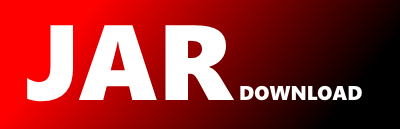
dev.fitko.fitconnect.api.config.Environment Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.config;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import static java.util.Optional.ofNullable;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Environment {
private String authBaseUrl;
private String routingBaseUrl;
private List submissionBaseUrls = Collections.emptyList();
private String selfServicePortalBaseUrl;
private String destinationBaseUrl;
private Boolean enableAutoReject;
private Boolean allowInsecurePublicKey;
private Boolean skipSubmissionDataValidation;
/**
* Merges current env with provided other env.
* If a field of the current env is null or empty, the value from other will be set.
*
* @param other environment to merge the current environment with
* @return merged environment
*/
public Environment merge(final Environment other){
final Environment mergedEnv = new Environment();
mergedEnv.setAuthBaseUrl(ofNullOrEmpty(authBaseUrl).orElse(other.getAuthBaseUrl()));
mergedEnv.setRoutingBaseUrl(ofNullOrEmpty(routingBaseUrl).orElse(other.getRoutingBaseUrl()));
mergedEnv.setSubmissionBaseUrls(ofNullOrEmpty(submissionBaseUrls).orElse(other.getSubmissionBaseUrls()));
mergedEnv.setSelfServicePortalBaseUrl(ofNullOrEmpty(selfServicePortalBaseUrl).orElse(other.getSelfServicePortalBaseUrl()));
mergedEnv.setDestinationBaseUrl(ofNullOrEmpty(destinationBaseUrl).orElse(other.getDestinationBaseUrl()));
mergedEnv.setEnableAutoReject(ofNullable(enableAutoReject).orElse(other.getEnableAutoReject()));
mergedEnv.setAllowInsecurePublicKey(ofNullable(allowInsecurePublicKey).orElse(other.getAllowInsecurePublicKey()));
mergedEnv.setSkipSubmissionDataValidation(ofNullable(skipSubmissionDataValidation).orElse(other.getSkipSubmissionDataValidation()));
return mergedEnv;
}
private static Optional ofNullOrEmpty(final String s){
return s == null || s.isEmpty() ? Optional.empty() : Optional.of(s);
}
private static Optional> ofNullOrEmpty(final List list){
return list == null || list.isEmpty() ? Optional.empty() : Optional.of(list);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy