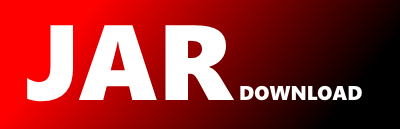
org.gradle.api.tasks.TaskContainer Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.tasks;
import groovy.lang.Closure;
import org.gradle.api.*;
import org.gradle.internal.HasInternalProtocol;
import java.util.Map;
/**
* A {@code TaskContainer} is responsible for managing a set of {@link Task} instances.
*
* You can obtain a {@code TaskContainer} instance by calling {@link org.gradle.api.Project#getTasks()}, or using the
* {@code tasks} property in your build script.
*/
@HasInternalProtocol
public interface TaskContainer extends TaskCollection, PolymorphicDomainObjectContainer {
/**
* Locates a task by path. You can supply a task name, a relative path, or an absolute path. Relative paths are
* interpreted relative to the project for this container. This method returns null if no task with the given path
* exists.
*
* @param path the path of the task to be returned
* @return The task. Returns null if so such task exists.
*/
Task findByPath(String path);
/**
* Locates a task by path. You can supply a task name, a relative path, or an absolute path. Relative paths are
* interpreted relative to the project for this container. This method throws an exception if no task with the given
* path exists.
*
* @param path the path of the task to be returned
* @return The task. Never returns null
* @throws UnknownTaskException If no task with the given path exists.
*/
Task getByPath(String path) throws UnknownTaskException;
/**
* Creates a {@link Task} and adds it to this container. A map of creation options can be passed to this method
* to control how the task is created. The following options are available:
*
*
*
* Option Description Default Value
*
* {@value org.gradle.api.Task#TASK_NAME}
The name of the task to create. None.
* Must be specified.
*
* {@value org.gradle.api.Task#TASK_TYPE}
The class of the task to
* create. {@link org.gradle.api.DefaultTask}
*
* {@value org.gradle.api.Task#TASK_ACTION}
The closure or {@link Action} to
* execute when the task executes. See {@link Task#doFirst(Action)}. null
*
* {@value org.gradle.api.Task#TASK_OVERWRITE}
Replace an existing
* task? false
*
* {@value org.gradle.api.Task#TASK_DEPENDS_ON}
The dependencies of the task. See here for more details. []
*
* {@value org.gradle.api.Task#TASK_GROUP}
The group of the task. null
*
*
* {@value org.gradle.api.Task#TASK_DESCRIPTION}
The description of the task.
* null
*
*
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* If a task with the given name already exists in this container and the {@value org.gradle.api.Task#TASK_OVERWRITE}
* option is not set to true, an exception is thrown.
*
* @param options The task creation options.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task create(Map options) throws InvalidUserDataException;
/**
* Creates a {@link Task} adds it to this container. A map of creation options can be passed to this method to
* control how the task is created. See {@link #create(java.util.Map)} for the list of options available. The given
* closure is used to configure the task before it is returned by this method.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param options The task creation options.
* @param configureClosure The closure to use to configure the task.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task create(Map options, Closure configureClosure) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name adds it to this container. The given closure is used to configure
* the task before it is returned by this method.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created
* @param configureClosure The closure to use to configure the task.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task create(String name, Closure configureClosure) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and adds it to this container.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task create(String name) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and type, and adds it to this container.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created.
* @param type The type of task to create.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
T create(String name, Class type) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and type, configures it with the given action, and adds it to this container.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created.
* @param type The type of task to create.
* @param configuration The action to configure the task with.
* @return The newly created task object.
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
T create(String name, Class type, Action super T> configuration) throws InvalidUserDataException;
/**
* Creates a {@link Task} with the given name and adds it to this container, replacing any existing task with the
* same name.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
Task replace(String name);
/**
* Creates a {@link Task} with the given name and type, and adds it to this container, replacing any existing
* task of the same name.
*
* After the task is added, it is made available as a property of the project, so that you can reference the task
* by name in your build file. See here for more details.
*
* @param name The name of the task to be created.
* @param type The type of task to create.
* @return The newly created task object
* @throws InvalidUserDataException If a task with the given name already exists in this project.
*/
T replace(String name, Class type);
}