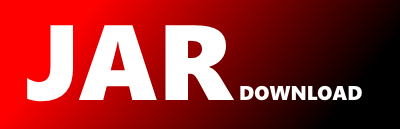
org.gradle.launcher.daemon.AndroidDexingSoakTest.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.launcher.daemon
import org.gradle.api.internal.cache.HeapProportionalCacheSizer
import org.gradle.integtests.fixtures.daemon.DaemonIntegrationSpec
import org.gradle.soak.categories.SoakTest
import org.gradle.util.Requires
import org.gradle.util.TestPrecondition
import org.junit.experimental.categories.Category
@Category(SoakTest)
@Requires(TestPrecondition.NOT_WINDOWS)
class AndroidDexingSoakTest extends DaemonIntegrationSpec {
int buildCount
int maxRatio
def "dexing remains performant with cache-reserved space"() {
given:
simpleAndroidApp()
buildFile << """
configurations.all {
exclude group: 'com.android.support'
}
dependencies {
compile "joda-time:joda-time:2.2"
}
import com.google.common.cache.CacheBuilder
import com.google.common.cache.Cache
import org.gradle.api.internal.cache.HeapProportionalCacheSizer
// create a heap-proportional cache that we can fill up over multiple builds
class State {
static Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy