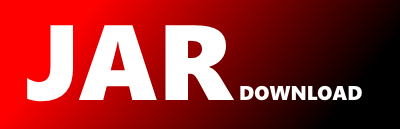
dev.galasa.boot.Launcher Maven / Gradle / Ivy
/*
* Copyright contributors to the Galasa project
*
* SPDX-License-Identifier: EPL-2.0
*/
package dev.galasa.boot;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.URLConnection;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import java.util.Set;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import dev.galasa.boot.BootLogger.Level;
import dev.galasa.boot.felix.FelixFramework;
/**
* Galasa command line launcher.
*
* Required parameters:
* --obr infra.obr --obr test.obr test.bundle/test.package.TestClass
*
*
* - Start Felix OSGi framework
* - Loads required bundles
* - Loads OSGi Bundle repositories from one or more
--obr
* parameters
* - Loads test bundle
test.bundle
from OBR
* - Loads and runs test class
test.package.TestClass
*
*
*/
public class Launcher {
private static final String OBR_OPTION = "obr";
private static final String BOOTSTRAP_OPTION = "bootstrap";
private static final String OVERRIDES_OPTION = "overrides";
private static final String RESOURCEMANAGEMENT_OPTION = "resourcemanagement";
private static final String K8SCONTROLLER_OPTION = "k8scontroller";
private static final String API_OPTION = "api";
private static final String DOCKERCONTROLLER_OPTION = "dockercontroller";
private static final String METRICSERVER_OPTION = "metricserver";
private static final String TEST_OPTION = "test";
private static final String RUN_OPTION = "run";
private static final String GHERKIN_OPTION = "gherkin";
private static final String BUNDLE_OPTION = "bundle";
private static final String METRICS_OPTION = "metrics";
private static final String HEALTH_OPTION = "health";
private static final String LOCALMAVEN_OPTION = "localmaven";
private static final String REMOTEMAVEN_OPTION = "remotemaven";
private static final String TRACE_OPTION = "trace";
private static final String BACKUPCPS_OPTION = "backupcps";
private static final String RESTORECPS_OPTION = "restorecps";
private static final String FILE_OPTION = "f";
private static final String FILE_OPTION_LONG = "file";
private static final String DRY_RUN_OPTION = "dryrun";
private static final String SETUPECO_OPTION = "setupeco";
private static final String VALIDATEECO_OPTION = "validateeco";
private static final String USER_HOME = "user.home";
private static final BootLogger logger = new BootLogger();
private List bundleRepositories = new ArrayList<>();
private String testName;
private String testBundleName;
private String testClassName;
private String runName;
private String gherkinName;
private String filePath;
private String galasaHome;
private FelixFramework felixFramework;
private Properties bootstrapProperties;
private Properties overridesProperties;
private boolean testRun;
private boolean resourceManagement;
private boolean k8sController;
private boolean dockerController;
private boolean metricsServer;
private boolean api;
private boolean backupCPS;
private boolean restoreCPS;
private boolean dryRun;
private boolean setupEco;
private boolean validateEco;
private Integer metrics;
private Integer health;
private List bundles = new ArrayList<>();
private URL localMavenRepo;
private List remoteMavenRepos = new ArrayList<>();
public Environment env = new SystemEnvironment();
/**
* Launcher main method
*
* @param args Arguments from the command line
*/
public static void main(String[] args) {
Launcher launcher = new Launcher();
try {
launcher.launch(args);
} catch(Exception e) {
logger.error("Exiting launcher due to exception",e);
System.exit(16);
}
System.exit(0);
}
/**
* Process supplied command line arguments and run the test
*
* @param args test run parameters
* @throws InterruptedException
* @throws Exception
*/
protected void launch(String[] args) throws LauncherException, InterruptedException {
validateJavaLevel(env);
this.galasaHome = getGalasaHome(env);
felixFramework = new FelixFramework();
// Build Felix framework and install required bundles
try {
processCommandLine(args);
} catch (ParseException e) {
throw new LauncherException("Unable to parse command line arguments", e);
}
logger.debug("OBR Repository Files: " + bundleRepositories);
logger.debug("Launching Framework...");
try {
// Build the Framework
buildFramework();
if (testRun) {
if (testBundleName != null && testClassName != null) {
// Run test class
logger.debug("Test Bundle: " + testBundleName);
logger.debug("Test Class: " + testClassName);
overridesProperties.setProperty("framework.run.testbundleclass", this.testName);
} else if (runName != null) {
logger.debug("Test Run: " + runName);
overridesProperties.setProperty("framework.run.name", this.runName);
} else {
logger.debug("Gherkin Run: " + gherkinName.toString());
overridesProperties.setProperty("framework.run.gherkintest", this.gherkinName);
}
felixFramework.runTest(bootstrapProperties, overridesProperties);
} else if (resourceManagement) {
logger.debug("Resource Management");
felixFramework.runResourceManagement(bootstrapProperties, overridesProperties, bundles, metrics, health);
} else if (k8sController) {
logger.debug("Kubernetes Controller");
felixFramework.runK8sController(bootstrapProperties, overridesProperties, bundles, metrics, health);
} else if (dockerController) {
logger.debug("Docker Controller");
felixFramework.runDockerController(bootstrapProperties, overridesProperties, bundles, metrics, health);
} else if (metricsServer) {
logger.debug("Metrics Server");
felixFramework.runMetricsServer(bootstrapProperties, overridesProperties, bundles, metrics, health);
} else if (api) {
logger.debug("Web API Server");
felixFramework.runWebApiServer(bootstrapProperties, overridesProperties, bundles, metrics, health);
} else if (backupCPS) {
logger.debug("Back Up CPS Properties");
felixFramework.runBackupCPS(bootstrapProperties, overridesProperties, filePath);
} else if (restoreCPS) {
felixFramework.runRestoreCPS(bootstrapProperties, overridesProperties, filePath, dryRun);
} else if (setupEco) {
felixFramework.runSetupEcosystem(bootstrapProperties, overridesProperties);
} else if (validateEco) {
felixFramework.runValidateEcosystem(bootstrapProperties, overridesProperties);
}
} catch (LauncherException e) {
logger.error("Unable to run test class", e);
throw e;
} finally {
if (felixFramework != null) {
felixFramework.stopFramework();
}
}
logger.info("Boot complete");
}
private void buildFramework() throws LauncherException {
logger.debug("Launching Framework...");
try {
felixFramework.buildFramework(bundleRepositories, this.bootstrapProperties, localMavenRepo,
remoteMavenRepos, galasaHome);
} catch (Exception e) {
throw new LauncherException("Unable to create and initialize Felix framework", e);
}
}
/**
* Process the supplied command line augments
*
* @param args supplied arguments
* @throws ParseException
*/
private void processCommandLine(String[] args) throws ParseException {
StringBuilder messageBuffer = new StringBuilder();
messageBuffer.append("Supplied command line arguments: ");
for (String arg : args) {
messageBuffer.append(arg + " ");
}
logger.debug(messageBuffer.toString());
Options options = new Options();
Option propertyOption = Option.builder().longOpt("D").argName("property=value").hasArgs().valueSeparator()
.numberOfArgs(2).desc("use value for given properties").build();
options.addOption(propertyOption);
options.addOption(null, OBR_OPTION, true, "Felix OBR Repository File name");
options.addOption(null, BOOTSTRAP_OPTION, true, "Bootstrap properties file url");
options.addOption(null, OVERRIDES_OPTION, true, "Overrides properties file url");
options.addOption(null, RESOURCEMANAGEMENT_OPTION, false, "A Resource Management server");
options.addOption(null, K8SCONTROLLER_OPTION, false, "A k8s Controller server");
options.addOption(null, API_OPTION, false, "A Web App server, list bundles to load or ALL");
options.addOption(null, DOCKERCONTROLLER_OPTION, false, "A Docker Controller server");
options.addOption(null, METRICSERVER_OPTION, false, "A Metrics server");
options.addOption(null, TEST_OPTION, true, "The test to run");
options.addOption(null, RUN_OPTION, true, "The run name");
options.addOption(null, GHERKIN_OPTION, true, "The gherkin test to run");
options.addOption(null, BUNDLE_OPTION, true, "Extra bundles to load");
options.addOption(null, METRICS_OPTION, true, "The port the metrics server will open, 0 to disable");
options.addOption(null, HEALTH_OPTION, true, "The port the health server will open, 0 to disable");
options.addOption(null, LOCALMAVEN_OPTION, true, "The local maven repository, defaults to ~/.m2/repository");
options.addOption(null, REMOTEMAVEN_OPTION, true, "The remote maven repositories, defaults to central");
options.addOption(null, TRACE_OPTION, false, "Enable TRACE logging");
options.addOption(null, BACKUPCPS_OPTION, false, "Back up CPS properties to file");
options.addOption(null, RESTORECPS_OPTION, false, "Restore CPS properties from file");
options.addOption(FILE_OPTION, FILE_OPTION_LONG, true, "File for data input/output");
options.addOption(null, DRY_RUN_OPTION, false, "Perform a dry-run of the specified actions. Can be combined with \"" + FILE_OPTION_LONG + "\"");
options.addOption(null, SETUPECO_OPTION, false, "Setup the Galasa Ecosystem");
options.addOption(null, VALIDATEECO_OPTION, false, "Validate the Galasa Ecosystem");
CommandLineParser parser = new DefaultParser();
CommandLine commandLine = null;
commandLine = parser.parse(options, args);
if (commandLine.hasOption("D")) {
Properties commandLinePropety = commandLine.getOptionProperties("D");
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy