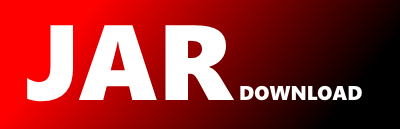
dev.cel.expr.UnknownSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime Show documentation
Show all versions of runtime Show documentation
Common Expression Language Runtime for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: cel/expr/eval.proto
// Protobuf Java Version: 4.28.2
package dev.cel.expr;
/**
*
* A set of expressions for which the value is unknown.
*
* The unknowns included depend on the context. See `ExprValue.unknown`.
*
*
* Protobuf type {@code cel.expr.UnknownSet}
*/
public final class UnknownSet extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:cel.expr.UnknownSet)
UnknownSetOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
UnknownSet.class.getName());
}
// Use UnknownSet.newBuilder() to construct.
private UnknownSet(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private UnknownSet() {
exprs_ = emptyLongList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dev.cel.expr.EvalProto.internal_static_cel_expr_UnknownSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return dev.cel.expr.EvalProto.internal_static_cel_expr_UnknownSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dev.cel.expr.UnknownSet.class, dev.cel.expr.UnknownSet.Builder.class);
}
public static final int EXPRS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.LongList exprs_ =
emptyLongList();
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @return A list containing the exprs.
*/
@java.lang.Override
public java.util.List
getExprsList() {
return exprs_;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @return The count of exprs.
*/
public int getExprsCount() {
return exprs_.size();
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @param index The index of the element to return.
* @return The exprs at the given index.
*/
public long getExprs(int index) {
return exprs_.getLong(index);
}
private int exprsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getExprsList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(exprsMemoizedSerializedSize);
}
for (int i = 0; i < exprs_.size(); i++) {
output.writeInt64NoTag(exprs_.getLong(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < exprs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(exprs_.getLong(i));
}
size += dataSize;
if (!getExprsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
exprsMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof dev.cel.expr.UnknownSet)) {
return super.equals(obj);
}
dev.cel.expr.UnknownSet other = (dev.cel.expr.UnknownSet) obj;
if (!getExprsList()
.equals(other.getExprsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getExprsCount() > 0) {
hash = (37 * hash) + EXPRS_FIELD_NUMBER;
hash = (53 * hash) + getExprsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static dev.cel.expr.UnknownSet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.UnknownSet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.UnknownSet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.UnknownSet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.UnknownSet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static dev.cel.expr.UnknownSet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static dev.cel.expr.UnknownSet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static dev.cel.expr.UnknownSet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static dev.cel.expr.UnknownSet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static dev.cel.expr.UnknownSet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static dev.cel.expr.UnknownSet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static dev.cel.expr.UnknownSet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(dev.cel.expr.UnknownSet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A set of expressions for which the value is unknown.
*
* The unknowns included depend on the context. See `ExprValue.unknown`.
*
*
* Protobuf type {@code cel.expr.UnknownSet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:cel.expr.UnknownSet)
dev.cel.expr.UnknownSetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return dev.cel.expr.EvalProto.internal_static_cel_expr_UnknownSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return dev.cel.expr.EvalProto.internal_static_cel_expr_UnknownSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
dev.cel.expr.UnknownSet.class, dev.cel.expr.UnknownSet.Builder.class);
}
// Construct using dev.cel.expr.UnknownSet.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
exprs_ = emptyLongList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return dev.cel.expr.EvalProto.internal_static_cel_expr_UnknownSet_descriptor;
}
@java.lang.Override
public dev.cel.expr.UnknownSet getDefaultInstanceForType() {
return dev.cel.expr.UnknownSet.getDefaultInstance();
}
@java.lang.Override
public dev.cel.expr.UnknownSet build() {
dev.cel.expr.UnknownSet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public dev.cel.expr.UnknownSet buildPartial() {
dev.cel.expr.UnknownSet result = new dev.cel.expr.UnknownSet(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(dev.cel.expr.UnknownSet result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
exprs_.makeImmutable();
result.exprs_ = exprs_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof dev.cel.expr.UnknownSet) {
return mergeFrom((dev.cel.expr.UnknownSet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(dev.cel.expr.UnknownSet other) {
if (other == dev.cel.expr.UnknownSet.getDefaultInstance()) return this;
if (!other.exprs_.isEmpty()) {
if (exprs_.isEmpty()) {
exprs_ = other.exprs_;
exprs_.makeImmutable();
bitField0_ |= 0x00000001;
} else {
ensureExprsIsMutable();
exprs_.addAll(other.exprs_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
long v = input.readInt64();
ensureExprsIsMutable();
exprs_.addLong(v);
break;
} // case 8
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureExprsIsMutable();
while (input.getBytesUntilLimit() > 0) {
exprs_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.LongList exprs_ = emptyLongList();
private void ensureExprsIsMutable() {
if (!exprs_.isModifiable()) {
exprs_ = makeMutableCopy(exprs_);
}
bitField0_ |= 0x00000001;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @return A list containing the exprs.
*/
public java.util.List
getExprsList() {
exprs_.makeImmutable();
return exprs_;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @return The count of exprs.
*/
public int getExprsCount() {
return exprs_.size();
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @param index The index of the element to return.
* @return The exprs at the given index.
*/
public long getExprs(int index) {
return exprs_.getLong(index);
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @param index The index to set the value at.
* @param value The exprs to set.
* @return This builder for chaining.
*/
public Builder setExprs(
int index, long value) {
ensureExprsIsMutable();
exprs_.setLong(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @param value The exprs to add.
* @return This builder for chaining.
*/
public Builder addExprs(long value) {
ensureExprsIsMutable();
exprs_.addLong(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @param values The exprs to add.
* @return This builder for chaining.
*/
public Builder addAllExprs(
java.lang.Iterable extends java.lang.Long> values) {
ensureExprsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, exprs_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The ids of the expressions with unknown values.
*
*
* repeated int64 exprs = 1;
* @return This builder for chaining.
*/
public Builder clearExprs() {
exprs_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:cel.expr.UnknownSet)
}
// @@protoc_insertion_point(class_scope:cel.expr.UnknownSet)
private static final dev.cel.expr.UnknownSet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new dev.cel.expr.UnknownSet();
}
public static dev.cel.expr.UnknownSet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UnknownSet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public dev.cel.expr.UnknownSet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy