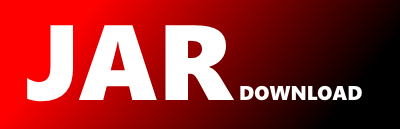
dev.cel.common.AutoValue_CelOverloadDecl Maven / Gradle / Ivy
package dev.cel.common;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import dev.cel.common.types.CelType;
import org.jspecify.nullness.Nullable;
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_CelOverloadDecl extends CelOverloadDecl {
private final String overloadId;
private final ImmutableList parameterTypes;
private final ImmutableSet typeParameterNames;
private final CelType resultType;
private final boolean isInstanceFunction;
private final String doc;
private AutoValue_CelOverloadDecl(
String overloadId,
ImmutableList parameterTypes,
ImmutableSet typeParameterNames,
CelType resultType,
boolean isInstanceFunction,
String doc) {
this.overloadId = overloadId;
this.parameterTypes = parameterTypes;
this.typeParameterNames = typeParameterNames;
this.resultType = resultType;
this.isInstanceFunction = isInstanceFunction;
this.doc = doc;
}
@Override
public String overloadId() {
return overloadId;
}
@Override
public ImmutableList parameterTypes() {
return parameterTypes;
}
@Override
public ImmutableSet typeParameterNames() {
return typeParameterNames;
}
@Override
public CelType resultType() {
return resultType;
}
@Override
public boolean isInstanceFunction() {
return isInstanceFunction;
}
@Override
public String doc() {
return doc;
}
@Override
public String toString() {
return "CelOverloadDecl{"
+ "overloadId=" + overloadId + ", "
+ "parameterTypes=" + parameterTypes + ", "
+ "typeParameterNames=" + typeParameterNames + ", "
+ "resultType=" + resultType + ", "
+ "isInstanceFunction=" + isInstanceFunction + ", "
+ "doc=" + doc
+ "}";
}
@Override
public boolean equals(@Nullable Object o) {
if (o == this) {
return true;
}
if (o instanceof CelOverloadDecl) {
CelOverloadDecl that = (CelOverloadDecl) o;
return this.overloadId.equals(that.overloadId())
&& this.parameterTypes.equals(that.parameterTypes())
&& this.typeParameterNames.equals(that.typeParameterNames())
&& this.resultType.equals(that.resultType())
&& this.isInstanceFunction == that.isInstanceFunction()
&& this.doc.equals(that.doc());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= overloadId.hashCode();
h$ *= 1000003;
h$ ^= parameterTypes.hashCode();
h$ *= 1000003;
h$ ^= typeParameterNames.hashCode();
h$ *= 1000003;
h$ ^= resultType.hashCode();
h$ *= 1000003;
h$ ^= isInstanceFunction ? 1231 : 1237;
h$ *= 1000003;
h$ ^= doc.hashCode();
return h$;
}
@Override
public CelOverloadDecl.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends CelOverloadDecl.Builder {
private @Nullable String overloadId;
private ImmutableList.@Nullable Builder parameterTypesBuilder$;
private @Nullable ImmutableList parameterTypes;
private @Nullable ImmutableSet typeParameterNames;
private @Nullable CelType resultType;
private boolean isInstanceFunction;
private @Nullable String doc;
private byte set$0;
Builder() {
}
private Builder(CelOverloadDecl source) {
this.overloadId = source.overloadId();
this.parameterTypes = source.parameterTypes();
this.typeParameterNames = source.typeParameterNames();
this.resultType = source.resultType();
this.isInstanceFunction = source.isInstanceFunction();
this.doc = source.doc();
set$0 = (byte) 1;
}
@Override
public CelOverloadDecl.Builder setOverloadId(String overloadId) {
if (overloadId == null) {
throw new NullPointerException("Null overloadId");
}
this.overloadId = overloadId;
return this;
}
@Override
public CelOverloadDecl.Builder setParameterTypes(ImmutableSet parameterTypes) {
if (parameterTypesBuilder$ != null) {
throw new IllegalStateException("Cannot set parameterTypes after calling parameterTypesBuilder()");
}
this.parameterTypes = ImmutableList.copyOf(parameterTypes);
return this;
}
@Override
ImmutableList.Builder parameterTypesBuilder() {
if (parameterTypesBuilder$ == null) {
if (parameterTypes == null) {
parameterTypesBuilder$ = ImmutableList.builder();
} else {
parameterTypesBuilder$ = ImmutableList.builder();
parameterTypesBuilder$.addAll(parameterTypes);
parameterTypes = null;
}
}
return parameterTypesBuilder$;
}
@Override
public ImmutableList parameterTypes() {
if (parameterTypesBuilder$ != null) {
return parameterTypesBuilder$.build();
}
if (parameterTypes == null) {
parameterTypes = ImmutableList.of();
}
return parameterTypes;
}
@Override
CelOverloadDecl.Builder setTypeParameterNames(ImmutableSet typeParameterNames) {
if (typeParameterNames == null) {
throw new NullPointerException("Null typeParameterNames");
}
this.typeParameterNames = typeParameterNames;
return this;
}
@Override
public CelOverloadDecl.Builder setResultType(CelType resultType) {
if (resultType == null) {
throw new NullPointerException("Null resultType");
}
this.resultType = resultType;
return this;
}
@Override
public CelType resultType() {
if (this.resultType == null) {
throw new IllegalStateException("Property \"resultType\" has not been set");
}
return resultType;
}
@Override
public CelOverloadDecl.Builder setIsInstanceFunction(boolean isInstanceFunction) {
this.isInstanceFunction = isInstanceFunction;
set$0 |= (byte) 1;
return this;
}
@Override
public boolean isInstanceFunction() {
if ((set$0 & 1) == 0) {
throw new IllegalStateException("Property \"isInstanceFunction\" has not been set");
}
return isInstanceFunction;
}
@Override
public CelOverloadDecl.Builder setDoc(String doc) {
if (doc == null) {
throw new NullPointerException("Null doc");
}
this.doc = doc;
return this;
}
@Override
CelOverloadDecl autoBuild() {
if (parameterTypesBuilder$ != null) {
this.parameterTypes = parameterTypesBuilder$.build();
} else if (this.parameterTypes == null) {
this.parameterTypes = ImmutableList.of();
}
if (set$0 != 1
|| this.overloadId == null
|| this.typeParameterNames == null
|| this.resultType == null
|| this.doc == null) {
StringBuilder missing = new StringBuilder();
if (this.overloadId == null) {
missing.append(" overloadId");
}
if (this.typeParameterNames == null) {
missing.append(" typeParameterNames");
}
if (this.resultType == null) {
missing.append(" resultType");
}
if ((set$0 & 1) == 0) {
missing.append(" isInstanceFunction");
}
if (this.doc == null) {
missing.append(" doc");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CelOverloadDecl(
this.overloadId,
this.parameterTypes,
this.typeParameterNames,
this.resultType,
this.isInstanceFunction,
this.doc);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy