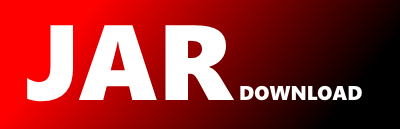
dev.fitko.fitconnect.api.domain.sender.SendableEncryptedSubmission Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.domain.sender;
import dev.fitko.fitconnect.api.domain.model.callback.Callback;
import dev.fitko.fitconnect.api.domain.model.submission.ServiceType;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedBuildStep;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedDataStep;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedDestinationStep;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedMetadataStep;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedOptionalPropertiesStep;
import dev.fitko.fitconnect.api.domain.sender.steps.encrypted.EncryptedServiceTypeStep;
import lombok.Getter;
import java.net.URI;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
@Getter
public final class SendableEncryptedSubmission {
private final UUID destinationId;
private final UUID caseId;
private final Map attachments;
private final String data;
private final String metadata;
private final ServiceType serviceType;
private final String serviceRegion;
private final Callback callback;
private SendableEncryptedSubmission(final Builder builder) {
destinationId = builder.getDestinationId();
caseId = builder.getCaseId();
attachments = Collections.unmodifiableMap(builder.getEncryptedAttachments());
data = builder.getEncryptedData();
metadata = builder.getEncryptedMetadata();
serviceType = builder.getServiceType();
serviceRegion = builder.getServiceRegion();
callback = builder.getCallback();
}
public static EncryptedDestinationStep Builder() {
return new Builder();
}
@Getter
private static final class Builder implements EncryptedDestinationStep,
EncryptedServiceTypeStep,
EncryptedMetadataStep,
EncryptedDataStep,
EncryptedOptionalPropertiesStep,
EncryptedBuildStep {
private UUID destinationId;
private UUID caseId;
private ServiceType serviceType;
private String serviceRegion;
private String encryptedData;
private String encryptedMetadata;
private Callback callback;
private final Map encryptedAttachments = new HashMap<>();
private Builder() {
}
@Override
public EncryptedServiceTypeStep setDestination(final UUID destinationId) {
this.destinationId = destinationId;
return this;
}
@Override
public EncryptedOptionalPropertiesStep addEncryptedAttachments(final Map attachments) {
if (attachments != null) {
encryptedAttachments.putAll(attachments);
}
return this;
}
@Override
public EncryptedOptionalPropertiesStep addEncryptedAttachment(final UUID id, final String content) {
addEncryptedAttachments(Map.of(id, content));
return this;
}
@Override
public EncryptedOptionalPropertiesStep setCallback(final URI callbackUri, final String callbackSecret) {
callback = new Callback(callbackUri, callbackSecret);
return this;
}
@Override
public EncryptedOptionalPropertiesStep setCase(final UUID caseID) {
caseId = caseID;
return this;
}
@Override
public EncryptedOptionalPropertiesStep setEncryptedData(final String data) {
encryptedData = data;
return this;
}
@Override
public EncryptedDataStep setEncryptedMetadata(final String encryptedMetadata) {
this.encryptedMetadata = encryptedMetadata;
return this;
}
@Override
public EncryptedMetadataStep setServiceType(final String serviceIdentifier, final String serviceName) {
this.serviceType = new ServiceType(serviceName, serviceIdentifier);
return this;
}
@Override
public EncryptedMetadataStep setServiceTypeWithRegion(String serviceIdentifier, String serviceName, String serviceRegion) {
this.serviceType = new ServiceType(serviceName, serviceIdentifier);
this.serviceRegion = serviceRegion;
return this;
}
@Override
public SendableEncryptedSubmission build() {
return new SendableEncryptedSubmission(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy