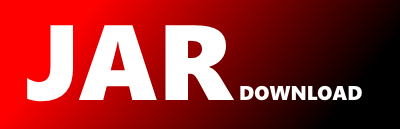
dev.fitko.fitconnect.client.bootstrap.ApplicationConfigLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client Show documentation
Show all versions of client Show documentation
Library that provides client access to the FIT-Connect api-endpoints for sending, subscribing and
routing
The newest version!
package dev.fitko.fitconnect.client.bootstrap;
import dev.fitko.fitconnect.api.config.ApplicationConfig;
import dev.fitko.fitconnect.api.config.defaults.Environments;
import dev.fitko.fitconnect.api.config.Environment;
import dev.fitko.fitconnect.api.config.EnvironmentName;
import dev.fitko.fitconnect.api.exceptions.client.FitConnectInitialisationException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.error.YAMLException;
import org.yaml.snakeyaml.introspector.BeanAccess;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.InvalidPathException;
import java.nio.file.Path;
import java.util.Map;
public final class ApplicationConfigLoader {
private static final Logger LOGGER = LoggerFactory.getLogger(ApplicationConfigLoader.class);
private static final String CONFIG_ENV_KEY_NAME = "FIT_CONNECT_CONFIG";
private ApplicationConfigLoader() {
}
/**
* Load ApplicationConfig from path.
*
* @param configPath path to config file
* @return ApplicationConfig
* @throws FitConnectInitialisationException if the config file could not be loaded
*/
public static ApplicationConfig loadConfigFromPath(final Path configPath) {
try {
return loadConfigFromYamlString(Files.readString(configPath));
} catch (final IOException | YAMLException | NullPointerException e) {
LOGGER.error("config file could not be loaded from path {}", configPath);
throw new FitConnectInitialisationException(e.getMessage(), e);
}
}
/**
* Load ApplicationConfig from FIT_CONNECT_CONFIG environment variable.
* Make sure this variable is set and points to a config.yaml.
*
* @return ApplicationConfig
* @throws FitConnectInitialisationException if the config file could not be loaded
*/
public static ApplicationConfig loadConfigFromEnvironment() {
final Path configPath = getPathFromEnvironment();
return loadConfigFromPath(configPath);
}
/**
* Load ApplicationConfig from yaml string.
*
* @param configYaml string content of the yaml config file
* @return ApplicationConfig
*/
public static ApplicationConfig loadConfigFromYamlString(final String configYaml) {
return mergeConfigEnvironmentsWithSdkDefaults(initNewYaml().loadAs(configYaml, ApplicationConfig.class));
}
/**
* Merge the configured default environments with the config from user.
*
* @param config loaded config with user overrides
* @return application config that contains default environments, overrides of the defaults user specific environments
*/
private static ApplicationConfig mergeConfigEnvironmentsWithSdkDefaults(final ApplicationConfig config) {
final Map sdkEnvironments = Environments.getEnvironmentsAsMap();
config.getEnvironments().forEach((key, value) -> {
if (sdkEnvironments.containsKey(key)) {
sdkEnvironments.put(key, value.merge(sdkEnvironments.get(key)));
} else {
sdkEnvironments.put(key, value);
}
});
return config.withEnvironments(sdkEnvironments);
}
private static Path getPathFromEnvironment() {
try {
return Path.of(System.getenv(CONFIG_ENV_KEY_NAME));
} catch (final NullPointerException | InvalidPathException e) {
LOGGER.warn("Environment variable {} could not be loaded", CONFIG_ENV_KEY_NAME);
throw new FitConnectInitialisationException(e.getMessage(), e);
}
}
private static Yaml initNewYaml() {
final Yaml yaml = new Yaml();
yaml.setBeanAccess(BeanAccess.FIELD);
return yaml;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy