expr.ConstantOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
// @@protoc_insertion_point(builder_scope:cel.expr.Reference)
}
// @@protoc_insertion_point(class_scope:cel.expr.Reference)
private static final dev.cel.expr.Reference DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dev.cel.expr.Reference();
}
public static dev.cel.expr.Reference getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Reference parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dev.cel.expr.Reference getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy