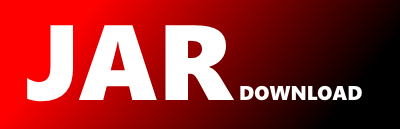
org.gradle.internal.serialize.BaseSerializerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.internal.serialize;
import com.google.common.collect.ImmutableMap;
import java.io.File;
import java.util.Map;
public class BaseSerializerFactory {
public static final Serializer STRING_SERIALIZER = new StringSerializer();
public static final Serializer BOOLEAN_SERIALIZER = new BooleanSerializer();
public static final Serializer LONG_SERIALIZER = new LongSerializer();
public static final Serializer FILE_SERIALIZER = new FileSerializer();
public static final Serializer BYTE_ARRAY_SERIALIZER = new ByteArraySerializer();
public static final Serializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy