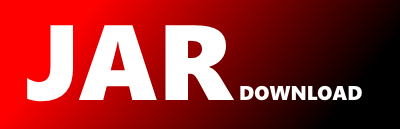
dev.galasa.framework.FrameworkRuns Maven / Gradle / Ivy
/*
* Copyright contributors to the Galasa project
*
* SPDX-License-Identifier: EPL-2.0
*/
package dev.galasa.framework;
import java.time.Instant;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.UUID;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.validation.constraints.NotNull;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import com.google.gson.JsonArray;
import dev.galasa.framework.beans.Property;
import dev.galasa.framework.beans.SubmitRunRequest;
import dev.galasa.framework.spi.AbstractManager;
import dev.galasa.framework.spi.ConfigurationPropertyStoreException;
import dev.galasa.framework.spi.DynamicStatusStoreException;
import dev.galasa.framework.spi.FrameworkException;
import dev.galasa.framework.spi.IConfigurationPropertyStoreService;
import dev.galasa.framework.spi.IDynamicStatusStoreService;
import dev.galasa.framework.spi.IFramework;
import dev.galasa.framework.spi.IFrameworkRuns;
import dev.galasa.framework.spi.IRun;
import dev.galasa.framework.spi.utils.GalasaGson;
import dev.galasa.framework.spi.utils.GalasaGsonBuilder;
public class FrameworkRuns implements IFrameworkRuns {
private final static Log logger = LogFactory.getLog(FrameworkRuns.class);
private final Pattern runPattern = Pattern.compile("^\\Qrun.\\E(\\w+)\\Q.\\E.*$");
private final IFramework framework;
private final IDynamicStatusStoreService dss;
private final IConfigurationPropertyStoreService cps;
private final String NO_GROUP = "none";
private final String NO_BUNDLE = "none";
private final String NO_RUNTYPE = "UNKNOWN";
// private final String NO_REQUESTER = "unknown";
private final String RUN_PREFIX = "run.";
private final GalasaGson gson = new GalasaGson();
public FrameworkRuns(IFramework framework) throws FrameworkException {
this.framework = framework;
this.dss = framework.getDynamicStatusStoreService("framework");
this.cps = framework.getConfigurationPropertyService("framework");
gson.setGsonBuilder(new GalasaGsonBuilder(false));
}
@Override
public List getActiveRuns() throws FrameworkException {
List runs = getAllRuns();
Iterator iruns = runs.iterator();
while (iruns.hasNext()) {
IRun run = iruns.next();
if (run.getHeartbeat() != null) {
continue;
}
if ("allocated".equals(run.getStatus())) {
continue;
}
if (run.isSharedEnvironment()) {
continue;
}
iruns.remove();
}
return runs;
}
@Override
public @NotNull List getQueuedRuns() throws FrameworkException {
List runs = getAllRuns();
Iterator iruns = runs.iterator();
while (iruns.hasNext()) {
IRun run = iruns.next();
if (!"queued".equals(run.getStatus())) {
iruns.remove();
}
}
return runs;
}
@Override
public List getAllRuns() throws FrameworkException {
HashMap runs = new HashMap<>();
logger.trace("Fetching all runs from DSS");
Map runProperties = dss.getPrefix(RUN_PREFIX);
logger.trace("Fetched all runs from DSS");
for (String key : runProperties.keySet()) {
Matcher matcher = runPattern.matcher(key);
if (matcher.find()) {
String runName = matcher.group(1);
if (!runs.containsKey(runName)) {
runs.put(runName, new RunImpl(runName, this.dss));
}
}
}
LinkedList returnRuns = new LinkedList<>(runs.values());
return returnRuns;
}
@Override
public List getAllGroupedRuns(@NotNull String groupName) throws FrameworkException {
List allRuns = this.getAllRuns();
List groupedRuns = new LinkedList();
for (IRun run : allRuns) {
if (groupName.equals(run.getGroup())) {
groupedRuns.add(run);
}
}
return groupedRuns;
}
@Override
public @NotNull Set getActiveRunNames() throws FrameworkException {
List runs = getActiveRuns();
HashSet runNames = new HashSet<>();
for (IRun run : runs) {
runNames.add(run.getName());
}
return runNames;
}
private @NotNull IRun submitRun(SubmitRunRequest runRequest) throws FrameworkException {
IRun run = null;
setRunRequestDefaultsIfNotSet(runRequest);
if (runRequest.getSharedEnvironmentPhase() != null) {
run = submitSharedEnvironmentRun(runRequest);
} else {
try {
String runName = assignNewRunName(runRequest);
run = new RunImpl(runName, this.dss);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new FrameworkException("Interrupted", e);
} catch (Exception e) {
throw new FrameworkException("Problem submitting job", e);
}
}
return run;
}
@Override
@NotNull
public @NotNull IRun submitRun(String runType, String requestor, String bundleName,
@NotNull String testName, String groupName, String mavenRepository, String obr, String stream,
boolean local, boolean trace, Properties overrides, SharedEnvironmentPhase sharedEnvironmentPhase, String sharedEnvironmentRunName,
String language) throws FrameworkException {
SubmitRunRequest runRequest = new SubmitRunRequest(
runType,
requestor,
bundleName,
testName,
groupName,
mavenRepository,
obr,
stream,
local,
trace,
overrides,
sharedEnvironmentPhase,
sharedEnvironmentRunName,
language
);
return submitRun(runRequest);
}
private boolean storeRun(String runName, SubmitRunRequest runRequest) throws DynamicStatusStoreException {
String bundleName = runRequest.getBundleName();
String testName = runRequest.getTestName();
String bundleTest = runRequest.getBundleTest();
String gherkinTest = runRequest.getGherkinTest();
String runType = runRequest.getRunType();
String mavenRepository = runRequest.getMavenRepository();
String obr = runRequest.getObr();
String stream = runRequest.getStream();
String groupName = runRequest.getGroupName();
String requestor = runRequest.getRequestor();
SharedEnvironmentPhase sharedEnvironmentPhase = runRequest.getSharedEnvironmentPhase();
Properties overrides = runRequest.getOverrides();
boolean local = runRequest.isLocalRun();
boolean trace = runRequest.isTraceEnabled();
String runPropertyPrefix = RUN_PREFIX + runName;
// *** Set up the otherRunProperties that will go with the Run number
HashMap otherRunProperties = new HashMap<>();
otherRunProperties.put(runPropertyPrefix + ".status", "queued");
otherRunProperties.put(runPropertyPrefix + ".queued", Instant.now().toString());
otherRunProperties.put(runPropertyPrefix + ".testbundle", bundleName);
otherRunProperties.put(runPropertyPrefix + ".testclass", testName);
otherRunProperties.put(runPropertyPrefix + ".request.type", runType);
otherRunProperties.put(runPropertyPrefix + ".local", Boolean.toString(local));
if (trace) {
otherRunProperties.put(runPropertyPrefix + ".trace", "true");
}
if (mavenRepository != null) {
otherRunProperties.put(runPropertyPrefix + ".repository", mavenRepository);
}
if (obr != null) {
otherRunProperties.put(runPropertyPrefix + ".obr", obr);
}
if (stream != null) {
otherRunProperties.put(runPropertyPrefix + ".stream", stream);
}
if (groupName != null) {
otherRunProperties.put(runPropertyPrefix + ".group", groupName);
} else {
otherRunProperties.put(runPropertyPrefix + ".group", UUID.randomUUID().toString());
}
otherRunProperties.put(runPropertyPrefix + ".requestor", requestor);
if (sharedEnvironmentPhase != null) {
otherRunProperties.put(runPropertyPrefix + ".shared.environment", "true");
overrides.put("framework.run.shared.environment.phase", sharedEnvironmentPhase.toString());
}
if (gherkinTest != null) {
otherRunProperties.put(runPropertyPrefix + ".gherkin", gherkinTest);
}
// *** Add in the overrides as a single property
if (!overrides.isEmpty()) {
JsonArray overridesArray = new JsonArray();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy