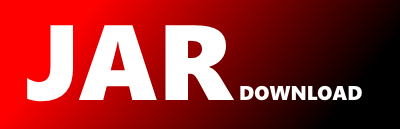
org.gradle.api.internal.changedetection.state.InMemoryTaskArtifactCache Maven / Gradle / Ivy
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.internal.changedetection.state;
import com.google.common.cache.*;
import org.gradle.api.internal.cache.HeapProportionalCacheSizer;
import com.google.common.collect.ImmutableSet;
import org.gradle.api.logging.LogLevel;
import org.gradle.api.logging.Logger;
import org.gradle.api.logging.Logging;
import org.gradle.cache.internal.CacheDecorator;
import org.gradle.cache.internal.FileLock;
import org.gradle.cache.internal.MultiProcessSafePersistentIndexedCache;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class InMemoryTaskArtifactCache implements CacheDecorator {
private final static Logger LOG = Logging.getLogger(InMemoryTaskArtifactCache.class);
private final static Object NULL = new Object();
private static final Map CACHE_CAPS = new CacheCapSizer().calculateCaps();
private static final Set WEAK_REFERENCE_CACHES = ImmutableSet.copyOf(new String[]{"fileSnapshots"});
static class CacheCapSizer {
private static final Map DEFAULT_CAP_SIZES = new HashMap();
static {
DEFAULT_CAP_SIZES.put("fileSnapshots", 10000);
DEFAULT_CAP_SIZES.put("fileSnapshotsToTreeSnapshotsIndex", 10000);
DEFAULT_CAP_SIZES.put("treeSnapshots", 20000);
DEFAULT_CAP_SIZES.put("treeSnapshotUsage", 20000);
DEFAULT_CAP_SIZES.put("taskArtifacts", 2000);
DEFAULT_CAP_SIZES.put("fileHashes", 400000);
DEFAULT_CAP_SIZES.put("compilationState", 1000);
}
final HeapProportionalCacheSizer sizer;
CacheCapSizer(int maxHeapMB) {
this.sizer = maxHeapMB > 0 ? new HeapProportionalCacheSizer(maxHeapMB) : new HeapProportionalCacheSizer();
}
CacheCapSizer() {
this(0);
}
public Map calculateCaps() {
Map capSizes = new HashMap();
for (Map.Entry entry : DEFAULT_CAP_SIZES.entrySet()) {
capSizes.put(entry.getKey(), sizer.scaleCacheSize(entry.getValue()));
}
return capSizes;
}
}
private final Object lock = new Object();
private final Cache> cache = CacheBuilder.newBuilder()
.maximumSize(CACHE_CAPS.size() * 2) //X2 to factor in a child build (for example buildSrc)
.build();
private final Map states = new HashMap();
public MultiProcessSafePersistentIndexedCache decorate(final String cacheId, String cacheName, final MultiProcessSafePersistentIndexedCache original) {
final Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy