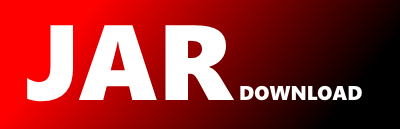
dev.fuxing.airtable.exceptions.AirtableApiException Maven / Gradle / Ivy
Show all versions of airtable-api Show documentation
package dev.fuxing.airtable.exceptions;
/**
* Exceptions that originated from https://api.airtable.com.
*
* Created by: Fuxing
* Date: 2019-04-20
* Time: 23:51
*/
public class AirtableApiException extends AirtableException {
private int code;
private String type;
/**
*
* {
* "error": {
* "type": "UNKNOWN_FIELD_NAME",
* "message": "Unknown field name: \" Name\""
* }
* }
*
*
*
* {
* "error": "NOT_FOUND"
* }
*
*
* @param code from http status
* @param type from node: 'error.type'
* @param message from node: 'error.message'
*/
public AirtableApiException(int code, String type, String message) {
super(message);
this.type = type;
this.code = code;
}
/**
* These errors generally indicate a problem on the client side.
* If you are getting one of these, check your code and the request details.
*
* User Error Code:
*
* 400: Bad Request
* The request encoding is invalid; the request can't be parsed as a valid JSON.
*
* 401: Unauthorized
* Accessing a protected resource without authorization or with invalid credentials.
*
* 402: Payment Required
* The account associated with the API key making requests hits a quota that can be increased by upgrading the Airtable account plan.
*
* 403: Forbidden
* Accessing a protected resource with API credentials that don't have access to that resource.
*
* 404: Not Found
* Route or resource is not found.
* This error is returned when the request hits an undefined route, or if the resource doesn't exist (e.g. has been deleted).
*
* 413: Request Entity Too Large
* The request exceeded the maximum allowed payload size.
* You shouldn't encounter this under normal use.
*
* 422: Invalid Request
* The request data is invalid.
* This includes most of the base-specific validations.
* You will receive a detailed error message and code pointing to the exact issue.
*
* Server Error Code:
*
* 500: Internal Server Error
* The server encountered an unexpected condition.
*
* 502: Bad Gateway
* Airtable's servers are restarting or an unexpected outage is in progress.
* You should generally not receive this error, and requests are safe to retry.
*
* 503: Service Unavailable
* The server could not process your request in time.
* The server could be temporarily unavailable, or it could have timed out processing your request.
* You should retry the request with backoffs.
*
* @return status code, refer to airtable api document.
*/
public int getCode() {
return code;
}
public String getType() {
return type;
}
@Override
public String getMessage() {
return super.getMessage();
}
}