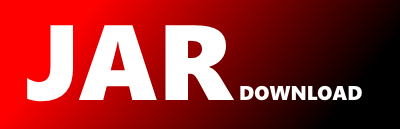
dev.fitko.fitconnect.api.domain.zbp.AuthorKeyPair Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.domain.zbp;
import com.nimbusds.jose.JOSEException;
import com.nimbusds.jose.jwk.JWK;
import com.nimbusds.jose.jwk.RSAKey;
import lombok.Value;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
@Value
public class AuthorKeyPair {
RSAKey authorPrivateKey;
String authorCertificateAsPem;
private AuthorKeyPair(RSAKey authorPrivateKey, String authorCertificateAsPem) {
this.authorPrivateKey = authorPrivateKey;
this.authorCertificateAsPem = authorCertificateAsPem;
}
public static AuthorKeyPairBuilder builder() {
return new AuthorKeyPairBuilder();
}
public static class AuthorKeyPairBuilder {
private String authorCertificateAsPem;
private RSAKey authorPrivateKey;
AuthorKeyPairBuilder() {
}
/**
* Set authors client certificate as {@link Path}.
*
* @param authorCertificatePath path to the public certificate in PEM format
* @return AuthorKeyPairBuilder
*/
public AuthorKeyPairBuilder authorCertificatePath(Path authorCertificatePath) {
this.authorCertificateAsPem = getPemFromPath(authorCertificatePath);
return this;
}
/**
* Set authors client certificate as PEM string.
*
* @param authorCertificateAsPem PEM string of the public certificate
* @return AuthorKeyPairBuilder
*/
public AuthorKeyPairBuilder authorCertificateAsPem(String authorCertificateAsPem) {
getKeyFromPemString(authorCertificateAsPem);
this.authorCertificateAsPem = authorCertificateAsPem;
return this;
}
/**
* Set authors private key as {@link Path}.
*
* @param authorPrivateKeyPath path to the private key in PEM format
* @return AuthorKeyPairBuilder
*/
public AuthorKeyPairBuilder authorPrivateKeyPath(Path authorPrivateKeyPath) {
this.authorPrivateKey = getKeyFromPath(authorPrivateKeyPath);
return this;
}
/**
* Set authors private key as PEM string.
*
* @param authorPrivateKeyAsPem PEM string of the private key
* @return AuthorKeyPairBuilder
*/
public AuthorKeyPairBuilder authorPrivateKeyAsPem(String authorPrivateKeyAsPem) {
this.authorPrivateKey = getKeyFromPemString(authorPrivateKeyAsPem);
return this;
}
/**
* Set authors private key.
*
* @param authorPrivateKey private key as {@link RSAKey}
* @return AuthorKeyPairBuilder
*/
public AuthorKeyPairBuilder authorPrivateKey(RSAKey authorPrivateKey) {
this.authorPrivateKey = authorPrivateKey;
return this;
}
public AuthorKeyPair build() {
return new AuthorKeyPair(this.authorPrivateKey, this.authorCertificateAsPem);
}
private RSAKey getKeyFromPath(Path path) {
try {
final String pemObject = Files.readString(path);
return getKeyFromPemString(pemObject);
} catch (IOException e) {
throw new RuntimeException("Failed reading path to PEM", e);
}
}
private String getPemFromPath(Path path) {
try {
final String pemObject = Files.readString(path);
// read as key for validation
getKeyFromPemString(pemObject);
return pemObject;
} catch (IOException e) {
throw new RuntimeException("Failed reading PEM", e);
}
}
private RSAKey getKeyFromPemString(String pemObject) {
try {
return JWK.parseFromPEMEncodedObjects(pemObject).toRSAKey();
} catch (JOSEException e) {
throw new RuntimeException("Failed parsing PEM", e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy