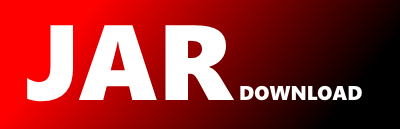
dev.cel.common.AutoValue_CelIssue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of validators Show documentation
Show all versions of validators Show documentation
Common Expression Language Validators for Java
The newest version!
package dev.cel.common;
import org.jspecify.nullness.Nullable;
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_CelIssue extends CelIssue {
private final CelIssue.Severity severity;
private final CelSourceLocation sourceLocation;
private final String message;
private AutoValue_CelIssue(
CelIssue.Severity severity,
CelSourceLocation sourceLocation,
String message) {
this.severity = severity;
this.sourceLocation = sourceLocation;
this.message = message;
}
@Override
public CelIssue.Severity getSeverity() {
return severity;
}
@Override
public CelSourceLocation getSourceLocation() {
return sourceLocation;
}
@Override
public String getMessage() {
return message;
}
@Override
public String toString() {
return "CelIssue{"
+ "severity=" + severity + ", "
+ "sourceLocation=" + sourceLocation + ", "
+ "message=" + message
+ "}";
}
@Override
public boolean equals(@Nullable Object o) {
if (o == this) {
return true;
}
if (o instanceof CelIssue) {
CelIssue that = (CelIssue) o;
return this.severity.equals(that.getSeverity())
&& this.sourceLocation.equals(that.getSourceLocation())
&& this.message.equals(that.getMessage());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= severity.hashCode();
h$ *= 1000003;
h$ ^= sourceLocation.hashCode();
h$ *= 1000003;
h$ ^= message.hashCode();
return h$;
}
static final class Builder extends CelIssue.Builder {
private CelIssue.@Nullable Severity severity;
private @Nullable CelSourceLocation sourceLocation;
private @Nullable String message;
Builder() {
}
@Override
public CelIssue.Builder setSeverity(CelIssue.Severity severity) {
if (severity == null) {
throw new NullPointerException("Null severity");
}
this.severity = severity;
return this;
}
@Override
public CelIssue.Builder setSourceLocation(CelSourceLocation sourceLocation) {
if (sourceLocation == null) {
throw new NullPointerException("Null sourceLocation");
}
this.sourceLocation = sourceLocation;
return this;
}
@Override
public CelIssue.Builder setMessage(String message) {
if (message == null) {
throw new NullPointerException("Null message");
}
this.message = message;
return this;
}
@Override
public CelIssue build() {
if (this.severity == null
|| this.sourceLocation == null
|| this.message == null) {
StringBuilder missing = new StringBuilder();
if (this.severity == null) {
missing.append(" severity");
}
if (this.sourceLocation == null) {
missing.append(" sourceLocation");
}
if (this.message == null) {
missing.append(" message");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CelIssue(
this.severity,
this.sourceLocation,
this.message);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy