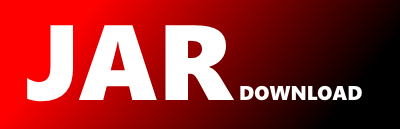
dev.failsafe.AsyncExecutionImpl Maven / Gradle / Ivy
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*/
package dev.failsafe;
import dev.failsafe.internal.util.Assert;
import dev.failsafe.spi.*;
import dev.failsafe.spi.*;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
import java.util.function.Function;
/**
* AsyncExecution and AsyncExecutionInternal implementation.
*
* @param result type
* @author Jonathan Halterman
*/
final class AsyncExecutionImpl extends ExecutionImpl implements AsyncExecutionInternal {
// -- Cross-attempt state --
private final FailsafeFuture future;
private final boolean asyncExecution;
// The outer-most function that executions begin with
private Function, CompletableFuture>> outerFn;
// -- Per-attempt state --
// Whether a policy executor completed post execution
private final boolean[] policyPostExecuted = new boolean[policyExecutors.size()];
// Whether a result has been publicly recorded
private volatile boolean recorded;
AsyncExecutionImpl(List> policies, Scheduler scheduler, FailsafeFuture future, boolean asyncExecution,
Function, CompletableFuture>> innerFn) {
super(policies);
this.future = future;
this.asyncExecution = asyncExecution;
outerFn = asyncExecution ? Functions.toExecutionAware(innerFn) : innerFn;
outerFn = Functions.toAsync(outerFn, scheduler, future);
for (PolicyExecutor policyExecutor : policyExecutors)
outerFn = policyExecutor.applyAsync(outerFn, scheduler, future);
}
/**
* Create an async execution for a new attempt.
*/
private AsyncExecutionImpl(AsyncExecutionImpl execution) {
super(execution);
outerFn = execution.outerFn;
future = execution.future;
asyncExecution = execution.asyncExecution;
}
@Override
public void complete() {
Assert.state(!recorded, "The most recent execution has already been recorded or completed");
recorded = true;
// Guard against race with a timeout expiring
synchronized (future) {
ExecutionResult result = this.result != null ? this.result : ExecutionResult.none();
complete(postExecute(result), null);
}
}
@Override
public boolean isComplete() {
return completed;
}
@Override
public void record(R result, Throwable failure) {
Assert.state(!recorded, "The most recent execution has already been recorded or completed");
recorded = true;
// Guard against race with a timeout expiring
synchronized (future) {
if (!attemptRecorded) {
Assert.state(!completed, "Execution has already been completed");
record(new ExecutionResult<>(result, failure));
}
// Proceed with handling the recorded result
executeAsync();
}
}
@Override
public void recordResult(R result) {
record(result, null);
}
@Override
public void recordFailure(Throwable failure) {
record(null, failure);
}
@Override
public boolean isAsyncExecution() {
return asyncExecution;
}
@Override
public boolean isRecorded() {
return recorded;
}
@Override
public synchronized void setPostExecuted(int policyIndex) {
policyPostExecuted[policyIndex] = true;
}
@Override
public synchronized boolean isPostExecuted(int policyIndex) {
return policyPostExecuted[policyIndex];
}
@Override
public AsyncExecutionInternal copy() {
return new AsyncExecutionImpl<>(this);
}
/**
* Performs an asynchronous execution.
*/
void executeAsync() {
outerFn.apply(this).whenComplete(this::complete);
}
private void complete(ExecutionResult result, Throwable error) {
if (result == null && error == null)
return;
completed = true;
if (!future.isDone()) {
if (result != null)
future.completeResult(result);
else {
if (error instanceof CompletionException)
error = error.getCause();
future.completeResult(ExecutionResult.failure(error));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy