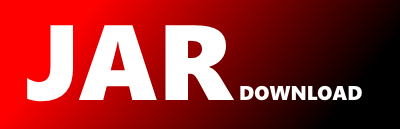
dev.fitko.fitconnect.api.services.destination.DestinationService Maven / Gradle / Ivy
Show all versions of client Show documentation
package dev.fitko.fitconnect.api.services.destination;
import dev.fitko.fitconnect.api.domain.model.destination.CreateDestination;
import dev.fitko.fitconnect.api.domain.model.destination.Destination;
import dev.fitko.fitconnect.api.domain.model.destination.Destinations;
import dev.fitko.fitconnect.api.domain.model.jwk.ApiJwk;
import dev.fitko.fitconnect.api.domain.model.jwk.ApiJwks;
import dev.fitko.fitconnect.api.exceptions.internal.RestApiException;
import java.util.UUID;
/**
* A REST-Service that provides access to the FIT-Connect API and allows the creating, updating, and the deletion of {@link Destination}s.
*
* @see FIT-Connect Destination API
*/
public interface DestinationService {
/**
* Get a {@link Destination} by id.
*
* @param destinationId unique destination identifier
* @return the {@link Destination}
* @throws RestApiException if an error occurred
*/
Destination getDestination(UUID destinationId) throws RestApiException;
/**
* Create a destination with the appropriate configuration for receiving submissions.
*
* @param destination {@link CreateDestination} payload of the destination to be created
* @return the {@link Destination} with a destinationId
* @throws RestApiException if an error occurred
*/
Destination createDestination(CreateDestination destination) throws RestApiException;
/**
* Update a given destination.
*
* @param destination the destination with fields to be updated.
* @return the updated {@link Destination}
* @throws RestApiException if an error occurred
*/
Destination updateDestination(Destination destination);
/**
* Delete a destination by id.
*
* Removal is allowed as long as it is in the 'created' status.
*
* @param destinationId unique identifier of the destination to be deleted
* @throws RestApiException if an error occurred
*/
void deleteDestination(UUID destinationId);
/**
* List all self-created destinations and their configurations.
*
* @param offset position in the dataset
* @param limit number of destinations in the result-set
* @return {@link Destinations} object with a set of destinations
* @throws RestApiException if an error occurred
*/
Destinations listDestinations(int offset, int limit) throws RestApiException;
/**
* List all public keys associated with a given destination. The keys are in JWKS RFC7517 format.
*
* @param destinationId unique identifier of the destination
* @param offset position in the dataset
* @param limit number of destinations in the result-set
* @return {@link ApiJwks} list of JWKs
* @throws RestApiException if an error occurred
*/
ApiJwks listKeys(UUID destinationId, int offset, int limit);
/**
* Retrieve a public-key of a given destination. The key is in JWKS RFC7517 format.
*
* @param destinationId unique identifier of the destination to be deleted
* @param keyId unique identifier of a destinations public key
* @return the public-key as {@link ApiJwk}
* @throws RestApiException if an error occurred
*/
ApiJwk getKey(UUID destinationId, String keyId);
/**
* Adds a new JWK to the destination.
*
* Be aware to NOT send private keys to the destination.
*
* @param destinationId unique identifier of the destination
* @param publicKey the public key for encryption or signature verification as {@link ApiJwk}
* @throws RestApiException if an error occurred
*/
void addKey(UUID destinationId, ApiJwk publicKey);
}