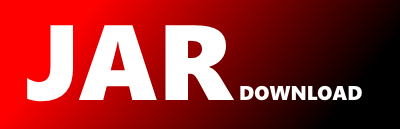
org.gradle.integtests.tooling.fixture.ToolingApiSpecification.groovy Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.integtests.tooling.fixture
import groovy.transform.stc.ClosureParams
import groovy.transform.stc.SimpleType
import org.gradle.test.fixtures.file.TestFile
import org.gradle.tooling.ProjectConnection
import org.gradle.util.GradleVersion
import org.junit.runner.RunWith
@ToolingApiVersion('>=2.0')
@TargetGradleVersion('>=1.2')
@RunWith(ToolingApiCompatibilitySuiteRunner)
abstract class ToolingApiSpecification extends AbstractToolingApiSpecification {
public T withConnection(@DelegatesTo(ProjectConnection) @ClosureParams(value = SimpleType, options = ["org.gradle.tooling.ProjectConnection"]) Closure cl) {
toolingApi.withConnection(cl)
}
public ConfigurableOperation withModel(Class modelType, Closure cl = {}) {
withConnection {
def model = it.model(modelType)
cl(model)
new ConfigurableOperation(model).buildModel()
}
}
public ConfigurableOperation withBuild(Closure cl = {}) {
withConnection {
def build = it.newBuild()
cl(build)
def out = new ConfigurableOperation(build)
build.run()
out
}
}
TestFile getProjectDir() {
temporaryFolder.testDirectory
}
TestFile getBuildFile() {
file("build.gradle")
}
TestFile getSettingsFile() {
file("settings.gradle")
}
TestFile file(Object... path) {
projectDir.file(path)
}
/**
* Returns the set of implicit task names expected for a non-root project for the target Gradle version.
*/
Set getImplicitTasks() {
if (GradleVersion.version(targetDist.version.baseVersion.version) >= GradleVersion.version("2.10")) {
return ['buildEnvironment', 'components', 'dependencies', 'dependencyInsight', 'help', 'projects', 'properties', 'tasks', 'model']
} else if (GradleVersion.version(targetDist.version.baseVersion.version) >= GradleVersion.version("2.4")) {
return ['components', 'dependencies', 'dependencyInsight', 'help', 'projects', 'properties', 'tasks', 'model']
} else if (GradleVersion.version(targetDist.version.baseVersion.version) >= GradleVersion.version("2.1")) {
return ['components', 'dependencies', 'dependencyInsight', 'help', 'projects', 'properties', 'tasks']
} else {
return ['dependencies', 'dependencyInsight', 'help', 'projects', 'properties', 'tasks']
}
}
/**
* Returns the set of implicit selector names expected for a non-root project for the target Gradle version.
*
* Note that in some versions the handling of implicit selectors was broken, so this method may return a different value
* to {@link #getImplicitTasks()}.
*/
Set getImplicitSelectors() {
if (GradleVersion.version(targetDist.version.baseVersion.version) <= GradleVersion.version("2.0")) {
// Implicit tasks were ignored
return []
}
return getImplicitTasks()
}
/**
* Returns the set of implicit task names expected for a root project for the target Gradle version.
*/
Set getRootProjectImplicitTasks() {
def targetVersion = GradleVersion.version(targetDist.version.baseVersion.version)
if (targetVersion == GradleVersion.version("1.6")) {
return implicitTasks + ['setupBuild']
}
return implicitTasks + ['init', 'wrapper']
}
/**
* Returns the set of implicit selector names expected for a root project for the target Gradle version.
*
* Note that in some versions the handling of implicit selectors was broken, so this method may return a different value
* to {@link #getRootProjectImplicitTasks()}.
*/
Set getRootProjectImplicitSelectors() {
def targetVersion = GradleVersion.version(targetDist.version.baseVersion.version)
if (targetVersion == GradleVersion.version("1.6")) {
// Implicit tasks were ignored, and setupBuild was added as a regular task
return ['setupBuild']
}
if (targetVersion <= GradleVersion.version("2.0")) {
// Implicit tasks were ignored
return []
}
return rootProjectImplicitTasks
}
/**
* Returns the set of implicit tasks returned by GradleProject.getTasks()
*
* Note that in some versions the handling of implicit tasks was broken, so this method may return a different value
* to {@link #getRootProjectImplicitTasks()}.
*/
Set getRootProjectImplicitTasksForGradleProjectModel() {
def targetVersion = GradleVersion.version(targetDist.version.baseVersion.version)
if (targetVersion == GradleVersion.version("1.6")) {
// Implicit tasks were ignored, and setupBuild was added as a regular task
return ['setupBuild']
}
targetVersion < GradleVersion.version("2.3") ? [] : rootProjectImplicitTasks
}
public T loadToolingModel(Class modelClass) {
withConnection { connection -> connection.getModel(modelClass) }
}
}