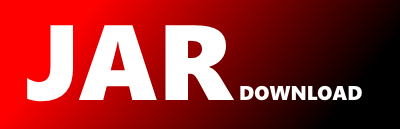
org.gradle.plugins.ide.eclipse.model.EclipseClasspath Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.plugins.ide.eclipse.model;
import com.google.common.base.Preconditions;
import groovy.lang.Closure;
import org.gradle.api.Action;
import org.gradle.api.artifacts.Configuration;
import org.gradle.api.tasks.SourceSet;
import org.gradle.plugins.ide.api.XmlFileContentMerger;
import org.gradle.plugins.ide.eclipse.model.internal.ClasspathFactory;
import org.gradle.plugins.ide.eclipse.model.internal.FileReferenceFactory;
import org.gradle.plugins.ide.internal.resolver.UnresolvedDependenciesLogger;
import org.gradle.util.ConfigureUtil;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The build path settings for the generated Eclipse project. Used by the
* {@link org.gradle.plugins.ide.eclipse.GenerateEclipseClasspath} task to generate an Eclipse .classpath file.
*
* The following example demonstrates the various configuration options.
* Keep in mind that all properties have sensible defaults; only configure them explicitly
* if the defaults don't match your needs.
*
*
* apply plugin: 'java'
* apply plugin: 'eclipse'
*
* configurations {
* provided
* someBoringConfig
* }
*
* eclipse {
* //if you want parts of paths in resulting file to be replaced by variables (files):
* pathVariables 'GRADLE_HOME': file('/best/software/gradle'), 'TOMCAT_HOME': file('../tomcat')
*
* classpath {
* //you can tweak the classpath of the Eclipse project by adding extra configurations:
* plusConfigurations += [ configurations.provided ]
*
* //you can also remove configurations from the classpath:
* minusConfigurations += [ configurations.someBoringConfig ]
*
* //if you want to append extra containers:
* containers 'someFriendlyContainer', 'andYetAnotherContainer'
*
* //customizing the classes output directory:
* defaultOutputDir = file('build-eclipse')
*
* //default settings for downloading sources and Javadoc:
* downloadSources = true
* downloadJavadoc = false
* }
* }
*
*
* For tackling edge cases, users can perform advanced configuration on the resulting XML file.
* It is also possible to affect the way that the Eclipse plugin merges the existing configuration
* via beforeMerged and whenMerged closures.
*
* The beforeMerged and whenMerged closures receive a {@link Classpath} object.
*
* Examples of advanced configuration:
*
*
* apply plugin: 'java'
* apply plugin: 'eclipse'
*
* eclipse {
* classpath {
* file {
* //if you want to mess with the resulting XML in whatever way you fancy
* withXml {
* def node = it.asNode()
* node.appendNode('xml', 'is what I love')
* }
*
* //closure executed after .classpath content is loaded from existing file
* //but before gradle build information is merged
* beforeMerged { classpath ->
* //you can tinker with the {@link Classpath} here
* }
*
* //closure executed after .classpath content is loaded from existing file
* //and after gradle build information is merged
* whenMerged { classpath ->
* //you can tinker with the {@link Classpath} here
* }
* }
* }
* }
*
*/
public class EclipseClasspath {
private Iterable sourceSets;
private Collection plusConfigurations = new ArrayList();
private Collection minusConfigurations = new ArrayList();
private Set containers = new LinkedHashSet();
private File defaultOutputDir;
private boolean downloadSources = true;
private boolean downloadJavadoc;
private XmlFileContentMerger file;
private Map pathVariables = new HashMap();
private boolean projectDependenciesOnly;
private List classFolders;
private final org.gradle.api.Project project;
public EclipseClasspath(org.gradle.api.Project project) {
this.project = project;
}
/**
* The source sets to be added.
*
* See {@link EclipseClasspath} for an example.
*/
public Iterable getSourceSets() {
return sourceSets;
}
public void setSourceSets(Iterable sourceSets) {
this.sourceSets = sourceSets;
}
/**
* The configurations whose files are to be added as classpath entries.
*
* See {@link EclipseClasspath} for an example.
*/
public Collection getPlusConfigurations() {
return plusConfigurations;
}
public void setPlusConfigurations(Collection plusConfigurations) {
this.plusConfigurations = plusConfigurations;
}
/**
* The configurations whose files are to be excluded from the classpath entries.
*
* See {@link EclipseClasspath} for an example.
*/
public Collection getMinusConfigurations() {
return minusConfigurations;
}
public void setMinusConfigurations(Collection minusConfigurations) {
this.minusConfigurations = minusConfigurations;
}
/**
* The classpath containers to be added.
*
* See {@link EclipseClasspath} for an example.
*/
public Set getContainers() {
return containers;
}
public void setContainers(Set containers) {
this.containers = containers;
}
/**
* The default output directory where Eclipse puts compiled classes.
*
* See {@link EclipseClasspath} for an example.
*/
public File getDefaultOutputDir() {
return defaultOutputDir;
}
public void setDefaultOutputDir(File defaultOutputDir) {
this.defaultOutputDir = defaultOutputDir;
}
/**
* Whether to download and associate source Jars with the dependency Jars. Defaults to true.
*
* See {@link EclipseClasspath} for an example.
*/
public boolean isDownloadSources() {
return downloadSources;
}
public void setDownloadSources(boolean downloadSources) {
this.downloadSources = downloadSources;
}
/**
* Whether to download and associate Javadoc Jars with the dependency Jars. Defaults to false.
*
* See {@link EclipseClasspath} for an example.
*/
public boolean isDownloadJavadoc() {
return downloadJavadoc;
}
public void setDownloadJavadoc(boolean downloadJavadoc) {
this.downloadJavadoc = downloadJavadoc;
}
/**
* See {@link #file(Action)}.
*/
public XmlFileContentMerger getFile() {
return file;
}
public void setFile(XmlFileContentMerger file) {
this.file = file;
}
public Map getPathVariables() {
return pathVariables;
}
public void setPathVariables(Map pathVariables) {
this.pathVariables = pathVariables;
}
public boolean isProjectDependenciesOnly() {
return projectDependenciesOnly;
}
public void setProjectDependenciesOnly(boolean projectDependenciesOnly) {
this.projectDependenciesOnly = projectDependenciesOnly;
}
public List getClassFolders() {
return classFolders;
}
public void setClassFolders(List classFolders) {
this.classFolders = classFolders;
}
public org.gradle.api.Project getProject() {
return project;
}
/**
* Further classpath containers to be added.
*
* See {@link EclipseClasspath} for an example.
*
* @param containers the classpath containers to be added
*/
public void containers(String... containers) {
Preconditions.checkNotNull(containers);
this.containers.addAll(Arrays.asList(containers));
}
/**
* Enables advanced configuration like tinkering with the output XML or affecting the way
* that the contents of an existing .classpath file is merged with Gradle build information.
* The object passed to the whenMerged{} and beforeMerged{} closures is of type {@link Classpath}.
*
* See {@link EclipseProject} for an example.
*/
public void file(Closure closure) {
ConfigureUtil.configure(closure, file);
}
/**
* Enables advanced configuration like tinkering with the output XML or affecting the way
* that the contents of an existing .classpath file is merged with Gradle build information.
* The object passed to the whenMerged{} and beforeMerged{} closures is of type {@link Classpath}.
*
* See {@link EclipseProject} for an example.
*
* @since 3.5
*/
public void file(Action super XmlFileContentMerger> action) {
action.execute(file);
}
/**
* Calculates, resolves and returns dependency entries of this classpath.
*/
public List resolveDependencies() {
ClasspathFactory classpathFactory = new ClasspathFactory(this);
List entries = classpathFactory.createEntries();
new UnresolvedDependenciesLogger().log(classpathFactory.getUnresolvedDependencies());
return entries;
}
public void mergeXmlClasspath(Classpath xmlClasspath) {
file.getBeforeMerged().execute(xmlClasspath);
List entries = resolveDependencies();
xmlClasspath.configure(entries);
file.getWhenMerged().execute(xmlClasspath);
}
public FileReferenceFactory getFileReferenceFactory() {
FileReferenceFactory referenceFactory = new FileReferenceFactory();
for (Map.Entry entry : pathVariables.entrySet()) {
referenceFactory.addPathVariable(entry.getKey(), entry.getValue());
}
return referenceFactory;
}
}