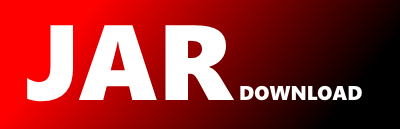
dev.crashteam.openapi.uzumanalytics.api.SellerApi Maven / Gradle / Ivy
Show all versions of openapi-uzum-analytics Show documentation
package dev.crashteam.openapi.uzumanalytics.api;
import dev.crashteam.openapi.uzumanalytics.ApiClient;
import dev.crashteam.openapi.uzumanalytics.model.Error;
import dev.crashteam.openapi.uzumanalytics.model.GetSellerShops400Response;
import java.time.OffsetDateTime;
import dev.crashteam.openapi.uzumanalytics.model.Seller;
import dev.crashteam.openapi.uzumanalytics.model.SellerOverallInfo200Response;
import java.util.UUID;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-02-21T16:53:18.489277710Z[Etc/UTC]")
public class SellerApi {
private ApiClient apiClient;
public SellerApi() {
this(new ApiClient());
}
public SellerApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Получить список магазинов продавца
*
* 200 - Список магазинов продавца
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param sellerLink (required)
* @return List<Seller>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getSellerShops(String sellerLink) throws RestClientException {
return getSellerShopsWithHttpInfo(sellerLink).getBody();
}
/**
* Получить список магазинов продавца
*
* 200 - Список магазинов продавца
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
* @param sellerLink (required)
* @return ResponseEntity<List<Seller>>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity> getSellerShopsWithHttpInfo(String sellerLink) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'sellerLink' is set
if (sellerLink == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'sellerLink' when calling getSellerShops");
}
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "sellerLink", sellerLink));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference> localReturnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI("/seller/link", HttpMethod.GET, Collections.emptyMap(), localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
/**
* Общая информация по магазину
*
* 200 - Получены общие аналитические данные по магазину
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
*
404 - Данные по магазину не найдены
* @param xRequestID Уникальный идентификатор запроса к системе (required)
* @param X_API_KEY Уникальный токен пользователя расширения (required)
* @param sellerLink (required)
* @param fromTime Start date time. (required)
* @param toTime End date time. (required)
* @return SellerOverallInfo200Response
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public SellerOverallInfo200Response sellerOverallInfo(UUID xRequestID, String X_API_KEY, String sellerLink, OffsetDateTime fromTime, OffsetDateTime toTime) throws RestClientException {
return sellerOverallInfoWithHttpInfo(xRequestID, X_API_KEY, sellerLink, fromTime, toTime).getBody();
}
/**
* Общая информация по магазину
*
*
200 - Получены общие аналитические данные по магазину
*
400 - Переданы ошибочные данные
*
401 - Несанкционированный доступ, использовались неверные учетные данные.
*
403 - Access forbidden.
*
404 - Данные по магазину не найдены
* @param xRequestID Уникальный идентификатор запроса к системе (required)
* @param X_API_KEY Уникальный токен пользователя расширения (required)
* @param sellerLink (required)
* @param fromTime Start date time. (required)
* @param toTime End date time. (required)
* @return ResponseEntity<SellerOverallInfo200Response>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public ResponseEntity sellerOverallInfoWithHttpInfo(UUID xRequestID, String X_API_KEY, String sellerLink, OffsetDateTime fromTime, OffsetDateTime toTime) throws RestClientException {
Object localVarPostBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling sellerOverallInfo");
}
// verify the required parameter 'X_API_KEY' is set
if (X_API_KEY == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'X_API_KEY' when calling sellerOverallInfo");
}
// verify the required parameter 'sellerLink' is set
if (sellerLink == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'sellerLink' when calling sellerOverallInfo");
}
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling sellerOverallInfo");
}
// verify the required parameter 'toTime' is set
if (toTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'toTime' when calling sellerOverallInfo");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("sellerLink", sellerLink);
final MultiValueMap localVarQueryParams = new LinkedMultiValueMap();
final HttpHeaders localVarHeaderParams = new HttpHeaders();
final MultiValueMap localVarCookieParams = new LinkedMultiValueMap();
final MultiValueMap localVarFormParams = new LinkedMultiValueMap();
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
localVarQueryParams.putAll(apiClient.parameterToMultiValueMap(null, "toTime", toTime));
if (xRequestID != null)
localVarHeaderParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (X_API_KEY != null)
localVarHeaderParams.add("X-API-KEY", apiClient.parameterToString(X_API_KEY));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "apiKey" };
ParameterizedTypeReference localReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/seller/{sellerLink}/overall-info", HttpMethod.GET, uriVariables, localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localReturnType);
}
}