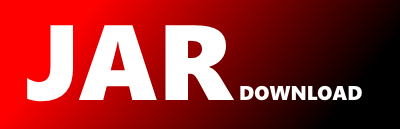
dev.crashteam.crm.CrmServiceGrpc Maven / Gradle / Ivy
package dev.crashteam.crm;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.56.1)",
comments = "Source: crm.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class CrmServiceGrpc {
private CrmServiceGrpc() {}
public static final String SERVICE_NAME = "crm.CrmService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getCreateLeadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "createLead",
requestType = dev.crashteam.crm.CreateLeadRequest.class,
responseType = dev.crashteam.crm.CreateLeadResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateLeadMethod() {
io.grpc.MethodDescriptor getCreateLeadMethod;
if ((getCreateLeadMethod = CrmServiceGrpc.getCreateLeadMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getCreateLeadMethod = CrmServiceGrpc.getCreateLeadMethod) == null) {
CrmServiceGrpc.getCreateLeadMethod = getCreateLeadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "createLead"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.CreateLeadRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.CreateLeadResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("createLead"))
.build();
}
}
}
return getCreateLeadMethod;
}
private static volatile io.grpc.MethodDescriptor getGetUserContactInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "getUserContactInfo",
requestType = dev.crashteam.crm.GetUserContactInfoRequest.class,
responseType = dev.crashteam.crm.GetUserContactInfoResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetUserContactInfoMethod() {
io.grpc.MethodDescriptor getGetUserContactInfoMethod;
if ((getGetUserContactInfoMethod = CrmServiceGrpc.getGetUserContactInfoMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getGetUserContactInfoMethod = CrmServiceGrpc.getGetUserContactInfoMethod) == null) {
CrmServiceGrpc.getGetUserContactInfoMethod = getGetUserContactInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "getUserContactInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.GetUserContactInfoRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.GetUserContactInfoResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("getUserContactInfo"))
.build();
}
}
}
return getGetUserContactInfoMethod;
}
private static volatile io.grpc.MethodDescriptor getUpdateUserContactInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "updateUserContactInfo",
requestType = dev.crashteam.crm.UpdateUserContactInfoRequest.class,
responseType = dev.crashteam.crm.UpdateUserContactInfoResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpdateUserContactInfoMethod() {
io.grpc.MethodDescriptor getUpdateUserContactInfoMethod;
if ((getUpdateUserContactInfoMethod = CrmServiceGrpc.getUpdateUserContactInfoMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getUpdateUserContactInfoMethod = CrmServiceGrpc.getUpdateUserContactInfoMethod) == null) {
CrmServiceGrpc.getUpdateUserContactInfoMethod = getUpdateUserContactInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "updateUserContactInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.UpdateUserContactInfoRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.UpdateUserContactInfoResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("updateUserContactInfo"))
.build();
}
}
}
return getUpdateUserContactInfoMethod;
}
private static volatile io.grpc.MethodDescriptor getSendUserMessageMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "sendUserMessage",
requestType = dev.crashteam.crm.SendUserMessageRequest.class,
responseType = dev.crashteam.crm.SendUserMessageResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSendUserMessageMethod() {
io.grpc.MethodDescriptor getSendUserMessageMethod;
if ((getSendUserMessageMethod = CrmServiceGrpc.getSendUserMessageMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getSendUserMessageMethod = CrmServiceGrpc.getSendUserMessageMethod) == null) {
CrmServiceGrpc.getSendUserMessageMethod = getSendUserMessageMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "sendUserMessage"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.SendUserMessageRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.SendUserMessageResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("sendUserMessage"))
.build();
}
}
}
return getSendUserMessageMethod;
}
private static volatile io.grpc.MethodDescriptor getSendUserAuthCodeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "sendUserAuthCode",
requestType = dev.crashteam.crm.SendUserAuthCodeRequest.class,
responseType = dev.crashteam.crm.SendUserAuthCodeResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSendUserAuthCodeMethod() {
io.grpc.MethodDescriptor getSendUserAuthCodeMethod;
if ((getSendUserAuthCodeMethod = CrmServiceGrpc.getSendUserAuthCodeMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getSendUserAuthCodeMethod = CrmServiceGrpc.getSendUserAuthCodeMethod) == null) {
CrmServiceGrpc.getSendUserAuthCodeMethod = getSendUserAuthCodeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "sendUserAuthCode"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.SendUserAuthCodeRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.SendUserAuthCodeResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("sendUserAuthCode"))
.build();
}
}
}
return getSendUserAuthCodeMethod;
}
private static volatile io.grpc.MethodDescriptor getCheckUserAuthCodeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "checkUserAuthCode",
requestType = dev.crashteam.crm.CheckUserAuthCodeRequest.class,
responseType = dev.crashteam.crm.CheckUserAuthCodeResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCheckUserAuthCodeMethod() {
io.grpc.MethodDescriptor getCheckUserAuthCodeMethod;
if ((getCheckUserAuthCodeMethod = CrmServiceGrpc.getCheckUserAuthCodeMethod) == null) {
synchronized (CrmServiceGrpc.class) {
if ((getCheckUserAuthCodeMethod = CrmServiceGrpc.getCheckUserAuthCodeMethod) == null) {
CrmServiceGrpc.getCheckUserAuthCodeMethod = getCheckUserAuthCodeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "checkUserAuthCode"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.CheckUserAuthCodeRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
dev.crashteam.crm.CheckUserAuthCodeResponse.getDefaultInstance()))
.setSchemaDescriptor(new CrmServiceMethodDescriptorSupplier("checkUserAuthCode"))
.build();
}
}
}
return getCheckUserAuthCodeMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static CrmServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CrmServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceStub(channel, callOptions);
}
};
return CrmServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static CrmServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CrmServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceBlockingStub(channel, callOptions);
}
};
return CrmServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static CrmServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CrmServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceFutureStub(channel, callOptions);
}
};
return CrmServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void createLead(dev.crashteam.crm.CreateLeadRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateLeadMethod(), responseObserver);
}
/**
*/
default void getUserContactInfo(dev.crashteam.crm.GetUserContactInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetUserContactInfoMethod(), responseObserver);
}
/**
*/
default void updateUserContactInfo(dev.crashteam.crm.UpdateUserContactInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpdateUserContactInfoMethod(), responseObserver);
}
/**
*/
default void sendUserMessage(dev.crashteam.crm.SendUserMessageRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSendUserMessageMethod(), responseObserver);
}
/**
*/
default void sendUserAuthCode(dev.crashteam.crm.SendUserAuthCodeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSendUserAuthCodeMethod(), responseObserver);
}
/**
*/
default void checkUserAuthCode(dev.crashteam.crm.CheckUserAuthCodeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCheckUserAuthCodeMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service CrmService.
*/
public static abstract class CrmServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return CrmServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service CrmService.
*/
public static final class CrmServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private CrmServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CrmServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceStub(channel, callOptions);
}
/**
*/
public void createLead(dev.crashteam.crm.CreateLeadRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateLeadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getUserContactInfo(dev.crashteam.crm.GetUserContactInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetUserContactInfoMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void updateUserContactInfo(dev.crashteam.crm.UpdateUserContactInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpdateUserContactInfoMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void sendUserMessage(dev.crashteam.crm.SendUserMessageRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSendUserMessageMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void sendUserAuthCode(dev.crashteam.crm.SendUserAuthCodeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSendUserAuthCodeMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void checkUserAuthCode(dev.crashteam.crm.CheckUserAuthCodeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCheckUserAuthCodeMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service CrmService.
*/
public static final class CrmServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private CrmServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CrmServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceBlockingStub(channel, callOptions);
}
/**
*/
public dev.crashteam.crm.CreateLeadResponse createLead(dev.crashteam.crm.CreateLeadRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateLeadMethod(), getCallOptions(), request);
}
/**
*/
public dev.crashteam.crm.GetUserContactInfoResponse getUserContactInfo(dev.crashteam.crm.GetUserContactInfoRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetUserContactInfoMethod(), getCallOptions(), request);
}
/**
*/
public dev.crashteam.crm.UpdateUserContactInfoResponse updateUserContactInfo(dev.crashteam.crm.UpdateUserContactInfoRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpdateUserContactInfoMethod(), getCallOptions(), request);
}
/**
*/
public dev.crashteam.crm.SendUserMessageResponse sendUserMessage(dev.crashteam.crm.SendUserMessageRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSendUserMessageMethod(), getCallOptions(), request);
}
/**
*/
public dev.crashteam.crm.SendUserAuthCodeResponse sendUserAuthCode(dev.crashteam.crm.SendUserAuthCodeRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSendUserAuthCodeMethod(), getCallOptions(), request);
}
/**
*/
public dev.crashteam.crm.CheckUserAuthCodeResponse checkUserAuthCode(dev.crashteam.crm.CheckUserAuthCodeRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCheckUserAuthCodeMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service CrmService.
*/
public static final class CrmServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private CrmServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CrmServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CrmServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture createLead(
dev.crashteam.crm.CreateLeadRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateLeadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getUserContactInfo(
dev.crashteam.crm.GetUserContactInfoRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetUserContactInfoMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture updateUserContactInfo(
dev.crashteam.crm.UpdateUserContactInfoRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpdateUserContactInfoMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture sendUserMessage(
dev.crashteam.crm.SendUserMessageRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSendUserMessageMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture sendUserAuthCode(
dev.crashteam.crm.SendUserAuthCodeRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSendUserAuthCodeMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture checkUserAuthCode(
dev.crashteam.crm.CheckUserAuthCodeRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCheckUserAuthCodeMethod(), getCallOptions()), request);
}
}
private static final int METHODID_CREATE_LEAD = 0;
private static final int METHODID_GET_USER_CONTACT_INFO = 1;
private static final int METHODID_UPDATE_USER_CONTACT_INFO = 2;
private static final int METHODID_SEND_USER_MESSAGE = 3;
private static final int METHODID_SEND_USER_AUTH_CODE = 4;
private static final int METHODID_CHECK_USER_AUTH_CODE = 5;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_CREATE_LEAD:
serviceImpl.createLead((dev.crashteam.crm.CreateLeadRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_USER_CONTACT_INFO:
serviceImpl.getUserContactInfo((dev.crashteam.crm.GetUserContactInfoRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE_USER_CONTACT_INFO:
serviceImpl.updateUserContactInfo((dev.crashteam.crm.UpdateUserContactInfoRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SEND_USER_MESSAGE:
serviceImpl.sendUserMessage((dev.crashteam.crm.SendUserMessageRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SEND_USER_AUTH_CODE:
serviceImpl.sendUserAuthCode((dev.crashteam.crm.SendUserAuthCodeRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CHECK_USER_AUTH_CODE:
serviceImpl.checkUserAuthCode((dev.crashteam.crm.CheckUserAuthCodeRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getCreateLeadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.CreateLeadRequest,
dev.crashteam.crm.CreateLeadResponse>(
service, METHODID_CREATE_LEAD)))
.addMethod(
getGetUserContactInfoMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.GetUserContactInfoRequest,
dev.crashteam.crm.GetUserContactInfoResponse>(
service, METHODID_GET_USER_CONTACT_INFO)))
.addMethod(
getUpdateUserContactInfoMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.UpdateUserContactInfoRequest,
dev.crashteam.crm.UpdateUserContactInfoResponse>(
service, METHODID_UPDATE_USER_CONTACT_INFO)))
.addMethod(
getSendUserMessageMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.SendUserMessageRequest,
dev.crashteam.crm.SendUserMessageResponse>(
service, METHODID_SEND_USER_MESSAGE)))
.addMethod(
getSendUserAuthCodeMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.SendUserAuthCodeRequest,
dev.crashteam.crm.SendUserAuthCodeResponse>(
service, METHODID_SEND_USER_AUTH_CODE)))
.addMethod(
getCheckUserAuthCodeMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
dev.crashteam.crm.CheckUserAuthCodeRequest,
dev.crashteam.crm.CheckUserAuthCodeResponse>(
service, METHODID_CHECK_USER_AUTH_CODE)))
.build();
}
private static abstract class CrmServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
CrmServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return dev.crashteam.crm.CrmProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("CrmService");
}
}
private static final class CrmServiceFileDescriptorSupplier
extends CrmServiceBaseDescriptorSupplier {
CrmServiceFileDescriptorSupplier() {}
}
private static final class CrmServiceMethodDescriptorSupplier
extends CrmServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
CrmServiceMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (CrmServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new CrmServiceFileDescriptorSupplier())
.addMethod(getCreateLeadMethod())
.addMethod(getGetUserContactInfoMethod())
.addMethod(getUpdateUserContactInfoMethod())
.addMethod(getSendUserMessageMethod())
.addMethod(getSendUserAuthCodeMethod())
.addMethod(getCheckUserAuthCodeMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy