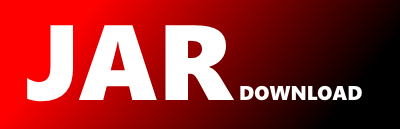
org.gradle.tooling.internal.consumer.DefaultBuildActionExecuter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.tooling.internal.consumer;
import org.gradle.api.Transformer;
import org.gradle.tooling.BuildAction;
import org.gradle.tooling.BuildActionExecuter;
import org.gradle.tooling.GradleConnectionException;
import org.gradle.tooling.ResultHandler;
import org.gradle.tooling.internal.consumer.async.AsyncConsumerActionExecutor;
import org.gradle.tooling.internal.consumer.connection.ConsumerAction;
import org.gradle.tooling.internal.consumer.connection.ConsumerConnection;
import org.gradle.tooling.internal.consumer.parameters.ConsumerOperationParameters;
class DefaultBuildActionExecuter extends AbstractLongRunningOperation> implements BuildActionExecuter {
private final BuildAction buildAction;
private final AsyncConsumerActionExecutor connection;
public DefaultBuildActionExecuter(BuildAction buildAction, AsyncConsumerActionExecutor connection, ConnectionParameters parameters) {
super(parameters);
operationParamsBuilder.setEntryPoint("BuildActionExecuter API");
this.buildAction = buildAction;
this.connection = connection;
}
@Override
protected DefaultBuildActionExecuter getThis() {
return this;
}
@Override
public BuildActionExecuter forTasks(String... tasks) {
operationParamsBuilder.setTasks(rationalizeInput(tasks));
return getThis();
}
@Override
public BuildActionExecuter forTasks(Iterable tasks) {
operationParamsBuilder.setTasks(rationalizeInput(tasks));
return getThis();
}
public T run() throws GradleConnectionException {
BlockingResultHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy