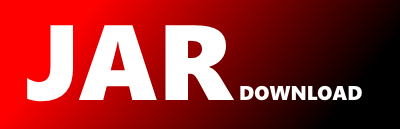
dev.fitko.fitconnect.api.domain.model.destination.DestinationReplyChannels Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.domain.model.destination;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationDeMail;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationElster;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationEmail;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationFink;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationFitConnect;
import dev.fitko.fitconnect.api.domain.model.destination.replychannels.DestinationIdBundDeMailbox;
import dev.fitko.fitconnect.api.domain.model.reply.replychannel.ReplyChannel;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.Collection;
import java.util.Objects;
@Data
@AllArgsConstructor
@NoArgsConstructor
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public class DestinationReplyChannels {
@JsonProperty("eMail")
private DestinationEmail destinationEmail;
@JsonProperty("deMail")
private DestinationDeMail destinationDeMail;
@JsonProperty("fink")
private DestinationFink destinationFink;
@JsonProperty("elster")
private DestinationElster destinationElster;
@JsonProperty("fitConnect")
private DestinationFitConnect destinationFitConnect;
@JsonProperty("idBundDeMailbox")
private DestinationIdBundDeMailbox destinationIdBundDeMailbox;
public static Builder builder() {
return new Builder();
}
public static class Builder {
private DestinationEmail destinationEmail;
private DestinationDeMail destinationDeMail;
private DestinationFink destinationFink;
private DestinationElster destinationElster;
private DestinationFitConnect destinationFitConnect;
private DestinationIdBundDeMailbox destinationIdBundDeMailbox;
Builder() {
}
public Builder withEmail(boolean usePgp) {
this.destinationEmail = new DestinationEmail(usePgp);
return this;
}
public Builder withEmail() {
this.destinationEmail = new DestinationEmail(false);
return this;
}
public Builder withDeMail() {
this.destinationDeMail = new DestinationDeMail();
return this;
}
public Builder withFink() {
this.destinationFink = new DestinationFink();
return this;
}
public Builder withElster() {
this.destinationElster = new DestinationElster();
return this;
}
public Builder withFitConnect(Collection processingStandards) {
this.destinationFitConnect = new DestinationFitConnect(processingStandards);
return this;
}
public Builder withIdBundDeMailbox(boolean useStatusMonitor) {
this.destinationIdBundDeMailbox = new DestinationIdBundDeMailbox(useStatusMonitor);
return this;
}
public Builder withIdBundDeMailbox() {
this.destinationIdBundDeMailbox = new DestinationIdBundDeMailbox(false);
return this;
}
public DestinationReplyChannels build() {
return new DestinationReplyChannels(this.destinationEmail, this.destinationDeMail, this.destinationFink, this.destinationElster, this.destinationFitConnect, this.destinationIdBundDeMailbox);
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DestinationReplyChannels that = (DestinationReplyChannels) o;
return Objects.equals(destinationEmail, that.destinationEmail) &&
Objects.equals(destinationDeMail != null, that.destinationDeMail != null) &&
Objects.equals(destinationFink != null, that.destinationFink != null) &&
Objects.equals(destinationElster != null, that.destinationElster != null) &&
Objects.equals(destinationIdBundDeMailbox != null, that.destinationIdBundDeMailbox != null) &&
Objects.equals(destinationFitConnect, that.destinationFitConnect);
}
public boolean allowsSubmissionReplyChannel(ReplyChannel submissionReplyChannel) {
if (submissionReplyChannel.isDeMail()) {
return destinationDeMail != null;
} else if (submissionReplyChannel.isFink()) {
return destinationFink != null;
} else if (submissionReplyChannel.isElster()) {
return destinationElster != null;
} else if (submissionReplyChannel.isIdBundDeMailbox()) {
return destinationIdBundDeMailbox != null;
} else if (submissionReplyChannel.isEMail()) {
return destinationEmail != null && destinationEmail.getUsePgp().equals(submissionReplyChannel.getEMail().getUsePgp());
} else if (submissionReplyChannel.isFitConnect()) {
return destinationFitConnect != null && destinationFitConnect.getProcessStandards().containsAll(submissionReplyChannel.getFitConnect().getProcessStandards());
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy