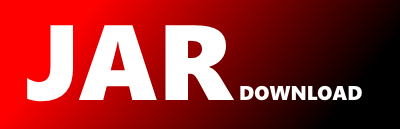
dev.cel.parser.AutoValue_CelMacro Maven / Gradle / Ivy
package dev.cel.parser;
import org.jspecify.nullness.Nullable;
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_CelMacro extends CelMacro {
private final String function;
private final int argumentCount;
private final boolean receiverStyle;
private final String key;
private final boolean variadic;
private final CelMacroExpander expander;
private AutoValue_CelMacro(
String function,
int argumentCount,
boolean receiverStyle,
String key,
boolean variadic,
CelMacroExpander expander) {
this.function = function;
this.argumentCount = argumentCount;
this.receiverStyle = receiverStyle;
this.key = key;
this.variadic = variadic;
this.expander = expander;
}
@Override
public String getFunction() {
return function;
}
@Override
public int getArgumentCount() {
return argumentCount;
}
@Override
boolean getReceiverStyle() {
return receiverStyle;
}
@Override
public String getKey() {
return key;
}
@Override
boolean getVariadic() {
return variadic;
}
@Override
public CelMacroExpander getExpander() {
return expander;
}
static final class Builder extends CelMacro.Builder {
private @Nullable String function;
private int argumentCount;
private boolean receiverStyle;
private @Nullable String key;
private boolean variadic;
private @Nullable CelMacroExpander expander;
private byte set$0;
Builder() {
}
@Override
CelMacro.Builder setFunction(String function) {
if (function == null) {
throw new NullPointerException("Null function");
}
this.function = function;
return this;
}
@Override
CelMacro.Builder setArgumentCount(int argumentCount) {
this.argumentCount = argumentCount;
set$0 |= (byte) 1;
return this;
}
@Override
CelMacro.Builder setReceiverStyle(boolean receiverStyle) {
this.receiverStyle = receiverStyle;
set$0 |= (byte) 2;
return this;
}
@Override
CelMacro.Builder setKey(String key) {
if (key == null) {
throw new NullPointerException("Null key");
}
this.key = key;
return this;
}
@Override
CelMacro.Builder setVariadic(boolean variadic) {
this.variadic = variadic;
set$0 |= (byte) 4;
return this;
}
@Override
CelMacro.Builder setExpander(CelMacroExpander expander) {
if (expander == null) {
throw new NullPointerException("Null expander");
}
this.expander = expander;
return this;
}
@Override
CelMacro build() {
if (set$0 != 7
|| this.function == null
|| this.key == null
|| this.expander == null) {
StringBuilder missing = new StringBuilder();
if (this.function == null) {
missing.append(" function");
}
if ((set$0 & 1) == 0) {
missing.append(" argumentCount");
}
if ((set$0 & 2) == 0) {
missing.append(" receiverStyle");
}
if (this.key == null) {
missing.append(" key");
}
if ((set$0 & 4) == 0) {
missing.append(" variadic");
}
if (this.expander == null) {
missing.append(" expander");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CelMacro(
this.function,
this.argumentCount,
this.receiverStyle,
this.key,
this.variadic,
this.expander);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy