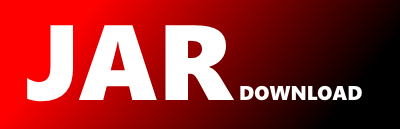
dev.dsf.fhir.authorization.AuthorizationRule Maven / Gradle / Ivy
package dev.dsf.fhir.authorization;
import java.sql.Connection;
import java.util.Optional;
import org.hl7.fhir.r4.model.Resource;
import dev.dsf.common.auth.conf.Identity;
public interface AuthorizationRule
{
Class getResourceType();
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @param newResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if create allowed
*/
Optional reasonCreateAllowed(Identity identity, R newResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param connection
* not null
* @param identity
* not null
* @param newResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if create allowed
*/
Optional reasonCreateAllowed(Connection connection, Identity identity, R newResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @param existingResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if read allowed
*/
Optional reasonReadAllowed(Identity identity, R existingResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param connection
* not null
* @param identity
* not null
* @param existingResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if read allowed
*/
Optional reasonReadAllowed(Connection connection, Identity identity, R existingResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @param oldResource
* not null
* @param newResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if update allowed
*/
Optional reasonUpdateAllowed(Identity identity, R oldResource, R newResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param connection
* not null
* @param identity
* not null
* @param oldResource
* not null
* @param newResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if update allowed
*/
Optional reasonUpdateAllowed(Connection connection, Identity identity, R oldResource, R newResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @param oldResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if delete allowed
*/
Optional reasonDeleteAllowed(Identity identity, R oldResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param connection
* not null
* @param identity
* not null
* @param oldResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if delete allowed
*/
Optional reasonDeleteAllowed(Connection connection, Identity identity, R oldResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @return Reason as String in {@link Optional#of(Object)} if delete allowed
*/
Optional reasonSearchAllowed(Identity identity);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @return Reason as String in {@link Optional#of(Object)} if delete allowed
*/
Optional reasonHistoryAllowed(Identity identity);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param identity
* not null
* @param oldResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if permanent delete allowed
*/
Optional reasonPermanentDeleteAllowed(Identity identity, R oldResource);
/**
* Override this method for non default behavior. Default: Not allowed.
*
* @param connection
* not null
* @param identity
* not null
* @param oldResource
* not null
* @return Reason as String in {@link Optional#of(Object)} if permanent delete allowed
*/
Optional reasonPermanentDeleteAllowed(Connection connection, Identity identity, R oldResource);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy