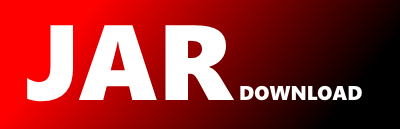
dev.galasa.ipnetwork.ICommandShell Maven / Gradle / Ivy
/*
* Licensed Materials - Property of IBM
*
* (c) Copyright IBM Corp. 2019.
*/
package dev.galasa.ipnetwork;
import dev.galasa.ipnetwork.internal.ssh.SSHException;
public interface ICommandShell {
public String issueCommand(String command) throws IpNetworkManagerException;
/**
* Issue a command using SSH. Equivalent to {@link #issueCommand(String, false,
* long)}
*
* @param command - command to issue
* @param timeout - time (in milliseconds) to wait with no new output appearing
* before timing out
* @return the output of the command (stdout and stderr)
* @throws SSHException
*/
public String issueCommand(String command, long timeout) throws IpNetworkManagerException;
/**
* Issue a command using SSH. Equivalent to
* {@link #issueCommand(String, boolean, defaultTimeout)}
*
* @param command - command to issue
* @param newShell - if true will start a new
* @return the output of the command (stdout and stderr)
* @throws SSHException
*/
public String issueCommand(String command, boolean newShell) throws IpNetworkManagerException;
/**
* Issue a command using SSH
*
* @param command - command to issue
* @param newShell - if true will start a new
* @param timeout - time (in milliseconds) to wait with no new output appearing
* before timing out
* @return the output of the command (stdout and stderr)
* @throws SSHException
*/
public String issueCommand(String command, boolean newShell, long timeout) throws IpNetworkManagerException;
// public void changeUser(String userid, String password);
public void connect() throws IpNetworkManagerException;
public void disconnect() throws IpNetworkManagerException;
public void restartShell() throws IpNetworkManagerException;
/**
* Define the right command used to change the shell prompt
*/
public void setChangePromptCommand(String command);
/**
* Issue a command using SSH shell. Equivalent to
* {@link #issueCommandToShell(String, false, defaultTimeout)} - not valid for
* Rexec implementation - equivalent to
* {@link #issueCommand(String, boolean, long)} for Telnet implementation
*
* @param command - command to issue
* @return the output of the command (stdout and stderr)
* @throws IpNetworkManagerException
*/
public String issueCommandToShell(String command) throws IpNetworkManagerException;
/**
* Issue a command using SSH shell. Equivalent to
* {@link #issueCommandToShell(String, false, long)} - not valid for Rexec
* implementation - equivalent to {@link #issueCommand(String, boolean, long)}
* for Telnet implementation
*
* @param command - command to issue - if true will start a new
* @return the output of the command (stdout and stderr)
* @throws IpNetworkManagerException
*/
public String issueCommandToShell(String command, long timeout) throws IpNetworkManagerException;
/**
* Issue a command using SSH shell. Equivalent to
* {@link #issueCommandToShell(String, boolean, defaultTimeout)} - not valid for
* Rexec implementation - equivalent to
* {@link #issueCommand(String, boolean, long)} for Telnet implementation
*
* @param command - command to issue
* @param newShell - if true will start a new
* @return the output of the command (stdout and stderr)
* @throws IpNetworkManagerException
*/
public String issueCommandToShell(String command, boolean newShell) throws IpNetworkManagerException;
/**
* Issue a command using SSH shell - not valid for Rexec implementation -
* equivalent to {@link #issueCommand(String, boolean, long)} for Telnet
* implementation
*
* @param command - command to issue
* @param newShell - if true will start a new
* @param timeout - time (in milliseconds) to wait with no new output appearing
* before timing out
* @return the output of the command
* @throws IpNetworkManagerException
*/
public String issueCommandToShell(String command, boolean newShell, long timeout) throws IpNetworkManagerException;
/**
* Ask the shell to log the result strings for all shell commands
*
* @param report whether the shell should log the results of the shell commands
*/
public void reportResultStrings(boolean report);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy