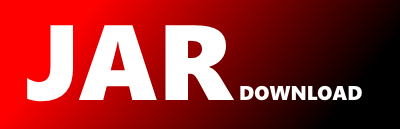
dev.fuxing.airtable.AirtableRecord Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of airtable-api Show documentation
Show all versions of airtable-api Show documentation
Java Airtable API for https://airtable.com/api
The newest version!
package dev.fuxing.airtable;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.MissingNode;
import com.fasterxml.jackson.databind.type.CollectionType;
import dev.fuxing.airtable.fields.AttachmentField;
import dev.fuxing.airtable.fields.CollaboratorField;
import org.apache.commons.lang3.time.FastDateFormat;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.text.ParseException;
import java.util.*;
/**
* Created by: Fuxing
* Date: 2019-04-20
* Time: 22:04
*/
public class AirtableRecord {
public static final ObjectMapper OBJECT_MAPPER = AirtableApi.OBJECT_MAPPER;
public static final FastDateFormat DATE_FORMAT = FastDateFormat.getInstance("yyyy-MM-dd'T'HH:mm:ss.SSS'Z'");
private String id;
private Map fields;
private Date createdTime;
/**
* @param id recordId
*/
public AirtableRecord(String id) {
this.fields = new HashMap<>();
this.id = id;
}
public AirtableRecord() {
this.fields = new HashMap<>();
}
/**
* @param node json node from https://api.airtable.com/v0
*/
public AirtableRecord(JsonNode node) {
this.id = node.path("id").asText();
this.fields = new HashMap<>();
try {
this.createdTime = DATE_FORMAT.parse(node.path("createdTime").asText());
} catch (ParseException e) {
// Shouldn't happen, wrapped in IllegalStateException
throw new IllegalStateException(e);
}
node.path("fields").fields().forEachRemaining(entry -> {
this.fields.put(entry.getKey(), entry.getValue());
});
}
/**
* @return id of the record, prefixed with 'rec'
*/
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
* @return all fields in the record
*/
public Map getFields() {
return fields;
}
public void setFields(Map fields) {
this.fields = fields;
}
/**
* @return date time when the record is created
*/
public Date getCreatedTime() {
return createdTime;
}
public void setCreatedTime(Date createdTime) {
this.createdTime = createdTime;
}
/**
* @param name of the field
* @param value string value
*/
public void putField(String name, String value) {
putField(name, (Object) value);
}
/**
* @param name of the field
* @param value int value
*/
public void putField(String name, int value) {
putField(name, (Object) value);
}
/**
* @param name of the field
* @param value long value
*/
public void putField(String name, long value) {
putField(name, (Object) value);
}
/**
* @param name of the field
* @param value double value
*/
public void putField(String name, double value) {
putField(name, (Object) value);
}
/**
* @param name of the field
* @param value boolean value
*/
public void putField(String name, boolean value) {
putField(name, (Object) value);
}
/**
* @param name of the field
* @param date object
*/
public void putField(String name, Date date) {
putField(name, (Object) DATE_FORMAT.format(date));
}
/**
* @param name of the field
* @param collaborator object
* @see CollaboratorField documents for Patch and Create operations
*/
public void putField(String name, CollaboratorField collaborator) {
putField(name, (Object) collaborator);
}
/**
* putField for any Array values
*
* @param name of the field
* @param values array object, Collaborator, Attachment, Values
*/
public void putField(String name, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy