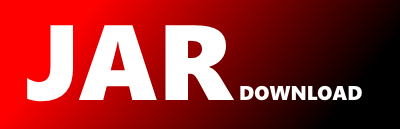
org.gradle.testkit.runner.fixtures.PluginUnderTest.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.testkit.runner.fixtures
import org.gradle.integtests.fixtures.executer.ForkingGradleExecuter
import org.gradle.integtests.fixtures.executer.UnderDevelopmentGradleDistribution
import org.gradle.internal.classpath.DefaultClassPath
import org.gradle.test.fixtures.file.TestDirectoryProvider
import org.gradle.test.fixtures.file.TestFile
import org.gradle.testkit.runner.internal.PluginUnderTestMetadataReading
class PluginUnderTest {
public static final String PLUGIN_ID = "com.company.helloworld"
private final TestDirectoryProvider testDirectoryProvider = new TestDirectoryProvider() {
@Override
TestFile getTestDirectory() {
return projectDir
}
@Override
void suppressCleanup() {
}
}
private final int num
private final TestFile projectDir
private List implClasspath = []
PluginUnderTest(TestFile projectDir) {
this(0, projectDir)
}
PluginUnderTest(int num, TestFile projectDir) {
this.num = num
this.projectDir = projectDir
this.implClasspath.addAll(getImplClasspath())
}
TestFile file(String path) {
projectDir.file(path)
}
PluginUnderTest implClasspath(File... files) {
implClasspath.clear()
implClasspath.addAll(files)
this
}
PluginUnderTest noImplClasspath() {
implClasspath = null
this
}
List getImplClasspath() {
[projectDir.file("build/classes/main"), projectDir.file('build/resources/main')]
}
PluginUnderTest build() {
writeSourceFiles()
writeBuildScript()
new ForkingGradleExecuter(new UnderDevelopmentGradleDistribution(), testDirectoryProvider)
.usingProjectDirectory(projectDir)
.withArguments('classes', '--no-daemon')
.run()
this
}
public T exposeMetadata(Closure closure) {
def originalClassLoader = Thread.currentThread().contextClassLoader
Thread.currentThread().contextClassLoader = new URLClassLoader(new DefaultClassPath(generateMetadataFile().parentFile).asURLArray, originalClassLoader)
try {
closure.call()
} finally {
Thread.currentThread().contextClassLoader = originalClassLoader
}
}
PluginUnderTest writeSourceFiles() {
pluginClassSourceFile() << """
package org.gradle.test
import org.gradle.api.Plugin
import org.gradle.api.Project
class HelloWorldPlugin$suffix implements Plugin {
void apply(Project project) {
project.task('helloWorld$suffix', type: HelloWorld$suffix)
}
}
"""
projectDir.file("src/main/groovy/org/gradle/test/HelloWorld${suffix}.groovy") << """
package org.gradle.test
import org.gradle.api.DefaultTask
import org.gradle.api.tasks.TaskAction
class HelloWorld$suffix extends DefaultTask {
@TaskAction
void doSomething() {
println 'Hello world!$suffix'
project.file("out.txt").text = "Hello world!$suffix"
}
}
"""
projectDir.file("src/main/resources/META-INF/gradle-plugins/${id}.properties") << """
implementation-class=org.gradle.test.HelloWorldPlugin$suffix
"""
this
}
TestFile pluginClassSourceFile() {
projectDir.file("src/main/groovy/org/gradle/test/HelloWorldPlugin${suffix}.groovy")
}
PluginUnderTest writeBuildScript() {
projectDir.file("build.gradle") << """
apply plugin: 'groovy'
dependencies {
compile gradleApi()
compile localGroovy()
}
"""
this
}
private File generateMetadataFile() {
def file = metadataFile.touch()
def properties = new Properties()
if (implClasspath != null) {
def content = implClasspath.collect { it.absolutePath.replaceAll('\\\\', '/') }.join(File.pathSeparator)
properties.setProperty(PluginUnderTestMetadataReading.IMPLEMENTATION_CLASSPATH_PROP_KEY, content)
}
file.withOutputStream { properties.store(it, null) }
file
}
TestFile getMetadataFile() {
projectDir.file("build/pluginUnderTestMetadata/${PluginUnderTestMetadataReading.PLUGIN_METADATA_FILE_NAME}")
}
String getSuffix() {
num > 0 ? "$num" : ""
}
String getUseDeclaration() {
"""
plugins {
id "$id"
}
"""
}
String getId() {
"$PLUGIN_ID$suffix"
}
String getTaskClassName() {
"org.gradle.test.HelloWorld${suffix}"
}
String echoClassNameTask() {
"""
task echo$suffix {
doLast {
println "class name: " + ${taskClassName}.name
}
}
"""
}
String echoClassNameTaskRuntime() {
"""
def loader = getClass().classLoader
task echo$suffix {
doLast {
try {
println "class name: " + loader.loadClass("$taskClassName").name
} catch (ClassNotFoundException e) {
throw new RuntimeException("failed to load class $taskClassName")
}
}
}
"""
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy