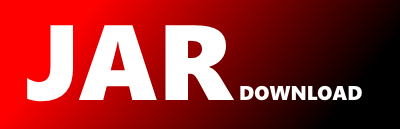
dev.crashteam.openapi.uzumanalytics.api.ReportApi Maven / Gradle / Ivy
Show all versions of openapi-uzum-analytics Show documentation
package dev.crashteam.openapi.uzumanalytics.api;
import dev.crashteam.openapi.uzumanalytics.ApiClient;
import dev.crashteam.openapi.uzumanalytics.model.Error;
import java.io.File;
import dev.crashteam.openapi.uzumanalytics.model.GetReportBySeller200Response;
import dev.crashteam.openapi.uzumanalytics.model.GetReportStateByJobId200Response;
import dev.crashteam.openapi.uzumanalytics.model.GetSellerShops400Response;
import java.time.OffsetDateTime;
import dev.crashteam.openapi.uzumanalytics.model.Report;
import java.util.UUID;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-02-21T16:53:18.489277710Z[Etc/UTC]")
public class ReportApi {
private ApiClient apiClient;
public ReportApi() {
this(new ApiClient());
}
public ReportApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Сгенерировать отчет по категории
*
* 200 - Идентификатор созданной job
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param categoryId (required)
* @param period (required)
* @param idempotenceKey (required)
* @return GetReportBySeller200Response
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public GetReportBySeller200Response getReportByCategory(Long categoryId, Integer period, String idempotenceKey) throws RestClientException {
return getReportByCategoryWithHttpInfo(categoryId, period, idempotenceKey).getBody();
}
/**
* Сгенерировать отчет по категории
*
*
200 - Идентификатор созданной job
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param categoryId (required)
* @param period (required)
* @param idempotenceKey (required)
* @return ResponseEntity<GetReportBySeller200Response>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity getReportByCategoryWithHttpInfo(Long categoryId, Integer period, String idempotenceKey) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'categoryId' is set
if (categoryId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'categoryId' when calling getReportByCategory");
}
// verify the required parameter 'period' is set
if (period == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'period' when calling getReportByCategory");
}
// verify the required parameter 'idempotenceKey' is set
if (idempotenceKey == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'idempotenceKey' when calling getReportByCategory");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("categoryId", categoryId);
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "period", period));
if (idempotenceKey != null)
localVarHeaderParams.add("Idempotence-Key", apiClient.parameterToString(idempotenceKey));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference localReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/report/category/{categoryId}", HttpMethod.GET, uriVariables, localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
/**
* Скачать файл по идентификатору отчета
*
* 200 - Файл отчета
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param reportId (required)
* @return File
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public File getReportByReportId(String reportId) throws RestClientException {
return getReportByReportIdWithHttpInfo(reportId).getBody();
}
/**
* Скачать файл по идентификатору отчета
*
*
200 - Файл отчета
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param reportId (required)
* @return ResponseEntity<File>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity getReportByReportIdWithHttpInfo(String reportId) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'reportId' is set
if (reportId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'reportId' when calling getReportByReportId");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("reportId", reportId);
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
final String[] localVarAccepts = {
"application/xlsx", "application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference localReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/report/{reportId}", HttpMethod.GET, uriVariables, localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
/**
* Сгенерировать отчет по магазину
*
* 200 - Идентификатор созданной job
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param sellerLink (required)
* @param period (required)
* @param idempotenceKey (required)
* @return GetReportBySeller200Response
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public GetReportBySeller200Response getReportBySeller(String sellerLink, Integer period, String idempotenceKey) throws RestClientException {
return getReportBySellerWithHttpInfo(sellerLink, period, idempotenceKey).getBody();
}
/**
* Сгенерировать отчет по магазину
*
*
200 - Идентификатор созданной job
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param sellerLink (required)
* @param period (required)
* @param idempotenceKey (required)
* @return ResponseEntity<GetReportBySeller200Response>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity getReportBySellerWithHttpInfo(String sellerLink, Integer period, String idempotenceKey) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'sellerLink' is set
if (sellerLink == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'sellerLink' when calling getReportBySeller");
}
// verify the required parameter 'period' is set
if (period == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'period' when calling getReportBySeller");
}
// verify the required parameter 'idempotenceKey' is set
if (idempotenceKey == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'idempotenceKey' when calling getReportBySeller");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("sellerLink", sellerLink);
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "period", period));
if (idempotenceKey != null)
localVarHeaderParams.add("Idempotence-Key", apiClient.parameterToString(idempotenceKey));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference localReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/report/seller/{sellerLink}", HttpMethod.GET, uriVariables, localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
/**
* Статус генерации отчета
*
* 200 - Статус отчета
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param jobId (required)
* @return GetReportStateByJobId200Response
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public GetReportStateByJobId200Response getReportStateByJobId(UUID jobId) throws RestClientException {
return getReportStateByJobIdWithHttpInfo(jobId).getBody();
}
/**
* Статус генерации отчета
*
*
200 - Статус отчета
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param jobId (required)
* @return ResponseEntity<GetReportStateByJobId200Response>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity getReportStateByJobIdWithHttpInfo(UUID jobId) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'jobId' when calling getReportStateByJobId");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("jobId", jobId);
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference localReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/report/state/{jobId}", HttpMethod.GET, uriVariables, localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
/**
* Получить список всех отчетов
*
* 200 - Список отчетов пользователя
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param fromTime Start date time. (required)
* @return List<Report>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getReports(OffsetDateTime fromTime) throws RestClientException {
return getReportsWithHttpInfo(fromTime).getBody();
}
/**
* Получить список всех отчетов
*
* 200 - Список отчетов пользователя
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param fromTime Start date time. (required)
* @return ResponseEntity<List<Report>>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity> getReportsWithHttpInfo(OffsetDateTime fromTime) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling getReports");
}
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference> localReturnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI("/reports", HttpMethod.GET, Collections.emptyMap(), localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
}