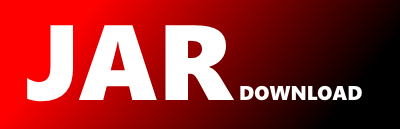
dev.crashteam.openapi.uzumanalytics.model.SellerOverallInfo200Response Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-uzum-analytics Show documentation
Show all versions of openapi-uzum-analytics Show documentation
Generates jar artifact containing compiled openapi classes based on generated openapi yaml files
/*
* CrashTeam Uzum Analytics API
* CrashTeam Dev Uzum Analytics API
*
* The version of the OpenAPI document: 0.0.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package dev.crashteam.openapi.uzumanalytics.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import dev.crashteam.openapi.uzumanalytics.model.DynamicSales;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* SellerOverallInfo200Response
*/
@JsonPropertyOrder({
SellerOverallInfo200Response.JSON_PROPERTY_AVERAGE_PRICE,
SellerOverallInfo200Response.JSON_PROPERTY_REVENUE,
SellerOverallInfo200Response.JSON_PROPERTY_ORDER_COUNT,
SellerOverallInfo200Response.JSON_PROPERTY_PRODUCT_COUNT,
SellerOverallInfo200Response.JSON_PROPERTY_PRODUCT_COUNT_WITH_SALES,
SellerOverallInfo200Response.JSON_PROPERTY_PRODUCT_COUNT_WITHOUT_SALES,
SellerOverallInfo200Response.JSON_PROPERTY_SALES_DYNAMIC
})
@JsonTypeName("sellerOverallInfo_200_response")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-02-21T16:53:18.489277710Z[Etc/UTC]")
public class SellerOverallInfo200Response {
public static final String JSON_PROPERTY_AVERAGE_PRICE = "averagePrice";
private Double averagePrice;
public static final String JSON_PROPERTY_REVENUE = "revenue";
private Double revenue;
public static final String JSON_PROPERTY_ORDER_COUNT = "orderCount";
private Long orderCount;
public static final String JSON_PROPERTY_PRODUCT_COUNT = "productCount";
private Long productCount;
public static final String JSON_PROPERTY_PRODUCT_COUNT_WITH_SALES = "productCountWithSales";
private Long productCountWithSales;
public static final String JSON_PROPERTY_PRODUCT_COUNT_WITHOUT_SALES = "productCountWithoutSales";
private Long productCountWithoutSales;
public static final String JSON_PROPERTY_SALES_DYNAMIC = "salesDynamic";
private List salesDynamic;
public SellerOverallInfo200Response() {
}
public SellerOverallInfo200Response averagePrice(Double averagePrice) {
this.averagePrice = averagePrice;
return this;
}
/**
* Средняя цена по магазину
* @return averagePrice
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AVERAGE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getAveragePrice() {
return averagePrice;
}
@JsonProperty(JSON_PROPERTY_AVERAGE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAveragePrice(Double averagePrice) {
this.averagePrice = averagePrice;
}
public SellerOverallInfo200Response revenue(Double revenue) {
this.revenue = revenue;
return this;
}
/**
* Общая выручка по магазину
* @return revenue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_REVENUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getRevenue() {
return revenue;
}
@JsonProperty(JSON_PROPERTY_REVENUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRevenue(Double revenue) {
this.revenue = revenue;
}
public SellerOverallInfo200Response orderCount(Long orderCount) {
this.orderCount = orderCount;
return this;
}
/**
* Кол-во заказов
* @return orderCount
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ORDER_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getOrderCount() {
return orderCount;
}
@JsonProperty(JSON_PROPERTY_ORDER_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOrderCount(Long orderCount) {
this.orderCount = orderCount;
}
public SellerOverallInfo200Response productCount(Long productCount) {
this.productCount = productCount;
return this;
}
/**
* Кол-во товаров (карточек)
* @return productCount
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getProductCount() {
return productCount;
}
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProductCount(Long productCount) {
this.productCount = productCount;
}
public SellerOverallInfo200Response productCountWithSales(Long productCountWithSales) {
this.productCountWithSales = productCountWithSales;
return this;
}
/**
* Кол-во товаров (карточек) с продажами
* @return productCountWithSales
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT_WITH_SALES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getProductCountWithSales() {
return productCountWithSales;
}
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT_WITH_SALES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProductCountWithSales(Long productCountWithSales) {
this.productCountWithSales = productCountWithSales;
}
public SellerOverallInfo200Response productCountWithoutSales(Long productCountWithoutSales) {
this.productCountWithoutSales = productCountWithoutSales;
return this;
}
/**
* Кол-во товаров (карточек) без продаж
* @return productCountWithoutSales
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT_WITHOUT_SALES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getProductCountWithoutSales() {
return productCountWithoutSales;
}
@JsonProperty(JSON_PROPERTY_PRODUCT_COUNT_WITHOUT_SALES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProductCountWithoutSales(Long productCountWithoutSales) {
this.productCountWithoutSales = productCountWithoutSales;
}
public SellerOverallInfo200Response salesDynamic(List salesDynamic) {
this.salesDynamic = salesDynamic;
return this;
}
public SellerOverallInfo200Response addSalesDynamicItem(DynamicSales salesDynamicItem) {
if (this.salesDynamic == null) {
this.salesDynamic = new ArrayList<>();
}
this.salesDynamic.add(salesDynamicItem);
return this;
}
/**
* Get salesDynamic
* @return salesDynamic
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SALES_DYNAMIC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSalesDynamic() {
return salesDynamic;
}
@JsonProperty(JSON_PROPERTY_SALES_DYNAMIC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSalesDynamic(List salesDynamic) {
this.salesDynamic = salesDynamic;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SellerOverallInfo200Response sellerOverallInfo200Response = (SellerOverallInfo200Response) o;
return Objects.equals(this.averagePrice, sellerOverallInfo200Response.averagePrice) &&
Objects.equals(this.revenue, sellerOverallInfo200Response.revenue) &&
Objects.equals(this.orderCount, sellerOverallInfo200Response.orderCount) &&
Objects.equals(this.productCount, sellerOverallInfo200Response.productCount) &&
Objects.equals(this.productCountWithSales, sellerOverallInfo200Response.productCountWithSales) &&
Objects.equals(this.productCountWithoutSales, sellerOverallInfo200Response.productCountWithoutSales) &&
Objects.equals(this.salesDynamic, sellerOverallInfo200Response.salesDynamic);
}
@Override
public int hashCode() {
return Objects.hash(averagePrice, revenue, orderCount, productCount, productCountWithSales, productCountWithoutSales, salesDynamic);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SellerOverallInfo200Response {\n");
sb.append(" averagePrice: ").append(toIndentedString(averagePrice)).append("\n");
sb.append(" revenue: ").append(toIndentedString(revenue)).append("\n");
sb.append(" orderCount: ").append(toIndentedString(orderCount)).append("\n");
sb.append(" productCount: ").append(toIndentedString(productCount)).append("\n");
sb.append(" productCountWithSales: ").append(toIndentedString(productCountWithSales)).append("\n");
sb.append(" productCountWithoutSales: ").append(toIndentedString(productCountWithoutSales)).append("\n");
sb.append(" salesDynamic: ").append(toIndentedString(salesDynamic)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy