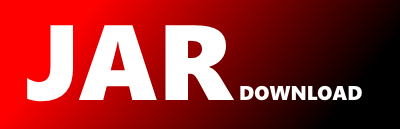
dev.galasa.cicsts.cicsresource.IJvmprofile Maven / Gradle / Ivy
/*
* Copyright contributors to the Galasa project
*/
package dev.galasa.cicsts.cicsresource;
import java.util.Map;
import dev.galasa.zosfile.IZosUNIXFile;
/**
* Represents a CICS JVM server JVM profile
*/
public interface IJvmprofile {
/**
* Set the JVM profile name in the JVM server resource definition
* @param name the profile name
* @throws CicsJvmserverResourceException
*/
public void setProfileName(String name) throws CicsJvmserverResourceException;
/**
* Set the location for the JVM profile on the zOS UNIX file system.
* WARNING: This should must equal to the CICS region JVMPROFILEDIR SIT parameter
* @param jvmProfileDir
* @throws CicsJvmserverResourceException
*/
public void setJvmProfileDir(String jvmProfileDir) throws CicsJvmserverResourceException;
/**
* Returns the JVM profile as a {@link IZosUNIXFile} object
* @return the JVM profile
*/
public IZosUNIXFile getProfile();
/**
* Returns the JVM profile name in the JVM server resource definition
* @return
*/
public String getProfileName();
/**
* Sets JVM server option or JVM system property in the JVM profile of the format key=value
.
* If the key exists, it will be replaced. To append a value to an existing option or property, use {@link #appendProfileValue(String, String)}.
* Adding the + character before key results in the value being appended to the existing option, rather than creating a new option entry
* Examples:
*
*
* Option
* key
* value
*
*
* PRINT_JVM_OPTIONS=TRUE
* PRINT_JVM_OPTIONS
* TRUE
*
*
* -Dcom.ibm.cics.jvmserver.trace.format=FULL
* -Dcom.ibm.cics.jvmserver.trace.format
* FULL
*
*
* -Xms256M
* -Xms
* 256M
*
*
* -Xshareclasses:printStats
* -Xshareclasses:
* printStats
*
*
* -Xnocompressedrefs
* -Xnocompressedrefs
* null
*
*
*
* @param key the JVM server option or JVM system property key
* @param value the JVM server option or JVM system property value
*/
public void setProfileValue(String key, String value);
/**
* Append a value to a JVM server option or JVM system property in the JVM profile of the format key=value
in the JVM profile.
* For example, given an existing JVM profile option of:
* OSGI_BUNDLES=/tmp/bundle1.jar
* the method call appendProfileValue("OSGI_BUNDLES", "/tmp/bundle2.jar")
would set the option to:
* OSGI_BUNDLES=/tmp/bundle1.jar,\\\n/tmp/bundle2.jar
*
* If the key does not exist, then this method performs the same function as {@link #setProfileValue(String, String)} *
* @param key the JVM server option or JVM system property key
* @param value the JVM server option or JVM system property value
*/
public void appendProfileValue(String key, String value);
/**
* Returns the value of a JVM server option or JVM system property in the JVM profile
* @param key the JVM server option or JVM system property key
* @return the JVM server option or JVM system property value
*/
public String getProfileValue(String key);
/**
* Removes the JVM server option or JVM system property from the JVM profile
* @param key
*/
public void removeProfileValue(String key);
/**
* Returns true it the current JVM profile object contains the given option
* @param option the JVM profile option
* @return true or false
*/
public boolean containsOption(String option);
/**
* Returns a {@link Map} of the JVM server options and JVM system property in the JVM profile
* @return content of the JVM Profile
*/
public Map getProfileMap();
/**
* Returns a {@link String} of the JVM server options and JVM system property in the JVM profile
* @return content of the JVM Profile
*/
public String getProfileString();
/**
* Returns the location for the JVM profile Directory
* @return the value of the JVM Profile Directory
*/
public String getJvmProfileDir();
/**
* Print the content of the JVM profile
*/
public void printProfile();
/**
* Build the JVM profile on the zOS UNIX file system
*
* @throws CicsJvmserverResourceException
*/
public void build() throws CicsJvmserverResourceException;
/**
* Delete the JVM profile on the zOS UNIX file system
*
* @throws CicsJvmserverResourceException
*/
public void delete() throws CicsJvmserverResourceException;
/**
* Store the content JVM profile on the zOS UNIX system to the Results Archive Store
* @param rasPath path in Results Archive Store
* @throws CicsJvmserverResourceException
*/
public void saveToResultsArchive(String rasPath) throws CicsJvmserverResourceException;
/**
* Set the value of the WLP_INSTALL_DIR
environment variable in the JVM profile
*
* Galasa sets the default value ofUSSHOME/wlp
* @param wlpInstallDir the value of WLP_INSTALL_DIR
* @throws CicsJvmserverResourceException
*/
public void setWlpInstallDir(String wlpInstallDir) throws CicsJvmserverResourceException;
/**
* Set the value of the WLP_USER_DIR
environment variable in the JVM profile
* @param wlpUserDir the value of WLP_USER_DIR
* @throws CicsJvmserverResourceException
*/
public void setWlpUserDir(String wlpUserDir) throws CicsJvmserverResourceException;
/**
* Set the value of the WLP_OUTPUT_DIR
environment variable in the JVM profile
* @param wlpOutputDir the value for WLP_OUTPUT_DIR
* @throws CicsJvmserverResourceException
*/
public void setWlpOutputDir(String wlpOutputDir) throws CicsJvmserverResourceException;
/**
* Set the value of the ZCEE_INSTALL_DIR
environment variable in the JVM profile using the value supplied
* in the Galasa Configuration Property Service
* @throws CicsJvmserverResourceException
*/
void setZosConnectInstallDir() throws CicsJvmserverResourceException;
/**
* Set the value of the ZCEE_INSTALL_DIR
environment variable in the JVM profile
* @param zOSConnectInstallDir the value of ZCEE_INSTALL_DIR
* @throws CicsJvmserverResourceException
*/
public void setZosConnectInstallDir(String zOSConnectInstallDir) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.server.name
JVM system property in the JVM profile.
* Liberty defaults this to defaultServer.
* @param serverName the value for -Dcom.ibm.cics.jvmserver.wlp.server.name
* @throws CicsJvmserverResourceException
*/
public void setWlpServerName(String serverName) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.autoconfigure
JVM system property in the JVM profile
* @param autoconfigure the value for -Dcom.ibm.cics.jvmserver.wlp.autoconfigure
* @throws CicsJvmserverResourceException
*/
public void setWlpAutoconfigure(boolean autoconfigure) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.server.host
JVM system property in the JVM profile
* @param hostname the value of -Dcom.ibm.cics.jvmserver.wlp.server.host
* @throws CicsJvmserverResourceException
*/
public void setWlpServerHost(String hostname) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.server.http.port
JVM system property in the JVM profile
* @param httpPort the value of -Dcom.ibm.cics.jvmserver.wlp.server.http.port
* @throws CicsJvmserverResourceException
*/
public void setWlpServerHttpPort(int httpPort) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.server.https.port
JVM system property in the JVM profile
* @param httpsPort the value of -Dcom.ibm.cics.jvmserver.wlp.server.https.port
* @throws CicsJvmserverResourceException
*/
public void setWlpServerHttpsPort(int httpsPort) throws CicsJvmserverResourceException;
/**
* Set the value of the -Dcom.ibm.cics.jvmserver.wlp.wab
JVM system property in the JVM profile
* @param wabEnabled the value of -Dcom.ibm.cics.jvmserver.wlp.wab
* @throws CicsJvmserverResourceException
*/
public void setWlpServerWabEnabled(boolean wabEnabled) throws CicsJvmserverResourceException;
/**
* Returns the value of the WLP_INSTALL_DIR
environment variable in the JVM profile
* @return the value of WLP_INSTALL_DIR
* @throws CicsJvmserverResourceException
*/
public String getWlpInstallDir() throws CicsJvmserverResourceException;
/**
* Returns the value of the WLP_USER_DIR
environment variable in the JVM profile
* @return the value of WLP_USER_DIR
*/
public String getWlpUserDir();
/**
* Returns the value of the WLP_OUTPUT_DIR
environment variable in the JVM profile
* @return the value of WLP_OUTPUT_DIR
*/
public String getWlpOutputDir();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.server.name
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.server.name
*/
public String getWlpServerName();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.autoconfigure
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.autoconfigure
*/
public String getWlpAutoconfigure();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.server.host
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.server.host
*/
public String getWlpServerHost();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.server.http.port
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.server.http.port
*/
public String getWlpServerHttpPort();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.server.https.port
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.server.https.port
*/
public String getWlpServerHttpsPort();
/**
* Returns the value of the -Dcom.ibm.cics.jvmserver.wlp.wab
JVM system property in the JVM profile
* @return the value of -Dcom.ibm.cics.jvmserver.wlp.wab
*/
public boolean getWlpServerWabEnabled();
}