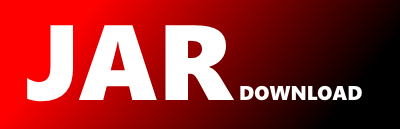
dev.fitko.fitconnect.api.domain.validation.ValidationResult Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.domain.validation;
import dev.fitko.fitconnect.api.domain.model.event.problems.Problem;
import dev.fitko.fitconnect.api.exceptions.internal.ValidationException;
import java.util.ArrayList;
import java.util.List;
/**
* Wrapper for validations including an exception
*/
public final class ValidationResult {
private final boolean isValid;
private final Exception error;
private final List validationProblems = new ArrayList<>();
private ValidationResult(final boolean isValid) {
this.isValid = isValid;
error = null;
}
private ValidationResult(final boolean isValid, final Exception error) {
this.isValid = isValid;
this.error = error;
}
private ValidationResult(final Problem problem) {
isValid = false;
error = null;
validationProblems.add(problem);
}
private ValidationResult(final List problems) {
isValid = false;
error = null;
validationProblems.addAll(problems);
}
private ValidationResult(final Exception error, final Problem problem) {
isValid = false;
this.error = error;
validationProblems.add(problem);
}
/**
* Create new valid result.
*
* @return the valid result
*/
public static ValidationResult ok() {
return new ValidationResult(true);
}
/**
* Create new failed result with an exception.
*
* @return the invalid result
*/
public static ValidationResult error(final Exception exception) {
return new ValidationResult(false, exception);
}
/**
* Create new failed result with an error message that's wrapped in a ValidationException.
*
* @return the invalid result
*/
public static ValidationResult error(final String errorMessage) {
return new ValidationResult(false, new ValidationException(errorMessage));
}
/**
* Create new failed result with a {@link Problem}.
*
* @return the invalid result
*/
public static ValidationResult problem(final Problem problem) {
return new ValidationResult(problem);
}
/**
* Create new failed result with a list of {@link Problem}.
*
* @return the invalid result
*/
public static ValidationResult problems(final List problems) {
return new ValidationResult(problems);
}
/**
* Create new failed result with an Exception and a {@link Problem}.
*
* @return the invalid result
*/
public static ValidationResult withErrorAndProblem(final Exception exception, final Problem problem) {
return new ValidationResult(exception, problem);
}
/**
* Successful validation without errors.
*
* @return true if valid
*/
public boolean isValid() {
return isValid;
}
/**
* Failed validation with an error.
*
* @return true if an error occurred
*/
public boolean hasError() {
return error != null;
}
/**
* Failed validation with a problem error that gets auto-rejected.
*
* @return true if a problem occurred
*/
public boolean hasProblems() {
return !validationProblems.isEmpty();
}
/**
* Gets the problem that was detected during validation.
*
* @return {@link Problem}
*/
public List getProblems() {
return validationProblems;
}
/**
* Gets the exception that occurred during validation.
*
* @return {@link Exception}
*/
public Exception getError() {
return error;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy