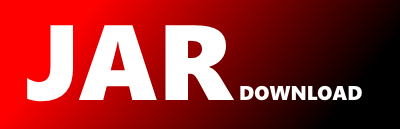
org.gradle.api.Project Maven / Gradle / Ivy
/*
* Copyright 2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api;
import groovy.lang.Closure;
import groovy.lang.MissingPropertyException;
import org.gradle.api.artifacts.ConfigurationContainer;
import org.gradle.api.artifacts.dsl.ArtifactHandler;
import org.gradle.api.artifacts.dsl.DependencyHandler;
import org.gradle.api.artifacts.dsl.RepositoryHandler;
import org.gradle.api.component.SoftwareComponentContainer;
import org.gradle.api.file.*;
import org.gradle.api.initialization.dsl.ScriptHandler;
import org.gradle.internal.HasInternalProtocol;
import org.gradle.api.invocation.Gradle;
import org.gradle.api.logging.Logger;
import org.gradle.api.logging.LoggingManager;
import org.gradle.api.plugins.*;
import org.gradle.api.resources.ResourceHandler;
import org.gradle.api.tasks.TaskContainer;
import org.gradle.api.tasks.WorkResult;
import org.gradle.process.ExecResult;
import org.gradle.process.ExecSpec;
import org.gradle.process.JavaExecSpec;
import java.io.File;
import java.net.URI;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* This interface is the main API you use to interact with Gradle from your build file. From a Project
,
* you have programmatic access to all of Gradle's features.
*
* Lifecycle
*
* There is a one-to-one relationship between a Project
and a {@value #DEFAULT_BUILD_FILE}
* file. During build initialisation, Gradle assembles a Project
object for each project which is to
* participate in the build, as follows:
*
*
*
* - Create a {@link org.gradle.api.initialization.Settings} instance for the build.
*
* - Evaluate the
{@value org.gradle.api.initialization.Settings#DEFAULT_SETTINGS_FILE}
script, if
* present, against the {@link org.gradle.api.initialization.Settings} object to configure it.
*
* - Use the configured {@link org.gradle.api.initialization.Settings} object to create the hierarchy of
*
Project
instances.
*
* - Finally, evaluate each
Project
by executing its {@value #DEFAULT_BUILD_FILE}
file, if
* present, against the project. The projects are evaluated in breadth-wise order, such that a project is evaluated
* before its child projects. This order can be overridden by calling {@link #evaluationDependsOnChildren()}
or by adding an
* explicit evaluation dependency using {@link #evaluationDependsOn(String)}
.
*
*
*
* Tasks
*
* A project is essentially a collection of {@link Task} objects. Each task performs some basic piece of work, such
* as compiling classes, or running unit tests, or zipping up a WAR file. You add tasks to a project using one of the
* {@code create()} methods on {@link TaskContainer}, such as {@link TaskContainer#create(String)}. You can locate existing
* tasks using one of the lookup methods on {@link TaskContainer}, such as {@link org.gradle.api.tasks.TaskCollection#getByName(String)}.
*
* Dependencies
*
* A project generally has a number of dependencies it needs in order to do its work. Also, a project generally
* produces a number of artifacts, which other projects can use. Those dependencies are grouped in configurations, and
* can be retrieved and uploaded from repositories. You use the {@link org.gradle.api.artifacts.ConfigurationContainer}
* returned by {@link #getConfigurations()} method to manage the configurations. The {@link
* org.gradle.api.artifacts.dsl.DependencyHandler} returned by {@link #getDependencies()} method to manage the
* dependencies. The {@link org.gradle.api.artifacts.dsl.ArtifactHandler} returned by {@link #getArtifacts()} method to
* manage the artifacts. The {@link org.gradle.api.artifacts.dsl.RepositoryHandler} returned by {@link
* #getRepositories()} method to manage the repositories.
*
* Multi-project Builds
*
* Projects are arranged into a hierarchy of projects. A project has a name, and a fully qualified path which
* uniquely identifies it in the hierarchy.
*
* Plugins
*
*
* Plugins can be used to modularise and reuse project configuration.
* Plugins can be applied using the {@link PluginAware#apply(java.util.Map)} method, or by using the {@link org.gradle.plugin.use.PluginDependenciesSpec plugins script block}.
*
*
* Properties
*
* Gradle executes the project's build file against the Project
instance to configure the project. Any
* property or method which your script uses is delegated through to the associated Project
object. This
* means, that you can use any of the methods and properties on the Project
interface directly in your script.
*
For example:
*
* defaultTasks('some-task') // Delegates to Project.defaultTasks()
* reportsDir = file('reports') // Delegates to Project.file() and the Java Plugin
*
* You can also access the Project
instance using the project
property. This can make the
* script clearer in some cases. For example, you could use project.name
rather than name
to
* access the project's name.
*
* A project has 5 property 'scopes', which it searches for properties. You can access these properties by name in
* your build file, or by calling the project's {@link #property(String)} method. The scopes are:
*
*
*
* - The
Project
object itself. This scope includes any property getters and setters declared by the
* Project
implementation class. For example, {@link #getRootProject()} is accessible as the
* rootProject
property. The properties of this scope are readable or writable depending on the presence
* of the corresponding getter or setter method.
*
* - The extra properties of the project. Each project maintains a map of extra properties, which
* can contain any arbitrary name -> value pair. Once defined, the properties of this scope are readable and writable.
* See extra properties for more details.
*
* - The extensions added to the project by the plugins. Each extension is available as a read-only property with the same name as the extension.
*
* - The convention properties added to the project by the plugins. A plugin can add properties and methods
* to a project through the project's {@link Convention} object. The properties of this scope may be readable or writable, depending on the convention objects.
*
* - The tasks of the project. A task is accessible by using its name as a property name. The properties of this
* scope are read-only. For example, a task called
compile
is accessible as the compile
* property.
*
* - The extra properties and convention properties inherited from the project's parent, recursively up to the root
* project. The properties of this scope are read-only.
*
*
*
* When reading a property, the project searches the above scopes in order, and returns the value from the first
* scope it finds the property in. If not found, an exception is thrown. See {@link #property(String)} for more details.
*
* When writing a property, the project searches the above scopes in order, and sets the property in the first scope
* it finds the property in. If not found, an exception is thrown. See {@link #setProperty(String, Object)} for more details.
*
* Extra Properties
*
* All extra properties must be defined through the "ext" namespace. Once an extra property has been defined,
* it is available directly on the owning object (in the below case the Project, Task, and sub-projects respectively) and can
* be read and updated. Only the initial declaration that needs to be done via the namespace.
*
*
* project.ext.prop1 = "foo"
* task doStuff {
* ext.prop2 = "bar"
* }
* subprojects { ext.${prop3} = false }
*
*
* Reading extra properties is done through the "ext" or through the owning object.
*
*
* ext.isSnapshot = version.endsWith("-SNAPSHOT")
* if (isSnapshot) {
* // do snapshot stuff
* }
*
*
* Dynamic Methods
*
* A project has 5 method 'scopes', which it searches for methods:
*
*
*
* - The
Project
object itself.
*
* - The build file. The project searches for a matching method declared in the build file.
*
* - The extensions added to the project by the plugins. Each extension is available as a method which takes
* a closure or {@link org.gradle.api.Action} as a parameter.
*
* - The convention methods added to the project by the plugins. A plugin can add properties and method to
* a project through the project's {@link Convention} object.
*
* - The tasks of the project. A method is added for each task, using the name of the task as the method name and
* taking a single closure or {@link org.gradle.api.Action} parameter. The method calls the {@link Task#configure(groovy.lang.Closure)} method for the
* associated task with the provided closure. For example, if the project has a task called
compile
, then a
* method is added with the following signature: void compile(Closure configureClosure)
.
*
* - The methods of the parent project, recursively up to the root project.
*
* - A property of the project whose value is a closure. The closure is treated as a method and called with the provided parameters.
* The property is located as described above.
*
*
*/
@HasInternalProtocol
public interface Project extends Comparable, ExtensionAware, PluginAware {
/**
* The default project build file name.
*/
public static final String DEFAULT_BUILD_FILE = "build.gradle";
/**
* The hierarchy separator for project and task path names.
*/
public static final String PATH_SEPARATOR = ":";
/**
* The default build directory name.
*/
public static final String DEFAULT_BUILD_DIR_NAME = "build";
public static final String GRADLE_PROPERTIES = "gradle.properties";
public static final String SYSTEM_PROP_PREFIX = "systemProp";
public static final String DEFAULT_VERSION = "unspecified";
public static final String DEFAULT_STATUS = "release";
/**
* Returns the root project for the hierarchy that this project belongs to. In the case of a single-project
* build, this method returns this project.
*
* @return The root project. Never returns null.
*/
Project getRootProject();
/**
* Returns the root directory of this project. The root directory is the project directory of the root
* project.
*
* @return The root directory. Never returns null.
*/
File getRootDir();
/**
* Returns the build directory of this project. The build directory is the directory which all artifacts are
* generated into. The default value for the build directory is projectDir/build
*
* @return The build directory. Never returns null.
*/
File getBuildDir();
/**
* Sets the build directory of this project. The build directory is the directory which all artifacts are
* generated into. The path parameter is evaluated as described for {@link #file(Object)}. This mean you can use,
* amongst other things, a relative or absolute path or File object to specify the build directory.
*
* @param path The build directory. This is evaluated as per {@link #file(Object)}
*/
void setBuildDir(Object path);
/**
* Returns the build file Gradle will evaluate against this project object. The default is {@value
* #DEFAULT_BUILD_FILE}
. If an embedded script is provided the build file will be null.
*
* @return Current build file. May return null.
*/
File getBuildFile();
/**
* Returns the parent project of this project, if any.
*
* @return The parent project, or null if this is the root project.
*/
Project getParent();
/**
* Returns the name of this project. The project's name is not necessarily unique within a project hierarchy. You
* should use the {@link #getPath()} method for a unique identifier for the project.
*
* @return The name of this project. Never return null.
*/
String getName();
/**
* Returns the description of this project.
*
* @return the description. May return null.
*/
String getDescription();
/**
* Sets a description for this project.
*
* @param description The description of the project. Might be null.
*/
void setDescription(String description);
/**
* Returns the group of this project. Gradle always uses the {@code toString()} value of the group. The group
* defaults to the path with dots as separators.
*
* @return The group of this project. Never returns null.
*/
Object getGroup();
/**
* Sets the group of this project.
*
* @param group The group of this project. Must not be null.
*/
void setGroup(Object group);
/**
* Returns the version of this project. Gradle always uses the {@code toString()} value of the version. The
* version defaults to {@value #DEFAULT_VERSION}.
*
* @return The version of this project. Never returns null.
*/
Object getVersion();
/**
* Sets the version of this project.
*
* @param version The version of this project. Must not be null.
*/
void setVersion(Object version);
/**
* Returns the status of this project. Gradle always uses the {@code toString()} value of the status. The status
* defaults to {@value #DEFAULT_STATUS}.
*
* The status of the project is only relevant, if you upload libraries together with a module descriptor. The
* status specified here, will be part of this module descriptor.
*
* @return The status of this project. Never returns null.
*/
Object getStatus();
/**
* Sets the status of this project.
*
* @param status The status. Must not be null.
*/
void setStatus(Object status);
/**
* Returns the direct children of this project.
*
* @return A map from child project name to child project. Returns an empty map if this project does not have
* any children.
*/
Map getChildProjects();
/**
* Sets a property of this project. This method searches for a property with the given name in the following
* locations, and sets the property on the first location where it finds the property.
*
*
*
* - The project object itself. For example, the
rootDir
project property.
*
* - The project's {@link Convention} object. For example, the
srcRootName
java plugin
* property.
*
* - The project's extra properties.
*
*
*
* If the property is not found, a {@link groovy.lang.MissingPropertyException} is thrown.
*
* @param name The name of the property
* @param value The value of the property
*/
void setProperty(String name, Object value) throws MissingPropertyException;
/**
* Returns this project. This method is useful in build files to explicitly access project properties and
* methods. For example, using project.name
can express your intent better than using
* name
. This method also allows you to access project properties from a scope where the property may
* be hidden, such as, for example, from a method or closure.
*
* @return This project. Never returns null.
*/
Project getProject();
/**
* Returns the set containing this project and its subprojects.
*
* @return The set of projects.
*/
Set getAllprojects();
/**
* Returns the set containing the subprojects of this project.
*
* @return The set of projects. Returns an empty set if this project has no subprojects.
*/
Set getSubprojects();
/**
* Creates a {@link Task} with the given name and adds it to this project. Calling this method is equivalent to
* calling {@link #task(java.util.Map, String)} with an empty options map.
*
* After the task is added to the project, it is made available as a property of the project, so that you can
* reference the task by name in your build file. See here for more details
*
* If a task with the given name already exists in this project, an exception is thrown.
*
* @param name The name of the task to be created
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task task(String name) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and adds it to this project. A map of creation options can be
* passed to this method to control how the task is created. The following options are available:
*
*
*
* Option Description Default Value
*
* {@value org.gradle.api.Task#TASK_TYPE}
The class of the task to
* create. {@link org.gradle.api.DefaultTask}
*
* {@value org.gradle.api.Task#TASK_OVERWRITE}
Replace an existing
* task? false
*
*
* {@value org.gradle.api.Task#TASK_DEPENDS_ON}
A task name or set of task names which
* this task depends on []
*
* {@value org.gradle.api.Task#TASK_ACTION}
A closure or {@link Action} to add to the
* task. null
*
* {@value org.gradle.api.Task#TASK_DESCRIPTION}
A description of the task.
* null
*
* {@value org.gradle.api.Task#TASK_GROUP}
A task group which this task belongs to.
* null
*
*
*
* After the task is added to the project, it is made available as a property of the project, so that you can
* reference the task by name in your build file. See here for more details
*
* If a task with the given name already exists in this project and the override
option is not set
* to true, an exception is thrown.
*
* @param args The task creation options.
* @param name The name of the task to be created
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task task(Map args, String name) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and adds it to this project. Before the task is returned, the given
* closure is executed to configure the task. A map of creation options can be passed to this method to control how
* the task is created. See {@link #task(java.util.Map, String)} for the available options.
*
* After the task is added to the project, it is made available as a property of the project, so that you can
* reference the task by name in your build file. See here for more details
*
* If a task with the given name already exists in this project and the override
option is not set
* to true, an exception is thrown.
*
* @param args The task creation options.
* @param name The name of the task to be created
* @param configureClosure The closure to use to configure the created task.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task task(Map args, String name, Closure configureClosure);
/**
* Creates a {@link Task} with the given name and adds it to this project. Before the task is returned, the given
* closure is executed to configure the task.
After the task is added to the project, it is made
* available as a property of the project, so that you can reference the task by name in your build file. See here for more details
*
* @param name The name of the task to be created
* @param configureClosure The closure to use to configure the created task.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task task(String name, Closure configureClosure);
/**
* Returns the path of this project. The path is the fully qualified name of the project.
*
* @return The path. Never returns null.
*/
String getPath();
/**
* Returns the names of the default tasks of this project. These are used when no tasks names are provided when
* starting the build.
*
* @return The default task names. Returns an empty list if this project has no default tasks.
*/
List getDefaultTasks();
/**
* Sets the names of the default tasks of this project. These are used when no tasks names are provided when
* starting the build.
*
* @param defaultTasks The default task names.
*/
void setDefaultTasks(List defaultTasks);
/**
* Sets the names of the default tasks of this project. These are used when no tasks names are provided when
* starting the build.
*
* @param defaultTasks The default task names.
*/
void defaultTasks(String... defaultTasks);
/**
* Declares that this project has an evaluation dependency on the project with the given path.
*
* @param path The path of the project which this project depends on.
* @return The project which this project depends on.
* @throws UnknownProjectException If no project with the given path exists.
*/
Project evaluationDependsOn(String path) throws UnknownProjectException;
/**
* Declares that this project has an evaluation dependency on each of its child projects.
*
*/
void evaluationDependsOnChildren();
/**
* Locates a project by path. If the path is relative, it is interpreted relative to this project.
*
* @param path The path.
* @return The project with the given path. Returns null if no such project exists.
*/
Project findProject(String path);
/**
* Locates a project by path. If the path is relative, it is interpreted relative to this project.
*
* @param path The path.
* @return The project with the given path. Never returns null.
* @throws UnknownProjectException If no project with the given path exists.
*/
Project project(String path) throws UnknownProjectException;
/**
* Locates a project by path and configures it using the given closure. If the path is relative, it is
* interpreted relative to this project. The target project is passed to the closure as the closure's delegate.
*
* @param path The path.
* @param configureClosure The closure to use to configure the project.
* @return The project with the given path. Never returns null.
* @throws UnknownProjectException If no project with the given path exists.
*/
Project project(String path, Closure configureClosure);
/**
* Returns a map of the tasks contained in this project, and optionally its subprojects.
*
* @param recursive If true, returns the tasks of this project and its subprojects. If false, returns the tasks of
* just this project.
* @return A map from project to a set of tasks.
*/
Map> getAllTasks(boolean recursive);
/**
* Returns the set of tasks with the given name contained in this project, and optionally its subprojects.
*
* @param name The name of the task to locate.
* @param recursive If true, returns the tasks of this project and its subprojects. If false, returns the tasks of
* just this project.
* @return The set of tasks. Returns an empty set if no such tasks exist in this project.
*/
Set getTasksByName(String name, boolean recursive);
/**
* The directory containing the project build file.
*
* @return The project directory. Never returns null.
*/
File getProjectDir();
/**
* Resolves a file path relative to the project directory of this project. This method converts the supplied path
* based on its type:
*
*
*
* - A {@link CharSequence}, including {@link String} or {@link groovy.lang.GString}. Interpreted relative to the project directory. A string
* that starts with {@code file:} is treated as a file URL.
*
* - A {@link File}. If the file is an absolute file, it is returned as is. Otherwise, the file's path is
* interpreted relative to the project directory.
*
* - A {@link java.net.URI} or {@link java.net.URL}. The URL's path is interpreted as the file path. Currently, only
* {@code file:} URLs are supported.
*
*
- A {@link Closure}. The closure's return value is resolved recursively.
*
* - A {@link java.util.concurrent.Callable}. The callable's return value is resolved recursively.
*
*
*
* @param path The object to resolve as a File.
* @return The resolved file. Never returns null.
*/
File file(Object path);
/**
* Resolves a file path relative to the project directory of this project and validates it using the given
* scheme. See {@link PathValidation} for the list of possible validations.
*
* @param path An object which toString method value is interpreted as a relative path to the project directory.
* @param validation The validation to perform on the file.
* @return The resolved file. Never returns null.
* @throws InvalidUserDataException When the file does not meet the given validation constraint.
*/
File file(Object path, PathValidation validation) throws InvalidUserDataException;
/**
* Resolves a file path to a URI, relative to the project directory of this project. Evaluates the provided path
* object as described for {@link #file(Object)}, with the exception that any URI scheme is supported, not just
* 'file:' URIs.
*
* @param path The object to resolve as a URI.
* @return The resolved URI. Never returns null.
*/
URI uri(Object path);
/**
* Returns the relative path from the project directory to the given path. The given path object is (logically)
* resolved as described for {@link #file(Object)}, from which a relative path is calculated.
*
* @param path The path to convert to a relative path.
* @return The relative path. Never returns null.
*/
String relativePath(Object path);
/**
* Returns a {@link ConfigurableFileCollection} containing the given files. You can pass any of the following
* types to this method:
*
* - A {@link CharSequence}, including {@link String} or {@link groovy.lang.GString}. Interpreted relative to the project directory, as per {@link #file(Object)}. A string
* that starts with {@code file:} is treated as a file URL.
*
* - A {@link File}. Interpreted relative to the project directory, as per {@link #file(Object)}.
*
* - A {@link java.net.URI} or {@link java.net.URL}. The URL's path is interpreted as a file path. Currently, only
* {@code file:} URLs are supported.
*
*
- A {@link java.util.Collection}, {@link Iterable}, or an array. May contain any of the types listed here. The elements of the collection
* are recursively converted to files.
*
* - A {@link org.gradle.api.file.FileCollection}. The contents of the collection are included in the returned
* collection.
*
* - A {@link java.util.concurrent.Callable}. The {@code call()} method may return any of the types listed here.
* The return value of the {@code call()} method is recursively converted to files. A {@code null} return value is
* treated as an empty collection.
*
* - A Closure. May return any of the types listed here. The return value of the closure is recursively converted
* to files. A {@code null} return value is treated as an empty collection.
*
* - A {@link Task}. Converted to the task's output files.
*
* - A {@link org.gradle.api.tasks.TaskOutputs}. Converted to the output files the related task.
*
* - Anything else is treated as a failure.
*
*
*
* The returned file collection is lazy, so that the paths are evaluated only when the contents of the file
* collection are queried. The file collection is also live, so that it evaluates the above each time the contents
* of the collection is queried.
*
* The returned file collection maintains the iteration order of the supplied paths.
*
* @param paths The paths to the files. May be empty.
* @return The file collection. Never returns null.
*/
ConfigurableFileCollection files(Object... paths);
/**
* Creates a new {@code ConfigurableFileCollection} using the given paths. The paths are evaluated as per {@link
* #files(Object...)}. The file collection is configured using the given closure. The file collection is passed to
* the closure as its delegate. Example:
*
* files "$buildDir/classes" {
* builtBy 'compile'
* }
*
* The returned file collection is lazy, so that the paths are evaluated only when the contents of the file
* collection are queried. The file collection is also live, so that it evaluates the above each time the contents
* of the collection is queried.
*
* @param paths The contents of the file collection. Evaluated as per {@link #files(Object...)}.
* @param configureClosure The closure to use to configure the file collection.
* @return the configured file tree. Never returns null.
*/
ConfigurableFileCollection files(Object paths, Closure configureClosure);
/**
* Creates a new {@code ConfigurableFileTree} using the given base directory. The given baseDir path is evaluated
* as per {@link #file(Object)}.
*
* The returned file tree is lazy, so that it scans for files only when the contents of the file tree are
* queried. The file tree is also live, so that it scans for files each time the contents of the file tree are
* queried.
*
*
* def myTree = fileTree("src")
* myTree.include "**/*.java"
* myTree.builtBy "someTask"
*
* task copy(type: Copy) {
* from myTree
* }
*
*
* @param baseDir The base directory of the file tree. Evaluated as per {@link #file(Object)}.
* @return the file tree. Never returns null.
*/
ConfigurableFileTree fileTree(Object baseDir);
/**
* Creates a new {@code ConfigurableFileTree} using the given base directory. The given baseDir path is evaluated
* as per {@link #file(Object)}. The closure will be used to configure the new file tree.
* The file tree is passed to the closure as its delegate. Example:
*
*
* def myTree = fileTree('src') {
* exclude '**/.data/**'
* builtBy 'someTask'
* }
*
* task copy(type: Copy) {
* from myTree
* }
*
*
* The returned file tree is lazy, so that it scans for files only when the contents of the file tree are
* queried. The file tree is also live, so that it scans for files each time the contents of the file tree are
* queried.
*
* @param baseDir The base directory of the file tree. Evaluated as per {@link #file(Object)}.
* @param configureClosure Closure to configure the {@code ConfigurableFileTree} object.
* @return the configured file tree. Never returns null.
*/
ConfigurableFileTree fileTree(Object baseDir, Closure configureClosure);
/**
* Creates a new {@code ConfigurableFileTree} using the provided map of arguments. The map will be applied as
* properties on the new file tree. Example:
*
*
* def myTree = fileTree(dir:'src', excludes:['**/ignore/**', '**/.data/**'])
*
* task copy(type: Copy) {
* from myTree
* }
*
*
* The returned file tree is lazy, so that it scans for files only when the contents of the file tree are
* queried. The file tree is also live, so that it scans for files each time the contents of the file tree are
* queried.
*
* @param args map of property assignments to {@code ConfigurableFileTree} object
* @return the configured file tree. Never returns null.
*/
ConfigurableFileTree fileTree(Map args);
/**
* Creates a new {@code FileTree} which contains the contents of the given ZIP file. The given zipPath path is
* evaluated as per {@link #file(Object)}. You can combine this method with the {@link #copy(groovy.lang.Closure)}
* method to unzip a ZIP file.
*
* The returned file tree is lazy, so that it scans for files only when the contents of the file tree are
* queried. The file tree is also live, so that it scans for files each time the contents of the file tree are
* queried.
*
* @param zipPath The ZIP file. Evaluated as per {@link #file(Object)}.
* @return the file tree. Never returns null.
*/
FileTree zipTree(Object zipPath);
/**
* Creates a new {@code FileTree} which contains the contents of the given TAR file. The given tarPath path can be:
*
* - an instance of {@link org.gradle.api.resources.Resource}
* - any other object is evaluated as per {@link #file(Object)}
*
*
* The returned file tree is lazy, so that it scans for files only when the contents of the file tree are
* queried. The file tree is also live, so that it scans for files each time the contents of the file tree are
* queried.
*
* Unless custom implementation of resources is passed, the tar tree attempts to guess the compression based on the file extension.
*
* You can combine this method with the {@link #copy(groovy.lang.Closure)}
* method to untar a TAR file:
*
*
* task untar(type: Copy) {
* from tarTree('someCompressedTar.gzip')
*
* //tar tree attempts to guess the compression based on the file extension
* //however if you must specify the compression explicitly you can:
* from tarTree(resources.gzip('someTar.ext'))
*
* //in case you work with unconventionally compressed tars
* //you can provide your own implementation of a ReadableResource:
* //from tarTree(yourOwnResource as ReadableResource)
*
* into 'dest'
* }
*
*
* @param tarPath The TAR file or an instance of {@link org.gradle.api.resources.Resource}.
* @return the file tree. Never returns null.
*/
FileTree tarTree(Object tarPath);
/**
* Creates a directory and returns a file pointing to it.
*
* @param path The path for the directory to be created. Evaluated as per {@link #file(Object)}.
* @return the created directory
* @throws org.gradle.api.InvalidUserDataException If the path points to an existing file.
*/
File mkdir(Object path);
/**
* Deletes files and directories.
*
* This will not follow symlinks. If you need to follow symlinks too use {@link #delete(Action)}.
*
* @param paths Any type of object accepted by {@link org.gradle.api.Project#files(Object...)}
* @return true if anything got deleted, false otherwise
*/
boolean delete(Object... paths);
/**
* Deletes the specified files. The given action is used to configure a {@link DeleteSpec}, which is then used to
* delete the files.
*
Example:
*
* project.delete {
* delete 'somefile'
* followSymlinks = true
* }
*
*
* @param action Action to configure the DeleteSpec
* @return {@link WorkResult} that can be used to check if delete did any work.
*/
WorkResult delete(Action super DeleteSpec> action);
/**
* Executes a Java main class. The closure configures a {@link org.gradle.process.JavaExecSpec}.
*
* @param closure The closure for configuring the execution.
* @return the result of the execution
*/
ExecResult javaexec(Closure closure);
/**
* Executes an external Java process.
*
* The given action configures a {@link org.gradle.process.JavaExecSpec}, which is used to launch the process.
* This method blocks until the process terminates, with its result being returned.
*
* @param action The action for configuring the execution.
* @return the result of the execution
*/
ExecResult javaexec(Action super JavaExecSpec> action);
/**
* Executes an external command. The closure configures a {@link org.gradle.process.ExecSpec}.
*
* @param closure The closure for configuring the execution.
* @return the result of the execution
*/
ExecResult exec(Closure closure);
/**
* Executes an external command.
*
* The given action configures a {@link org.gradle.process.ExecSpec}, which is used to launch the process.
* This method blocks until the process terminates, with its result being returned.
*
* @param action The action for configuring the execution.
* @return the result of the execution
*/
ExecResult exec(Action super ExecSpec> action);
/**
*
Converts a name to an absolute project path, resolving names relative to this project.
*
* @param path The path to convert.
* @return The absolute path.
*/
String absoluteProjectPath(String path);
/**
* Converts a name to a project path relative to this project.
*
* @param path The path to convert.
* @return The relative path.
*/
String relativeProjectPath(String path);
/**
* Returns the AntBuilder
for this project. You can use this in your build file to execute ant
* tasks. See example below.
*
* task printChecksum {
* doLast {
* ant {
* //using ant checksum task to store the file checksum in the checksumOut ant property
* checksum(property: 'checksumOut', file: 'someFile.txt')
*
* //we can refer to the ant property created by checksum task:
* println "The checksum is: " + checksumOut
* }
*
* //we can refer to the ant property later as well:
* println "I just love to print checksums: " + ant.checksumOut
* }
* }
*
*
* Consider following example of ant target:
*
* <target name='printChecksum'>
* <checksum property='checksumOut'>
* <fileset dir='.'>
* <include name='agile.txt'/>
* </fileset>
* </checksum>
* <echo>The checksum is: ${checksumOut}</echo>
* </target>
*
*
* Here's how it would look like in gradle. Observe how the ant XML is represented in groovy by the ant builder
*
* task printChecksum {
* doLast {
* ant {
* checksum(property: 'checksumOut') {
* fileset(dir: '.') {
* include name: 'agile1.txt'
* }
* }
* }
* logger.lifecycle("The checksum is $ant.checksumOut")
* }
* }
*
*
* @return The AntBuilder
for this project. Never returns null.
*/
AntBuilder getAnt();
/**
* Creates an additional AntBuilder
for this project. You can use this in your build file to execute
* ant tasks.
*
* @return Creates an AntBuilder
for this project. Never returns null.
* @see #getAnt()
*/
AntBuilder createAntBuilder();
/**
* Executes the given closure against the AntBuilder
for this project. You can use this in your
* build file to execute ant tasks. The AntBuild
is passed to the closure as the closure's
* delegate. See example in javadoc for {@link #getAnt()}
*
* @param configureClosure The closure to execute against the AntBuilder
.
* @return The AntBuilder
. Never returns null.
*/
AntBuilder ant(Closure configureClosure);
/**
* Returns the configurations of this project.
*
* Examples:
See docs for {@link ConfigurationContainer}
*
* @return The configuration of this project.
*/
ConfigurationContainer getConfigurations();
/**
* Configures the dependency configurations for this project.
*
*
This method executes the given closure against the {@link ConfigurationContainer}
* for this project. The {@link ConfigurationContainer} is passed to the closure as the closure's delegate.
*
*
Examples:
See docs for {@link ConfigurationContainer}
*
* @param configureClosure the closure to use to configure the dependency configurations.
*/
void configurations(Closure configureClosure);
/**
* Returns a handler for assigning artifacts produced by the project to configurations.
* Examples:
See docs for {@link ArtifactHandler}
*/
ArtifactHandler getArtifacts();
/**
* Configures the published artifacts for this project.
*
*
This method executes the given closure against the {@link ArtifactHandler} for this project. The {@link
* ArtifactHandler} is passed to the closure as the closure's delegate.
*
*
Example:
*
* configurations {
* //declaring new configuration that will be used to associate with artifacts
* schema
* }
*
* task schemaJar(type: Jar) {
* //some imaginary task that creates a jar artifact with the schema
* }
*
* //associating the task that produces the artifact with the configuration
* artifacts {
* //configuration name and the task:
* schema schemaJar
* }
*
*
* @param configureClosure the closure to use to configure the published artifacts.
*/
void artifacts(Closure configureClosure);
/**
* Returns the {@link Convention} for this project.
You can access this property in your build file
* using convention
. You can also can also access the properties and methods of the convention object
* as if they were properties and methods of this project. See here for more details
*
* @return The Convention
. Never returns null.
*/
Convention getConvention();
/**
* Compares the nesting level of this project with another project of the multi-project hierarchy.
*
* @param otherProject The project to compare the nesting level with.
* @return a negative integer, zero, or a positive integer as this project has a nesting level less than, equal to,
* or greater than the specified object.
* @see #getDepth()
*/
int depthCompare(Project otherProject);
/**
* Returns the nesting level of a project in a multi-project hierarchy. For single project builds this is always
* 0. In a multi-project hierarchy 0 is returned for the root project.
*/
int getDepth();
/**
* Returns the tasks of this project.
*
* @return the tasks of this project.
*/
TaskContainer getTasks();
/**
* Configures the sub-projects of this project
*
* This method executes the given {@link Action} against the sub-projects of this project.
*
* @param action The action to execute.
*/
void subprojects(Action super Project> action);
/**
* Configures the sub-projects of this project.
*
* This method executes the given closure against each of the sub-projects of this project. The target {@link
* Project} is passed to the closure as the closure's delegate.
*
* @param configureClosure The closure to execute.
*/
void subprojects(Closure configureClosure);
/**
* Configures this project and each of its sub-projects.
*
* This method executes the given {@link Action} against this project and each of its sub-projects.
*
* @param action The action to execute.
*/
void allprojects(Action super Project> action);
/**
* Configures this project and each of its sub-projects.
*
* This method executes the given closure against this project and its sub-projects. The target {@link Project}
* is passed to the closure as the closure's delegate.
*
* @param configureClosure The closure to execute.
*/
void allprojects(Closure configureClosure);
/**
* Adds an action to execute immediately before this project is evaluated.
*
* @param action the action to execute.
*/
void beforeEvaluate(Action super Project> action);
/**
* Adds an action to execute immediately after this project is evaluated.
*
* @param action the action to execute.
*/
void afterEvaluate(Action super Project> action);
/**
* Adds a closure to be called immediately before this project is evaluated. The project is passed to the closure
* as a parameter.
*
* @param closure The closure to call.
*/
void beforeEvaluate(Closure closure);
/**
* Adds a closure to be called immediately after this project has been evaluated. The project is passed to the
* closure as a parameter. Such a listener gets notified when the build file belonging to this project has been
* executed. A parent project may for example add such a listener to its child project. Such a listener can further
* configure those child projects based on the state of the child projects after their build files have been
* run.
*
* @param closure The closure to call.
*/
void afterEvaluate(Closure closure);
/**
* Determines if this project has the given property. See here for details of the
* properties which are available for a project.
*
* @param propertyName The name of the property to locate.
* @return True if this project has the given property, false otherwise.
*/
boolean hasProperty(String propertyName);
/**
* Returns the properties of this project. See here for details of the properties which
* are available for a project.
*
* @return A map from property name to value.
*/
Map getProperties();
/**
* Returns the value of the given property. This method locates a property as follows:
*
*
*
* - If this project object has a property with the given name, return the value of the property.
*
* - If this project has an extension with the given name, return the extension.
*
* - If this project's convention object has a property with the given name, return the value of the
* property.
*
* - If this project has an extra property with the given name, return the value of the property.
*
* - If this project has a task with the given name, return the task.
*
* - Search up through this project's ancestor projects for a convention property or extra property with the
* given name.
*
* - If not found, a {@link MissingPropertyException} is thrown.
*
*
*
* @param propertyName The name of the property.
* @return The value of the property, possibly null.
* @throws MissingPropertyException When the given property is unknown.
* @see Project#findProperty(String)
*/
Object property(String propertyName) throws MissingPropertyException;
/**
* Returns the value of the given property or null if not found.
* This method locates a property as follows:
*
*
*
* - If this project object has a property with the given name, return the value of the property.
*
* - If this project has an extension with the given name, return the extension.
*
* - If this project's convention object has a property with the given name, return the value of the
* property.
*
* - If this project has an extra property with the given name, return the value of the property.
*
* - If this project has a task with the given name, return the task.
*
* - Search up through this project's ancestor projects for a convention property or extra property with the
* given name.
*
* - If not found, null value is returned.
*
*
*
* @param propertyName The name of the property.
* @return The value of the property, possibly null or null if not found.
* @see Project#property(String)
*/
@Incubating
Object findProperty(String propertyName);
/**
* Returns the logger for this project. You can use this in your build file to write log messages.
*
* @return The logger. Never returns null.
*/
Logger getLogger();
/**
* Returns the {@link org.gradle.api.invocation.Gradle} invocation which this project belongs to.
*
* @return The Gradle object. Never returns null.
*/
Gradle getGradle();
/**
* Returns the {@link org.gradle.api.logging.LoggingManager} which can be used to control the logging level and
* standard output/error capture for this project's build script. By default, System.out is redirected to the Gradle
* logging system at the QUIET log level, and System.err is redirected at the ERROR log level.
*
* @return the LoggingManager. Never returns null.
*/
LoggingManager getLogging();
/**
* Configures an object via a closure, with the closure's delegate set to the supplied object. This way you don't
* have to specify the context of a configuration statement multiple times.
Instead of:
*
* MyType myType = new MyType()
* myType.doThis()
* myType.doThat()
*
* you can do:
*
* MyType myType = configure(new MyType()) {
* doThis()
* doThat()
* }
*
*
* The object being configured is also passed to the closure as a parameter, so you can access it explicitly if
* required:
*
* configure(someObj) { obj -> obj.doThis() }
*
*
* @param object The object to configure
* @param configureClosure The closure with configure statements
* @return The configured object
*/
Object configure(Object object, Closure configureClosure);
/**
* Configures a collection of objects via a closure. This is equivalent to calling {@link #configure(Object,
* groovy.lang.Closure)} for each of the given objects.
*
* @param objects The objects to configure
* @param configureClosure The closure with configure statements
* @return The configured objects.
*/
Iterable> configure(Iterable> objects, Closure configureClosure);
/**
* Configures a collection of objects via an action.
*
* @param objects The objects to configure
* @param configureAction The action to apply to each object
* @return The configured objects.
*/
Iterable configure(Iterable objects, Action super T> configureAction);
/**
* Returns a handler to create repositories which are used for retrieving dependencies and uploading artifacts
* produced by the project.
*
* @return the repository handler. Never returns null.
*/
RepositoryHandler getRepositories();
/**
* Configures the repositories for this project.
*
*
This method executes the given closure against the {@link RepositoryHandler} for this project. The {@link
* RepositoryHandler} is passed to the closure as the closure's delegate.
*
* @param configureClosure the closure to use to configure the repositories.
*/
void repositories(Closure configureClosure);
/**
* Returns the dependency handler of this project. The returned dependency handler instance can be used for adding
* new dependencies. For accessing already declared dependencies, the configurations can be used.
*
*
Examples:
* See docs for {@link DependencyHandler}
*
* @return the dependency handler. Never returns null.
* @see #getConfigurations()
*/
DependencyHandler getDependencies();
/**
* Configures the dependencies for this project.
*
*
This method executes the given closure against the {@link DependencyHandler} for this project. The {@link
* DependencyHandler} is passed to the closure as the closure's delegate.
*
*
Examples:
* See docs for {@link DependencyHandler}
*
* @param configureClosure the closure to use to configure the dependencies.
*/
void dependencies(Closure configureClosure);
/**
* Returns the build script handler for this project. You can use this handler to query details about the build
* script for this project, and manage the classpath used to compile and execute the project's build script.
*
* @return the classpath handler. Never returns null.
*/
ScriptHandler getBuildscript();
/**
* Configures the build script classpath for this project.
*
*
The given closure is executed against this project's {@link ScriptHandler}. The {@link ScriptHandler} is
* passed to the closure as the closure's delegate.
*
* @param configureClosure the closure to use to configure the build script classpath.
*/
void buildscript(Closure configureClosure);
/**
* Copies the specified files. The given closure is used to configure a {@link CopySpec}, which is then used to
* copy the files. Example:
*
* copy {
* from configurations.runtime
* into 'build/deploy/lib'
* }
*
* Note that CopySpecs can be nested:
*
* copy {
* into 'build/webroot'
* exclude '**/.svn/**'
* from('src/main/webapp') {
* include '**/*.jsp'
* filter(ReplaceTokens, tokens:[copyright:'2009', version:'2.3.1'])
* }
* from('src/main/js') {
* include '**/*.js'
* }
* }
*
*
* @param closure Closure to configure the CopySpec
* @return {@link WorkResult} that can be used to check if the copy did any work.
*/
WorkResult copy(Closure closure);
/**
* Creates a {@link CopySpec} which can later be used to copy files or create an archive. The given closure is used
* to configure the {@link CopySpec} before it is returned by this method.
*
*
* def baseSpec = copySpec {
* from "source"
* include "**/*.java"
* }
*
* task copy(type: Copy) {
* into "target"
* with baseSpec
* }
*
*
* @param closure Closure to configure the CopySpec
* @return The CopySpec
*/
CopySpec copySpec(Closure closure);
/**
* Copies the specified files. The given action is used to configure a {@link CopySpec}, which is then used to
* copy the files.
* @see #copy(Closure)
* @param action Action to configure the CopySpec
* @return {@link WorkResult} that can be used to check if the copy did any work.
*/
WorkResult copy(Action super CopySpec> action);
/**
* Creates a {@link CopySpec} which can later be used to copy files or create an archive. The given action is used
* to configure the {@link CopySpec} before it is returned by this method.
*
* @see #copySpec(Closure)
* @param action Action to configure the CopySpec
* @return The CopySpec
*/
CopySpec copySpec(Action super CopySpec> action);
/**
* Creates a {@link CopySpec} which can later be used to copy files or create an archive.
*
* @return a newly created copy spec
*/
CopySpec copySpec();
/**
* Returns the evaluation state of this project. You can use this to access information about the evaluation of this
* project, such as whether it has failed.
*
* @return the project state. Never returns null.
*/
ProjectState getState();
/**
* Creates a container for managing named objects of the specified type. The specified type must have a public constructor which takes the name as a String parameter.
*
*
All objects MUST expose their name as a bean property named "name". The name must be constant for the life of the object.
*
* @param type The type of objects for the container to contain.
* @param The type of objects for the container to contain.
* @return The container.
*/
NamedDomainObjectContainer container(Class type);
/**
* Creates a container for managing named objects of the specified type. The given factory is used to create object instances.
*
* All objects MUST expose their name as a bean property named "name". The name must be constant for the life of the object.
*
* @param type The type of objects for the container to contain.
* @param factory The factory to use to create object instances.
* @param The type of objects for the container to contain.
* @return The container.
*/
NamedDomainObjectContainer container(Class type, NamedDomainObjectFactory factory);
/**
* Creates a container for managing named objects of the specified type. The given closure is used to create object instances. The name of the instance to be created is passed as a parameter to
* the closure.
*
* All objects MUST expose their name as a bean property named "name". The name must be constant for the life of the object.
*
* @param type The type of objects for the container to contain.
* @param factoryClosure The closure to use to create object instances.
* @param The type of objects for the container to contain.
* @return The container.
*/
NamedDomainObjectContainer container(Class type, Closure factoryClosure);
/**
* Allows adding DSL extensions to the project. Useful for plugin authors.
*
* @return Returned instance allows adding DSL extensions to the project
*/
ExtensionContainer getExtensions();
/**
* Provides access to resource-specific utility methods, for example factory methods that create various resources.
*
* @return Returned instance contains various resource-specific utility methods.
*/
ResourceHandler getResources();
/**
* Returns the software components produced by this project.
*
* @return The components for this project.
*/
@Incubating
SoftwareComponentContainer getComponents();
}