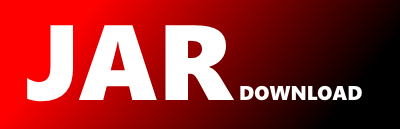
org.gradle.apiModuleDependency Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.artifacts;
import org.gradle.api.Action;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import java.util.Map;
import java.util.Set;
import static groovy.lang.Closure.DELEGATE_FIRST;
/**
* A {@code ModuleDependency} is a {@link org.gradle.api.artifacts.Dependency} on a module outside the current
* build.
*
* For examples on configuring the exclude rules please refer to {@link #exclude(java.util.Map)}.
*/
public interface ModuleDependency extends Dependency {
/**
* Adds an exclude rule to exclude transitive dependencies of this dependency.
*
* Excluding a particular transitive dependency does not guarantee that it does not show up
* in the dependencies of a given configuration.
* For example, some other dependency, which does not have any exclude rules,
* might pull in exactly the same transitive dependency.
* To guarantee that the transitive dependency is excluded from the entire configuration
* please use per-configuration exclude rules: {@link Configuration#getExcludeRules()}.
* In fact, in majority of cases the actual intention of configuring per-dependency exclusions
* is really excluding a dependency from the entire configuration (or classpath).
*
* If your intention is to exclude a particular transitive dependency
* because you don't like the version it pulls in to the configuration
* then consider using forced versions' feature: {@link ResolutionStrategy#force(Object...)}.
*
*
* apply plugin: 'java' //so that I can declare 'compile' dependencies
*
* dependencies {
* compile('org.hibernate:hibernate:3.1') {
* //excluding a particular transitive dependency:
* exclude module: 'cglib' //by artifact name
* exclude group: 'org.jmock' //by group
* exclude group: 'org.unwanted', module: 'iAmBuggy' //by both name and group
* }
* }
*
*
* @param excludeProperties the properties to define the exclude rule.
* @return this
*/
ModuleDependency exclude(Map excludeProperties);
/**
* Returns the exclude rules for this dependency.
*
* @see #exclude(java.util.Map)
*/
Set getExcludeRules();
/**
* Returns the artifacts belonging to this dependency.
*
* @see #addArtifact(DependencyArtifact)
*/
Set getArtifacts();
/**
* Adds an artifact to this dependency.
*
* If no artifact is added to a dependency, an implicit default artifact is used. This default artifact has the
* same name as the module and its type and extension is jar. If at least one artifact is explicitly added,
* the implicit default artifact won't be used any longer.
*
* @return this
*/
ModuleDependency addArtifact(DependencyArtifact artifact);
/**
* Adds an artifact to this dependency. The given closure is passed a {@link
* org.gradle.api.artifacts.DependencyArtifact} instance, which it can configure.
*
* If no artifact is added to a dependency, an implicit default artifact is used. This default artifact has the
* same name as the module and its type and extension is jar. If at least one artifact is explicitly added,
* the implicit default artifact won't be used any longer.
*
* @return this
*/
DependencyArtifact artifact(@DelegatesTo(value = DependencyArtifact.class, strategy = DELEGATE_FIRST) Closure configureClosure);
/**
* Adds an artifact to this dependency. The given action is passed a {@link
* org.gradle.api.artifacts.DependencyArtifact} instance, which it can configure.
*
* If no artifact is added to a dependency, an implicit default artifact is used. This default artifact has the
* same name as the module and its type and extension is jar. If at least one artifact is explicitly added,
* the implicit default artifact won't be used any longer.
*
* @return this
*
* @since 3.1
*/
DependencyArtifact artifact(Action super DependencyArtifact> configureAction);
/**
* Returns whether this dependency should be resolved including or excluding its transitive dependencies.
*
* @see #setTransitive(boolean)
*/
boolean isTransitive();
/**
* Sets whether this dependency should be resolved including or excluding its transitive dependencies. The artifacts
* belonging to this dependency might themselves have dependencies on other artifacts. The latter are called
* transitive dependencies.
*
* @param transitive Whether transitive dependencies should be resolved.
* @return this
*/
ModuleDependency setTransitive(boolean transitive);
/**
* Returns the configuration of this dependency module (not the configurations this dependency belongs too). Never
* returns null. The default value for the configuration is {@link #DEFAULT_CONFIGURATION}. A dependency source
* might have multiple configurations. Every configuration represents a different set of artifacts and dependencies
* for this dependency module.
*/
String getConfiguration();
/**
* {@inheritDoc}
*/
ModuleDependency copy();
}