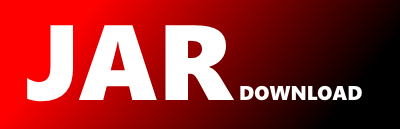
dev.fitko.fitconnect.api.services.reply.ReplyService Maven / Gradle / Ivy
package dev.fitko.fitconnect.api.services.reply;
import dev.fitko.fitconnect.api.domain.model.attachment.Attachment;
import dev.fitko.fitconnect.api.domain.model.event.authtags.AuthenticationTags;
import dev.fitko.fitconnect.api.domain.model.event.problems.Problem;
import dev.fitko.fitconnect.api.domain.model.reply.AcceptReply;
import dev.fitko.fitconnect.api.domain.model.reply.AnnounceReply;
import dev.fitko.fitconnect.api.domain.model.reply.CreatedReply;
import dev.fitko.fitconnect.api.domain.model.reply.RepliesForPickup;
import dev.fitko.fitconnect.api.domain.model.reply.Reply;
import dev.fitko.fitconnect.api.domain.model.reply.ReplyForPickup;
import dev.fitko.fitconnect.api.domain.model.reply.SentReply;
import dev.fitko.fitconnect.api.domain.model.reply.SubmitReply;
import dev.fitko.fitconnect.api.exceptions.internal.RestApiException;
import java.util.List;
import java.util.UUID;
/**
* Common Service that provides access to all reply endpoints of the FIT-Connect REST-API.
*/
public interface ReplyService {
/**
* Creates a new reply announcement without data and metadata.
*
* @param announceReply reply announcement with attachmentsId to add in the {@link ReplyService#submitReply(UUID, SubmitReply)}
* @return {@link CreatedReply}
* @throws RestApiException if a technical error occurs during the request
*/
CreatedReply announceReply(AnnounceReply announceReply) throws RestApiException;
/**
* Send reply with encrypted data and metadata payload.
*
* @param replyId the unique reply identifier created in {@link CreatedReply}
* @param submitReply reply with encrypted data and metadata
* @return {@link SentReply}
* @throws RestApiException if a technical error occurs during the request
*/
SentReply submitReply(UUID replyId, SubmitReply submitReply) throws RestApiException;
/**
* Gets a single reply by id.
*
* @param replyId the unique reply identifier
* @return {@link Reply} with encrypted data, metadata and attachments
* @throws RestApiException if a technical error occurs during the request
*/
Reply getReply(UUID replyId) throws RestApiException;
/**
* Loads all available replies ready for pickup (replies in submitted state).
*
* @param limit max entries per request
* @param offset skip n elements
* @return {@link RepliesForPickup} with all available {@link ReplyForPickup}
* @throws RestApiException if a technical error occurs during the request
*/
RepliesForPickup getAvailableReplies(int limit, int offset) throws RestApiException;
/**
* Accepts a valid reply.
*
* @param replyId max entries per request
* @param acceptReply event payload with an optional list of {@link Problem}s and {@link AuthenticationTags}
* @return confirmed event as string
* @throws RestApiException if a technical error occurs during the request
*/
String acceptReply(UUID replyId, AcceptReply acceptReply) throws RestApiException;
/**
* Rejects an invalid reply.
*
* @param problems list of {@link Problem}s that lead to the rejection
* @return confirmed event as string
* @throws RestApiException if a technical error occurs during the request
*/
String rejectReply(UUID replyId, List problems) throws RestApiException;
/**
* Uploads an encrypted {@link Attachment}.
*
* @param replyId the unique identifier of a reply the attachment should be uploaded to
* @param attachmentId the unique identifier the attachment
* @throws RestApiException if a technical error occurs during the request
*/
void uploadAttachment(UUID replyId, UUID attachmentId, String encryptedAttachment) throws RestApiException;
/**
* Loads an {@link Attachment} by id for a specific reply.
*
* @param replyId the unique identifier of a reply the attachment belongs to
* @param attachmentId the unique identifier the attachment
* @return the encrypted attachment as string
* @throws RestApiException if a technical error occurs during the request
*/
String getAttachment(UUID replyId, UUID attachmentId) throws RestApiException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy