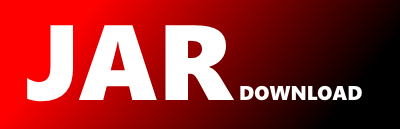
dev.dsf.fhir.webservice.specification.BasicResourceService Maven / Gradle / Ivy
package dev.dsf.fhir.webservice.specification;
import org.hl7.fhir.r4.model.Resource;
import dev.dsf.fhir.webservice.base.BasicService;
import jakarta.ws.rs.core.HttpHeaders;
import jakarta.ws.rs.core.Response;
import jakarta.ws.rs.core.UriInfo;
public interface BasicResourceService extends BasicService
{
/**
* standard and conditional create
*
* @param resource
* not null
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#create
*/
Response create(R resource, UriInfo uri, HttpHeaders headers);
/**
* read by id
*
* @param id
* not null
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#read
*/
Response read(String id, UriInfo uri, HttpHeaders headers);
/**
* read by id and version
*
* @param id
* not null
* @param version
* {@code >0}
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#vread
*/
Response vread(String id, long version, UriInfo uri, HttpHeaders headers);
Response history(UriInfo uri, HttpHeaders headers);
Response history(String id, UriInfo uri, HttpHeaders headers);
/**
* standard update
*
* @param id
* not null
* @param resource
* not null
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#update
*/
Response update(String id, R resource, UriInfo uri, HttpHeaders headers);
/**
* conditional update
*
* @param resource
* not null
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#update
*/
Response update(R resource, UriInfo uri, HttpHeaders headers);
/**
* standard delete
*
* @param id
* not null
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#delete
*/
Response delete(String id, UriInfo uri, HttpHeaders headers);
/**
* conditional delete
*
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#delete
*/
Response delete(UriInfo uri, HttpHeaders headers);
/**
* search by request parameter
*
* @param uri
* not null
* @param headers
* not null
* @return {@link Response} defined in
* https://www.hl7.org/fhir/http.html#search
*/
Response search(UriInfo uri, HttpHeaders headers);
Response deletePermanently(String deletePath, String id, UriInfo uri, HttpHeaders headers);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy