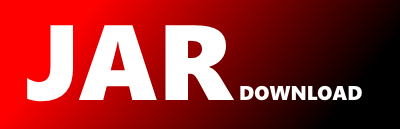
org.gradle.tooling.BuildController Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.tooling;
import org.gradle.api.Action;
import org.gradle.tooling.model.Model;
import org.gradle.tooling.model.gradle.GradleBuild;
import javax.annotation.Nullable;
/**
* Provides a {@link BuildAction} various ways to control a Gradle build and access information about the build.
*
* @since 1.8
*/
public interface BuildController {
/**
* Fetches a snapshot of the model of the given type for the default project. The default project is generally the
* project referenced when a {@link ProjectConnection} is created.
*
* Any of following models types may be available, depending on the version of Gradle being used by the target
* build:
*
*
* - {@link GradleBuild}
* - {@link org.gradle.tooling.model.build.BuildEnvironment}
* - {@link org.gradle.tooling.model.GradleProject}
* - {@link org.gradle.tooling.model.gradle.BuildInvocations}
* - {@link org.gradle.tooling.model.gradle.ProjectPublications}
* - {@link org.gradle.tooling.model.idea.IdeaProject}
* - {@link org.gradle.tooling.model.idea.BasicIdeaProject}
* - {@link org.gradle.tooling.model.eclipse.EclipseProject}
* - {@link org.gradle.tooling.model.eclipse.HierarchicalEclipseProject}
*
*
* A build may also expose additional custom tooling models. You can use this method to query these models.
*
* @param modelType The model type.
* @param The model type.
* @return The model.
* @throws UnknownModelException When the default project does not support the requested model.
*
* @since 1.8
*/
T getModel(Class modelType) throws UnknownModelException;
/**
* Fetches a snapshot of the model of the given type, if available.
*
* See {@link #getModel(Class)} for more details.
*
* @param modelType The model type.
* @param The model type.
* @return The model, or null if not present.
*/
@Nullable
T findModel(Class modelType);
/**
* Returns an overview of the Gradle build, including some basic details of the projects that make up the build.
* This is equivalent to calling {@code #getModel(GradleBuild.class)}.
*
* @return The model.
*/
GradleBuild getBuildModel();
/**
* Fetches a snapshot of the model of the given type for the given element, usually a Gradle project.
*
* The following elements are supported:
*
*
* - Any {@link org.gradle.tooling.model.gradle.BasicGradleProject}
* - Any {@link org.gradle.tooling.model.GradleProject}
* - Any {@link org.gradle.tooling.model.eclipse.EclipseProject}
* - Any {@link org.gradle.tooling.model.idea.IdeaModule}
*
*
* See {@link #getModel(Class)} for more details.
*
* @param target The target element, usually a project.
* @param modelType The model type.
* @param The model type.
* @return The model.
* @throws UnknownModelException When the target project does not support the requested model.
*/
T getModel(Model target, Class modelType) throws UnknownModelException;
/**
* Fetches a snapshot of the model of the given type, if available.
*
* See {@link #getModel(Model, Class)} for more details.
*
* @param modelType The model type.
* @param The model type.
* @return The model, or null if not present.
*/
@Nullable
T findModel(Model target, Class modelType);
/**
* Fetches a snapshot of the model of the given type using the given parameter.
*
* See {@link #getModel(Model, Class, Class, Action)} for more details.
*
* @param modelType The model type.
* @param The model type.
* @param parameterType The parameter type.
* @param The parameter type.
* @param parameterInitializer Action to configure the parameter
* @return The model.
* @throws UnknownModelException When the target project does not support the requested model.
* @throws UnsupportedVersionException When the target project does not support the requested model or Gradle version does not support parametrized models.
*
* @since 4.4
*/
T getModel(Class modelType, Class parameterType, Action super P> parameterInitializer) throws UnsupportedVersionException, UnknownModelException;
/**
* Fetches a snapshot of the model of the given type using the given parameter, if available.
*
*
See {@link #getModel(Model, Class, Class, Action)} for more details.
*
* @param modelType The model type.
* @param The model type.
* @param parameterType The parameter type.
* @param The parameter type.
* @param parameterInitializer Action to configure the parameter
* @return The model.
*
* @since 4.4
*/
@Nullable
T findModel(Class modelType, Class parameterType, Action super P> parameterInitializer);
/**
* Fetches a snapshot of the model of the given type for the given element using the given parameter.
*
*
The parameter type must be an interface only with getters and setters and no nesting is supported.
* The Tooling API will create a proxy instance of this interface and use the initializer to run against
* that instance to configure it and then pass to the model builder.
*
*
* See {@link #getModel(Class)} for more details.
*
* @param target The target element, usually a project.
* @param modelType The model type.
* @param The model type.
* @param parameterType The parameter type.
* @param The parameter type.
* @param parameterInitializer Action to configure the parameter
* @return The model.
* @throws UnknownModelException When the target project does not support the requested model.
* @throws UnsupportedVersionException When the target project does not support the requested model or Gradle version does not support parametrized models.
*
* @since 4.4
*/
T getModel(Model target, Class modelType, Class parameterType, Action super P> parameterInitializer) throws UnsupportedVersionException, UnknownModelException;
/**
* Fetches a snapshot of the model of the given type for the given element using the given parameter, if available.
*
*
See {@link #getModel(Model, Class, Class, Action)} for more details.
*
* @param target The target element, usually a project.
* @param modelType The model type.
* @param The model type.
* @param parameterType The parameter type.
* @param The parameter type.
* @param parameterInitializer Action to configure the parameter
* @return The model.
*
* @since 4.4
*/
@Nullable
T findModel(Model target, Class modelType, Class parameterType, Action super P> parameterInitializer);
}